Inside this article we will see the concept i.e How To Only Allow Numbers in a Text Box Using jQuery Example Tutorial. Article contains the classified information about How to allow only numeric (0-9) in HTML inputbox using jQuery.
If you are looking for a solution i.e How to allow only numbers in Textbox using jQuery then this article will help you a lot for this. Tutorial is super easy to understand and implement it in your code as well.
Examples (Only Numbers):
123456, 8529630, 555555, etc.
Read More: How To Check Laravel Application Environment Tutorial
Let’s get started.
Application Programming
Create a folder say number-validation in your localhost directory. Create a file index.html into it.
<!DOCTYPE html> <html lang="en"> <head> <title>Allow Only Numbers in Textbox jQuery On Keypress</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> </head> <body> <div class="container" style="margin-top: 40px;"> <div class="form-group col-sm-4"> <label for="phone">Phone Number:</label> <input type="text" class="form-control" id="phone" placeholder="Enter Phone Number" name="phone"> <span id="phone_err" style="color: red; display:none;">Only Numbers allowed.</span> </div> </div> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> <script type="text/javascript"> $(function() { $("#phone").keypress(function(e) { var keyCode = e.keyCode || e.which; //Regex for Valid Characters i.e. Numbers. var regex = /^[0-9]+$/; //Validate TextBox value against the Regex. var isValid = regex.test(String.fromCharCode(keyCode)); if (!isValid) { $("#phone_err").show(); }else{ $("#phone_err").hide(); } return isValid; }); }); </script> </body> </html>
Here, above code is only for validating numbers on keypress. This is not specific to enter mobile number or phone number.
Concept
$("#phone").keypress(function(e) {
var keyCode = e.keyCode || e.which;
//Regex for Valid Characters i.e. Numbers.
var regex = /^[0-9]+$/;
//Validate TextBox value against the Regex.
var isValid = regex.test(String.fromCharCode(keyCode));
if (!isValid) {
$("#phone_err").show();
}else{
$("#phone_err").hide();
}
return isValid;
});
Application Testing
Open browser and type your application URL:
Read More: How To Check Laravel Application Environment Tutorial
URL: http://localhost/number-validation/index.html
Output,
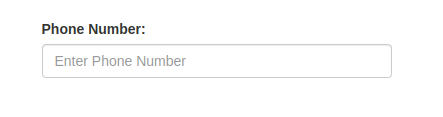
When you enter value other than numbers,
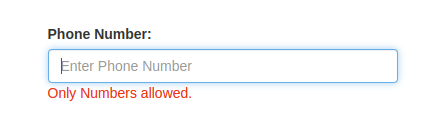
Only numbers input on input box,
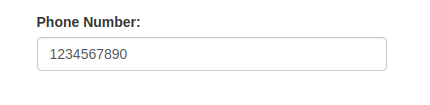
We hope this article helped you to learn How To Only Allow Numbers in a Text Box Using jQuery Example Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.