Inside this article we will see the concept i.e Laravel 9 Ajax PUT request example tutorial. Article contains the classified information about working with Ajax PUT request in laravel 9.
While working with forms we have these request methods – POST, GET, PUT etc. Article contains the information about PUT request in Ajax request. We will consider a list of users. To update a specific user we will fire an ajax request using PUT request type.
Learn More –
- Laravel 9 How To Return JSON Response Example Tutorial
- Laravel 9 Ajax DELETE Request Example Tutorial
- Laravel 9 Custom Blade Component Example Tutorial
- Laravel 9 How To Add Enumeration Column in Migration Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Generate Fake Data Using Factory
As you install laravel 9 setup, you should see these files –
- User.php a model file inside /app/Models folder.
- UserFactory.php a factory file inside /database/factories folder.
We will use concept of factory to seed dummy data to this application. But first you need to migrate your application and create users table into your database.
$ php artisan tinker
It will open tinker shell to execute commands. Copy and paste this command and execute to create test data.
>>> User::factory()->count(20)->create()
It will generate 20 fake rows of users into table.
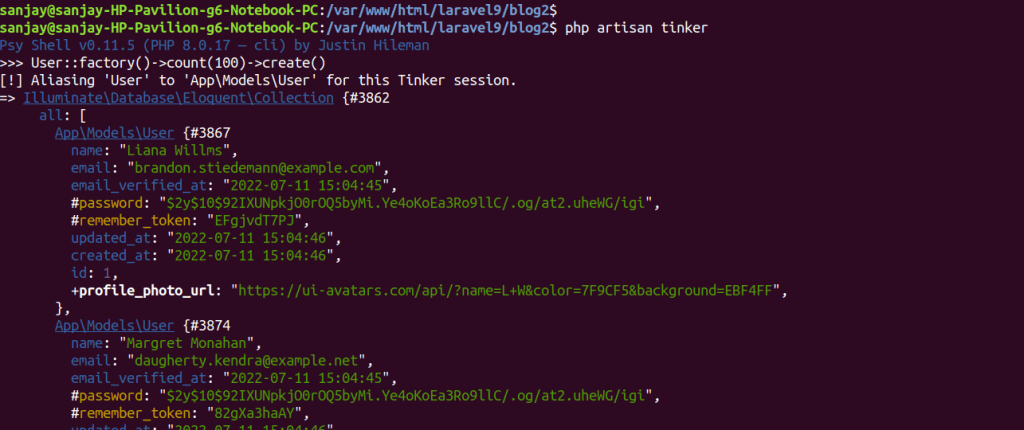
Create Controller
Next,
We need to create a controller file.
$ php artisan make:controller UserController
It will create a file UserController.php inside /app/Http/Controllers folder.
Open UserController.php and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\User; class UserController extends Controller { /** * Write code on Method * * @return response() */ public function index() { $users = User::paginate(10); return view('users', compact('users')); } /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function update(Request $request, $id) { User::find($id)->update([ 'name' => $request->name, 'email' => $request->email ]); return response()->json(['success' => 'User Updated Successfully!']); } }
Create Blade Layout File
Go to /resources/views folder and create a file with name users.blade.php
Open users.blade.php and write this complete code into it.
