Components are the re-usable section or layout which we can use inside laravel application. In laravel there are two methods to writing a components: class based components and anonymous components.
Inside this article we will see laravel 9 how to create reusable components using components and slots. This article will be super easy to understand for the implementation.
Laravel provide components and slots for creating blade component sections in application.
Learn More –
- How To Define Laravel 9 Global or Constant Variables
- How to Generate Unique Slug in Laravel 9 Tutorial
- How To Get File Size From URL in Laravel 9 Tutorial
- How To Get HTTP Hostname In Laravel 9 Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create a Reusable Component File
Create components folder inside /resources/views.
Create a file with name message.blade.php inside /components folder.
Open message.blade.php and write this code into it.
<div class="panel {{ $class }}"> <div class="panel-heading">{{ $title }}</div> <div class="panel-body">{{ $slot }}</div> </div>
Here, we have 3 placeholders as $class, $title and $slot.
When we will use this component then according to usage type we will replace these placeholder values.
Create a Layout File
Create a my-template.blade.php blade layout file inside /resources/views folder.
Open my-template.blade.php and write this code into it.
<!DOCTYPE html> <html> <head> <title>How to Create Reusable Components in Laravel 9</title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css" /> </head> <body> <div class="container"> <h3>How to Create Reusable Components in Laravel 9</h3> <div style="margin-top:20px;"> <!-- For General info --> @component('components.message') @slot('class') panel-primary @endslot @slot('title') This is from Online Web Tutor @endslot Custom components with primary class @endcomponent <br/> <!-- For Error --> @component('components.message') @slot('class') panel-danger @endslot @slot('title') This is from Online Web Tutor @endslot Custom components with danger class @endcomponent <br/> <!-- For Success --> @component('components.message') @slot('class') panel-success @endslot @slot('title') This is from Online Web Tutor @endslot Custom components with success class @endcomponent </div> </div> </body> </html>
Concept
<!-- For Success -->
@component('components.message') ----> message component
@slot('class')
panel-success ----> component class
@endslot
@slot('title')
This is from Online Web Tutor ----> component title
@endslot
Custom components with success class ----> slot
@endcomponent
Code Explanation
- @component(‘components.message’) … @endcomponent is used to use the component. It uses message.blade.php from components folder.
- @slot(‘class’) … @endslot It used to place dynamic value for class ($class) placeholder. Same will be for $title placeholder.
- Custom message – Custom components with success class will be rendered using $slot placeholder.
Add Route
Open web.php file from /routes folder. Add this route into it.
//... Route::get('my-message', function () { return view('my-template'); }); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL – http://127.0.0.1:8000/my-message
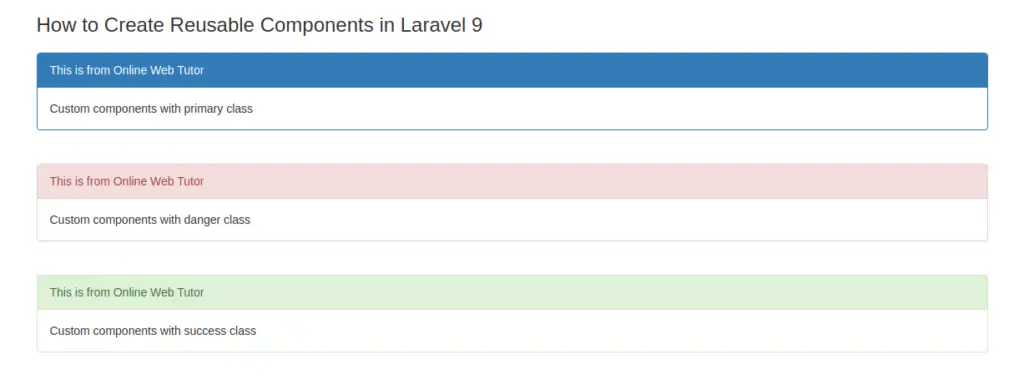
We hope this article helped you to learn about laravel 9 How to create reusable components in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.