Inside this article we will see the concept i.e PHP preview an image before upload using jquery. Article contains the classified information about render an upload preview of an image before sending to server.
We will use the very basic concept of jquery to read the uploaded and generate a preview of that. This concept is very useful when you are giving an upload option of images to your dashboards. Also we will add PHP validations on server for this image upload.
If you are looking for an article which provides you the information to create an image preview before upload then this article will help you in terms of PHP and jQuery.
Learn More –
- PHP MySQLi Select2 Ajax Autocomplete Search Tutorial
- How To Send PHP cURL POST Request with JSON Parameters
- How To Send PHP cURL POST Request with Parameters
- PHP MySQLi How To Use Select2 for Multiple Select Tutorial
Let’s get started.
Application Folder Structure
You need to create a folder structure to develop this application in PHP and MySQLi. Have a look the files and folder inside this application –
Create a folder with name php-preview-image-before-upload and create these 2 files into it. And also create a uploads/ folder. This is to store images. Make sure this folder should have sufficient permission to read and write files into it.
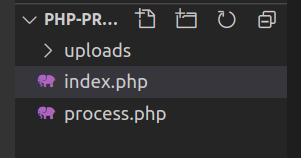
Application Programming
Open index.php file and write this following code into it. Inside this file we will create a layout as well as include jquery code which render preview for an image file when we upload.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>PHP Preview an Image Before Upload Using jQuery</title> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@4.6.1/dist/css/bootstrap.min.css" integrity="sha384-zCbKRCUGaJDkqS1kPbPd7TveP5iyJE0EjAuZQTgFLD2ylzuqKfdKlfG/eSrtxUkn" crossorigin="anonymous"> </head> <body> <div class="container mt-5"> <div class="row"> <div class="col-md-12"> <div class="card w-75 m-auto"> <div class="card-header text-center bg-primary text-white"> <h4>PHP Preview an Image Before Upload Using jQuery</h4> </div> <div class="card-body"> <form action="javascript:void(0);" id="form" method="post" enctype="multipart/form-data"> <div class="form-group"> <label for="myImage"><strong>Select Image : </strong><span class="text-danger">*</span></label> <input type="file" id="myImage" class="form-control"> <p id="errorMs" class="text-danger"></p> </div> <img id="preview-image" width="200px" class="mb-2"> <br> <div class="d-flex"> <input type="submit" class="btn btn-success" id="submit" value="Upload"> </div> </form> </div> </div> </div> </div> </div> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script> $(document).ready(function() { // Preview image $('#myImage').change(function() { let reader = new FileReader(); reader.onload = (e) => { $('#preview-image').attr('src', e.target.result); $("#errorMs").hide(); } reader.readAsDataURL(this.files[0]); }); // Submit data $("#submit").click(function(e) { e.preventDefault(); let form_data = new FormData(); let img = $("#myImage")[0].files; if (img.length > 0) { form_data.append('my_image', img[0]); $.ajax({ url: 'process.php', type: 'post', data: form_data, contentType: false, processData: false, success: function(res) { const data = JSON.parse(res); if (data.error != 1) { $('.form-group').prepend('<p class="alert alert-success">Uploaded Successfully.</p>') } else { $("#errorMs").text(data.em); } $('#preview-image').fadeOut(800); } }); } else { $("#errorMs").text("Please select an image."); } }); }); </script> </body> </html>
Concept
When upload any image file, this code will render preview of it.
// Preview image
$('#myImage').change(function() {
let reader = new FileReader();
reader.onload = (e) => {
$('#preview-image').attr('src', e.target.result);
$("#errorMs").hide();
}
reader.readAsDataURL(this.files[0]);
});
Ajax Handler – Form Submit
Open process.php file from folder and write this code into it. This file will process ajax request and save image file into uploads/ folder.