<!DOCTYPE html> <html> <head> <title>Laravel 9 Ajax PUT Request Example Tutorial</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.0.1/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js"></script> <meta name="csrf-token" content="{{ csrf_token() }}"> </head> <body> <div class="container" style="margin-top: 10px;"> <h3 style="text-align: center;">Laravel 9 Ajax PUT Request Example Tutorial</h3> <table class="table table-bordered data-table" style="margin-top: 30px;"> <thead> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Action</th> </tr> </thead> <tbody> @foreach ($users as $user) <tr data-id="{{ $user->id }}"> <td>{{ $user->id }}</td> <td>{{ $user->name }}</td> <td>{{ $user->email }}</td> <td> <a href="javascript:void(0)" class="btn btn-primary edit-user">Edit</a> </td> </tr> @endforeach </tbody> </table> </div> <!-- Modal --> <div class="modal fade" id="userEditModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title" id="exampleModalLabel">Edit User</h5> <button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button> </div> <div class="modal-body"> <input type="hidden" id="user-id" name="id"></span> <p><strong>Name:</strong> <br /> <input type="text" name="name" id="user-name" class="form-control"></span></p> <p><strong>Email:</strong> <br /> <input type="email" name="email" id="user-email" class="form-control"></span></p> </div> <div class="modal-footer"> <button type="button" class="btn btn-success" id="user-update">Update</button> <button type="button" class="btn btn-secondary" data-bs-dismiss="modal">Close</button> </div> </div> </div> </div> </body> <script type="text/javascript"> $(document).ready(function() { $.ajaxSetup({ headers: { 'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content') } }); /*------------------------------------------ -------------------------------------------- When click user on Edit Button -------------------------------------------- --------------------------------------------*/ $(document).on('click', '.edit-user', function() { var userURL = $(this).data('url'); $('#userEditModal').modal('show'); $('#user-id').val($(this).parents("tr").find("td:nth-child(1)").text()); $('#user-name').val($(this).parents("tr").find("td:nth-child(2)").text()); $('#user-email').val($(this).parents("tr").find("td:nth-child(3)").text()); }); /*------------------------------------------ -------------------------------------------- When click user on Show Button -------------------------------------------- --------------------------------------------*/ $('body').on('click', '#user-update', function() { var id = $('#user-id').val(); var name = $('#user-name').val(); var email = $('#user-email').val(); $.ajax({ url: '/users/' + id, type: 'PUT', dataType: 'json', data: { name: name, email: email }, success: function(data) { $('#userEditModal').modal('hide'); alert(data.success); $("tr[data-id=" + id + "]").find("td:nth-child(2)").text(name); $("tr[data-id=" + id + "]").find("td:nth-child(3)").text(email); } }); }); }); </script> </html>
Concept
To update a single user row, fired an ajax request using PUT request type.
$('body').on('click', '#user-update', function() {
var id = $('#user-id').val();
var name = $('#user-name').val();
var email = $('#user-email').val();
$.ajax({
url: '/users/' + id,
type: 'PUT',
dataType: 'json',
data: {
name: name,
email: email
},
success: function(data) {
$('#userEditModal').modal('hide');
alert(data.success);
$("tr[data-id=" + id + "]").find("td:nth-child(2)").text(name);
$("tr[data-id=" + id + "]").find("td:nth-child(3)").text(email);
}
});
});
Add Route
Open web.php from /routes folder and add these route into it.
//... use App\Http\Controllers\UserController; //... Route::get('users', [UserController::class, 'index']); Route::put('users/{id}', [UserController::class, 'update'])->name('users.update');
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/users

When you click on any row – Edit button. It will open modal with existing user value. If you put new updated values and click on Update button, it will save updated values into database.
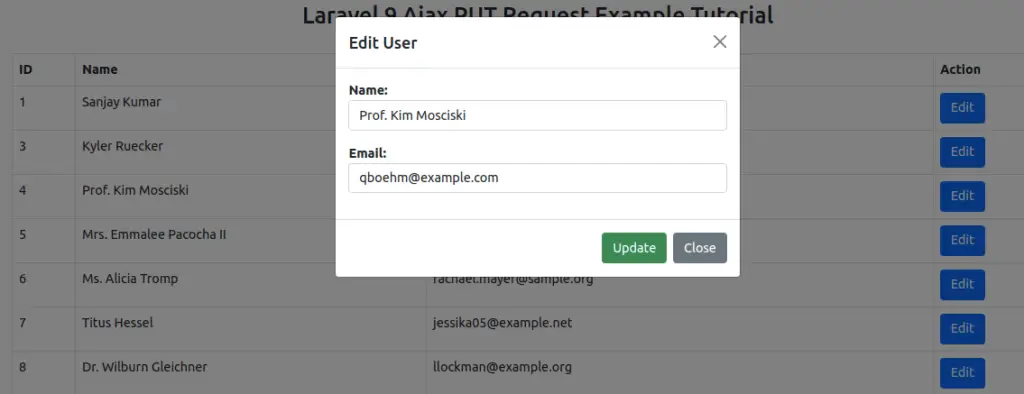
We hope this article helped you to Laravel 9 Ajax PUT Request Example Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.