Inside this article we will see the concept i.e How To Convert Associative Arrays into XML in PHP. Article contains the classified information about How To Convert multidimensional array to XML file.
Whenever you work with XML data and also with multidimensional array then may be you need help to convert array of data into XML format. So, if you are looking for this here, then article is totally for you to learn and see how to do it.
Also, If you want to store dynamic data into an XML file then we can easily store data in an XML file using PHP.
Read More: Convert Unix Timestamp To Readable Date Time in PHP
Let’s get started.
Prepare Data in Associative Array
Here, you can see we have a associative array of employees data.
// Associative array $empData = array( 'title' => 'Employee Details', 'employee' => array( array('firstname' => 'Sanjay', 'lastname' => 'Kumar', 'username' => 'sk987'), array('firstname' => 'Ashish', 'lastname' => 'Kumar', 'username' => 'ashish.985'), array('firstname' => 'Vijay', 'lastname' => 'Rohila', 'username' => 'vijayk.ro'), array('firstname' => 'Dhananjay', 'lastname' => 'Negi', 'username' => 'dj.negi'), array('firstname' => 'Siddharth', 'lastname' => 'Singh', 'username' => 'sid.992') ) );
This array of data will pass into a helper function which converts it into XML format.
Create a Helper Function (Array To XML)
By using the concept of PHP class and XML functions of it, we create a function which converts array of data into XML format and also saves it into specified folder.
// Function to convert Array To XML function createXMLFile($empData) { $title = $empData['title']; $totalEmployee = count($empData['employee']); $xmlDocument = new DOMDocument(); $root = $xmlDocument->appendChild($xmlDocument->createElement("employee_details")); $root->appendChild($xmlDocument->createElement("title", $title)); $root->appendChild($xmlDocument->createElement("totalEmployee", $totalEmployee)); $empRecords = $root->appendChild($xmlDocument->createElement('employee')); foreach ($empData['employee'] as $employee) { if (!empty($employee)) { $empRecord = $empRecords->appendChild($xmlDocument->createElement('employee')); foreach ($employee as $key => $val) { $empRecord->appendChild($xmlDocument->createElement($key, $val)); } } } $fileName = str_replace(' ', '_', $title) . '_' . time() . '.xml'; $xmlDocument->formatOutput = true; $xmlDocument->save("xml/" . $fileName); }
Application Programming
Create a folder say array-2-xml in your localhost directory. Create a file index.php into it.
Read More: How To Check If a Database Table Exists With Laravel
Next, create a sub folder with name xml into project folder. In this folder we will save generated XML file.
<?php // Associative array $empData = array( 'title' => 'Employee Details', 'employee' => array( array('firstname' => 'Sanjay', 'lastname' => 'Kumar', 'username' => 'sk987'), array('firstname' => 'Ashish', 'lastname' => 'Kumar', 'username' => 'ashish.985'), array('firstname' => 'Vijay', 'lastname' => 'Rohila', 'username' => 'vijayk.ro'), array('firstname' => 'Dhananjay', 'lastname' => 'Negi', 'username' => 'dj.negi'), array('firstname' => 'Siddharth', 'lastname' => 'Singh', 'username' => 'sid.992') ) ); // Function to convert Array To XML function createXMLFile($empData) { $title = $empData['title']; $totalEmployee = count($empData['employee']); $xmlDocument = new DOMDocument(); $root = $xmlDocument->appendChild($xmlDocument->createElement("employee_details")); $root->appendChild($xmlDocument->createElement("title", $title)); $root->appendChild($xmlDocument->createElement("totalEmployee", $totalEmployee)); $empRecords = $root->appendChild($xmlDocument->createElement('employee')); foreach ($empData['employee'] as $employee) { if (!empty($employee)) { $empRecord = $empRecords->appendChild($xmlDocument->createElement('employee')); foreach ($employee as $key => $val) { $empRecord->appendChild($xmlDocument->createElement($key, $val)); } } } $fileName = str_replace(' ', '_', $title) . '_' . time() . '.xml'; $xmlDocument->formatOutput = true; $xmlDocument->save("xml/" . $fileName); } echo createXMLFile($empData); // Function call
Output
Open browser and type this URL to run application.
URL: http://localhost/array-2-xml/index.php
Once, application executes. You will see a xml file with name Employee_Details_1671070573.xml into /xml folder.
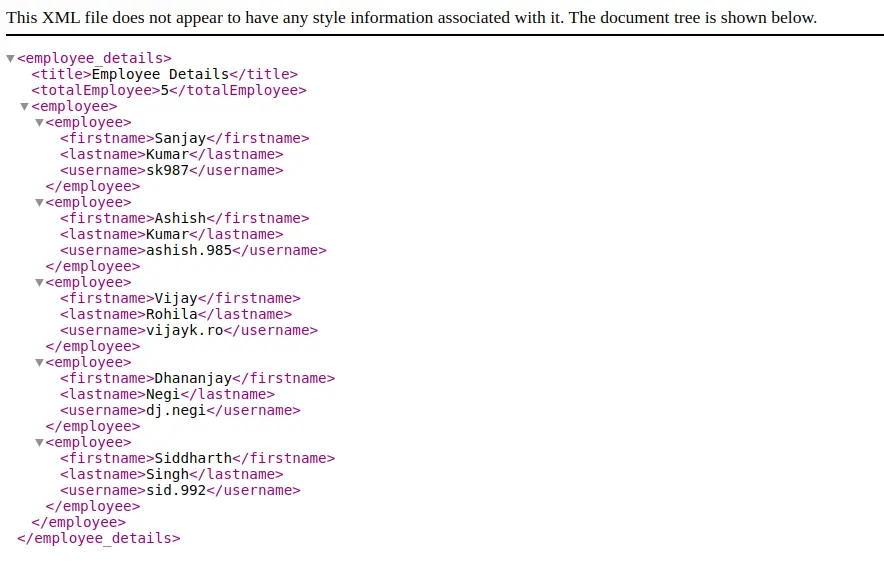
We hope this article helped you to learn How To Convert Associative Arrays into XML in PHP in a very detailed way.
Read More: How To Add JQuery Ajax Loading Spinner in Laravel Example
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.