Inside this article we will see the concept i.e PHP Exception: How To Create Your Own Exception Class. Article contains the classified information i.e PHP Exception Handling.
An error or unexpected behaviour that happens while a PHP script is being executed is referred to as an exception. Developers can manage problems in a more organised and predictable manner by passing control to an exception handler when an exception is thrown, interrupting the script’s normal flow.
Read More: What is CSRF Token and How To Use in Core PHP Tutorial
Let’s get started.
What is an Exception in PHP?
In PHP, an exception is an error or unexpected behavior that occurs during the execution of a script. When an exception is thrown, it interrupts the normal flow of the script and passes control to an exception handler. An exception can be thrown explicitly using the throw
keyword or implicitly by PHP itself when it encounters a fatal error or unhandled exception.
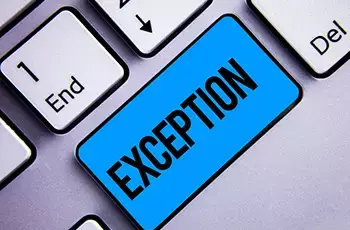
Exceptions in PHP are instances of classes that implement the built-in Throwable
interface. An exception object contains information about the error, such as the error message, file name, line number, and stack trace.
Using exceptions, developers can handle errors in a more structured and predictable way, rather than relying on error codes or other ad-hoc mechanisms. By catching and handling exceptions, developers can gracefully recover from errors, log error messages, or display custom error messages to users.
Built-in Exception Classes of PHP
PHP provides several built-in exception classes that developers can use to handle different types of errors. Here is a list of some of the most commonly used exception classes in PHP:
- Exception: The base class for all exceptions in PHP.
- ErrorException: An exception that represents a PHP error.
- InvalidArgumentException: An exception that is thrown when an argument is not of the expected type.
- RuntimeException: An exception that represents a runtime error that cannot be handled at compile time.
- OutOfBoundsException: An exception that is thrown when an index is out of range.
- PDOException: An exception that represents an error in a PDO operation.
- DivisionByZeroError: An exception that is thrown when a division by zero occurs.
- TypeError: An exception that is thrown when a function argument or return value is not of the expected type.
There are many other built-in exception classes in PHP, and developers can also create their own custom exception classes.
Read More: How Many Different ways of Writing Functions in JavaScript
How To Create Custom Exception in PHP?
Developers can create their own custom exception classes in PHP by extending the built-in Exception
class or any of its subclasses.
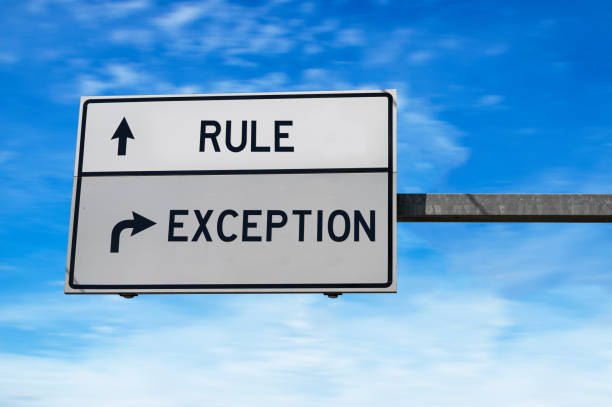
Here is an example of how to create a custom exception class in PHP:
<?php class MyException extends Exception { public function __construct($message, $code = 0, Exception $previous = null) { parent::__construct($message, $code, $previous); } }
In this example, we define a new class called MyException
that extends the built-in Exception
class. The class has a constructor that takes three parameters: the error message, an error code (optional), and a previous exception (optional).
Read More: How To Prevent Website From Being Loaded in Iframe
To throw an instance of our custom exception class, we can use the throw
keyword, like this:
throw new MyException('An error occurred.');
This will throw an instance of our MyException
class with the specified error message. We can catch and handle the exception using a try-catch block, like this:
try {
// Some code that may throw an exception
} catch (MyException $e) {
// Handle the exception
echo $e->getMessage();
}
In this example, we catch any instance of MyException
that is thrown and handle it by displaying the error message using the getMessage()
method.
We hope this article helped you to learn PHP Exception How To Create Your Own Exception Class Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.