<?php // Checking for File upload if (isset($_FILES['my_image'])) { // Gettings File Info $img_name = $_FILES['my_image']['name']; $img_size = $_FILES['my_image']['size']; $tmp_name = $_FILES['my_image']['tmp_name']; $error = $_FILES['my_image']['error']; if ($error === 0) { // File size validation if ($img_size > 1000000) { // In KB $em = "Sorry, your file is too large."; $error = array('error' => 1, 'em' => $em); echo json_encode($error); exit(); } else { // File extension validation $img_ex = pathinfo($img_name, PATHINFO_EXTENSION); $img_ex_lc = strtolower($img_ex); $allowed_exs = array("jpg", "jpeg", "png"); if (in_array($img_ex_lc, $allowed_exs)) { // On success: Upload image to uploads/ folder. $new_img_name = uniqid("IMG-", true) . '.' . $img_ex_lc; $img_upload_path = "uploads/" . $new_img_name; move_uploaded_file($tmp_name, $img_upload_path); $res = array('error' => 0, 'src' => $new_img_name); echo json_encode($res); exit(); } else { $em = "You can't upload files of this type"; $error = array('error' => 1, 'em' => $em); echo json_encode($error); exit(); } } } else { $em = "unknown error occurred!"; $error = array('error' => 1, 'em' => $em); echo json_encode($error); exit(); } }
Inside above code we have added file validation in terms of Size, file type.
On successful upload, image will be uploaded into /uploads folder.
Application Testing
Now,
URL: http://localhost/php-preview-image-before-upload/index.php
Upload Image Form
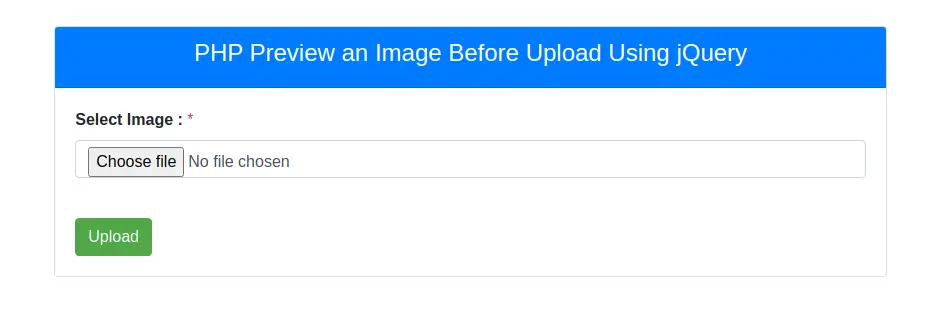
On image upload, we can see the image preview of it.
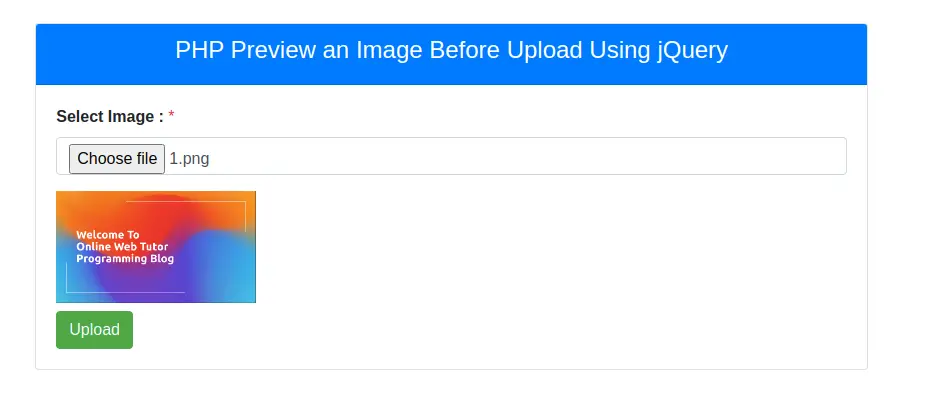
Download Complete Source Code
We hope this article helped you to learn PHP Preview an Image Before Upload Using jQuery Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.