Inside this article we will see i.e PHP how to list all files from AWS s3 bucket. There are very few simple steps by which we can list all uploaded files and folders from AWS s3 bucket.
AWS provides a composer package for PHP application. By the help of this package we can easily communicate with AWS s3 bucket with few keys and their APIs.
This article will give you the classified information about uploaded files, folders and subfolders from S3 bucket in PHP. If you are wondering to find the solution about to get information of uploaded files and folders, then this article will help you a lot with AWS S3 bucket.
Learn More –
- PHP How To Delete Folder From AWS S3 Bucket
- PHP How To Upload File To AWS S3 Bucket Tutorial
- PHP How To Upload Folder and Files To AWS S3 Bucket
Let’s get started.
Install AWS PHP SDK
Before installation installation, please make sure these –
- System should have composer installed
- Project should have composer.json file
How To generate composer.json file
To generate a composer.json file. Open project into terminal and run this command.
$ composer init
You can provide either the information to generate else you can simply press Enter to pick default value.
So, here we have generate composer.json file with all default values in it.
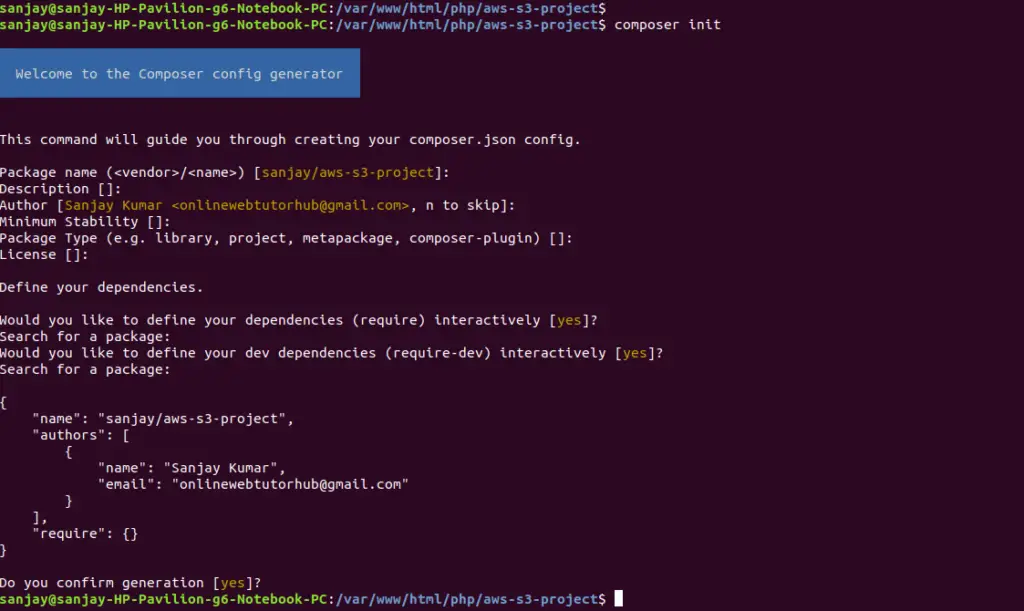
Next,
Install AWS SDK.
$ composer require aws/aws-sdk-php
You will see like this –
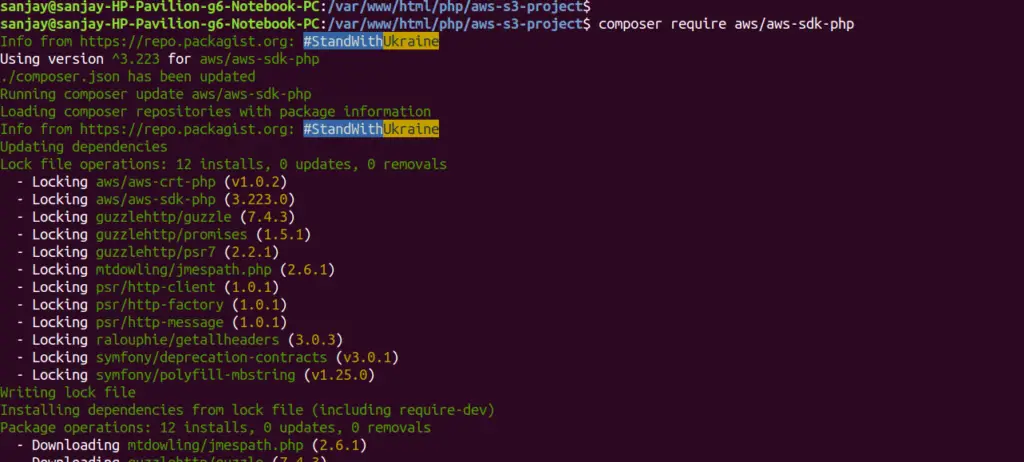
It will create a vendor folder into your project folder.
Next,
Create Application To Get Folder & Files
Create a file with any name like list-objects.php.
You need these while working with AWS S3 bucket to get information of uploaded files or folders.
- AWS Access Key
- AWS Secret Key
- Bucket Name
Step #1 – AWS S3 Connection
// Instantiate an Amazon S3 client.
$s3Client = new S3Client([
'version' => 'latest',
'region' => 'us-east-1', // Your AWS region
'credentials' => [
'key' => $awsAccessKey,
'secret' => $awsSecretKey
]
]);
Step #2 – Get All Objects
$results = $s3Client->getPaginator('ListObjects', [
'Bucket' => $bucket
]);
Step #3 – Get Each Details
foreach ($results as $result) {
foreach ($result['Contents'] as $object) {
echo $object['Key'] . PHP_EOL;
}
}
Open list-objects.php file and write this complete code into it.
<?php require 'vendor/autoload.php'; use Aws\S3\S3Client; $awsAccessKey = 'XXXX'; $awsSecretKey = 'YYYYYYYYYY'; $bucket = 'your-bucket-name'; // Instantiate an Amazon S3 client. $s3Client = new S3Client([ 'version' => 'latest', 'region' => 'us-east-1', // Your AWS region 'credentials' => [ 'key' => $awsAccessKey, 'secret' => $awsSecretKey ] ]); // Use the high-level iterators (returns ALL of your objects). try { $results = $s3Client->getPaginator('ListObjects', [ 'Bucket' => $bucket ]); echo "<pre>"; foreach ($results as $result) { foreach ($result['Contents'] as $object) { echo $object['Key'] . PHP_EOL; } } } catch (S3Exception $e) { echo $e->getMessage() . PHP_EOL; }
The above code will list all files, folders and subfolder what you have in your AWS s3 bucket.
We hope this article helped you to learn about PHP How To List All Files From AWS S3 Bucket Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.