With our detailed lesson on the CodeIgniter 4 Form Validation Library, you can unleash the potential of secure and trustworthy user input. Validating user input is critical in the changing world of web development to ensure data accuracy and the integrity of your apps.
Nowadays, submitting a form with user input data is a common process. But do you think user always proceed with the valid input values? If we think in real time application development then No, end users sure fill and do their experiment with form inputs with all possible ways.
Read More: CodeIgniter 4 How to Work with Redirection Tutorial
We’ll walk you through the process of using the Form Validation Library in CodeIgniter 4 in this tutorial. We’ll lead you through each step of creating a robust and user-friendly validation process, from setting up validation rules to smoothly integrating them into your forms.
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Now, let’s configure database and application connectivity.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Create Database
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
We will use MySQL command to create database. Run this command into Sql tab of PhpMyAdmin.
CREATE DATABASE codeigniter4_app;
Successfully, we have created a database.
Create Database Table
Next, we need a table. That table will be responsible to store data.
Let’s create table with some columns.
CREATE TABLE `tbl_members` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(120) DEFAULT NULL,
`email` varchar(120) DEFAULT NULL,
`mobile` varchar(45) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Successfully, we have created a table. Let’s connect with the application.
Read More: How To Cache Database Query Using CodeIgniter Cache?
Database Connection
Open .env file from project root.
Search for DATABASE. You should see the connection environment variables into it. Put your updated details of database connection string values.
#-------------------------------------------------------------------- # DATABASE #-------------------------------------------------------------------- database.default.hostname = localhost database.default.database = codeigniter4_app database.default.username = admin database.default.password = admin database.default.DBDriver = MySQLi database.default.DBPrefix = database.default.port = 3306
Now, database successfully connected with the application.
Create Model
Open project into terminal and run this spark command to create model.
php spark make:model Member --suffix
It will create MemberModel.php inside /app/Models folder. Open MemberModel.php and write this complete code into it.
<?php namespace App\Models; use CodeIgniter\Model; class MemberModel extends Model { protected $DBGroup = 'default'; protected $table = 'tbl_members'; protected $primaryKey = 'id'; protected $useAutoIncrement = true; protected $insertID = 0; protected $returnType = 'array'; protected $useSoftDelete = false; protected $protectFields = true; protected $allowedFields = [ "name", "email", "mobile" ]; // Dates protected $useTimestamps = false; protected $dateFormat = 'datetime'; protected $createdField = 'created_at'; protected $updatedField = 'updated_at'; protected $deletedField = 'deleted_at'; // Validation protected $validationRules = []; protected $validationMessages = []; protected $skipValidation = false; protected $cleanValidationRules = true; // Callbacks protected $allowCallbacks = true; protected $beforeInsert = []; protected $afterInsert = []; protected $beforeUpdate = []; protected $afterUpdate = []; protected $beforeFind = []; protected $afterFind = []; protected $beforeDelete = []; protected $afterDelete = []; }
Model is pointing tbl_members table. We have specified all the table columns into $allowedFields. If suppose we don’t specify then it restrict that missing field from insertion.
Create Layout File
Let’s create a view file add-member.php inside /app/Views folder.
Open file and write this complete code into it.
<!DOCTYPE html> <html lang="en"> <head> <title>CodeIgniter 4 Form Validation Example Tutorial</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> <style> p.error{ color:red; } </style> </head> <body> <div class="container"> <h3>Add Member</h3> <div class="panel panel-primary"> <div class="panel-heading">Add Member</div> <div class="panel-body"> <?php if(session()->has("success")){ ?> <div class="alert alert-success"><?php echo session()->get("success"); ?></div> <?php } ?> <?php if(session()->has("error")){ ?> <div class="alert alert-danger"><?php echo session()->get("error"); ?></div> <?php } ?> <form class="form-horizontal" method="post" action="<?php echo base_url('add-member'); ?>"> <div class="form-group"> <label class="control-label col-sm-2" for="name">Name:</label> <div class="col-sm-6"> <input type="text" class="form-control" id="name" name="name" placeholder="Enter name"> <?php if (isset($validation) && $validation->hasError('name')){ ?> <p class="error"><?php echo $validation->getError('name'); ?></p> <?php } ?> </div> </div> <div class="form-group"> <label class="control-label col-sm-2" for="email">Email:</label> <div class="col-sm-6"> <input type="email" class="form-control" id="email" name="email" placeholder="Enter email"> <?php if (isset($validation) && $validation->hasError('email')){ ?> <p class="error"><?php echo $validation->getError('email'); ?></p> <?php } ?> </div> </div> <div class="form-group"> <label class="control-label col-sm-2" for="mobile">Mobile:</label> <div class="col-sm-6"> <input type="text" class="form-control" id="mobile" name="mobile" placeholder="Enter mobile"> <?php if (isset($validation) && $validation->hasError('mobile')){ ?> <p class="error"><?php echo $validation->getError('mobile'); ?></p> <?php } ?> </div> </div> <div class="form-group"> <div class="col-sm-offset-2 col-sm-10"> <button type="submit" class="btn btn-success">Submit</button> </div> </div> </form> </div> </div> </div> </body> </html>
Code Explanation
- if(session()->has(“success”)){} – Checking for success key of temporary stored message.
- session()->get(“success”) – Print session flash – success key message
- if(session()->has(“error”)){} – Checking for success key of temporary stored message.
- session()->get(“error”) – Print session flash – error key message
- base_url(‘add-member’) – Site URL with add-member route
- if(isset($validation)){} – Checking validation variable if it contains any error
- $validation->hasError(‘mobile’) – Checking for error message for mobile specific field
- $validation->getError(‘mobile’) – Print the validation error message for mobile field.
Create Controller
Next we need to setup application controller.
Loading url Helper
Open BaseController.php from /app/Controllers folder. Search for $helpers, inside this helpers array simply add this url helper.
protected $helpers = [‘url’];
This url helper will provide base_url() and site_url() functions to use.
Also we have the option to load any helper directly to any controller using helper() function.
Back to terminal and run this spark command.
php spark make:controller Member --suffix
It will create MemberController.php inside /app/Controllers folder. Open MemberController.php and write this complete code into it.
<?php namespace App\Controllers; use App\Models\MemberModel; class MemberController extends BaseController { public function addMember() { helper(["url"]); if ($this->request->getMethod() == "post") { $memberModel = new MemberModel(); $rules = [ "name" => "required|min_length[3]|max_length[255]", "email" => "required|valid_email", "mobile" => "required", ]; // If we want to use custom messages for errors // otherwise by default system will take care automatically $messages = [ "name" => [ "required" => "Name is required", "max_length" => "Maximum length of Name is 255 chars", ], "email" => [ "required" => "Email is required", ], "mobile" => [ "required" => "Mobile Number is required", ], ]; $session = session(); // loading session service if (!$this->validate($rules, $messages)) { return view("add-member", [ "validation" => $this->validator, ]); } else { $data = [ "name" => $this->request->getVar("name"), "email" => $this->request->getVar("email"), "mobile" => $this->request->getVar("mobile"), ]; if ($memberModel->insert($data)) { $session->setFlashdata("success", "Data saved successfully"); } else { $session->setFlashdata("error", "Failed to save data"); } return redirect()->to('add-member'); } } return view("add-member"); } }
All available form validation rules for input fields Click here to go.
Code Explanation
- if ($this->request->getMethod() == “post”) {} – Checking request method type. Same method we are using for GET and POST.
- $memberModel = new MemberModel(); – Creating Model instance
- $rules = [] – Defining rules for input fields
- $messages = [] – Defining error message (user defined)
- if (!$this->validate($rules, $messages)) {} – Validating input field with rules and messages. It returns false when input fields not satisfies the defined rules of $rules = []; If it satisfies all conditions then return true value.
- “validation” => $this->validator – Validator returns the form validation error message, we are simply assigning to validation key.
- $this->request->getVar(“name”) – Reading value of input field with “name” attribute.
- if ($memberModel->insert($data)) {} – Saving data to table and returning it’s status after save.
- return redirect()->to(‘add-member’); – Redirecting to application to /add-member page.
Read More: Learn CodeIgniter 4 Tutorial From Beginners To Advance
Add Route
Open Routes.php from /app/Config folder. Add this route into it.
//... $routes->match(["get", "post"], "add-member", "MemberController::addMember"); //...
Application Testing
Open project terminal and start development server via command:
php spark serve
Open application into browser.
URL: http://localhost:8080/add-member
Form View (Submitted with no value)
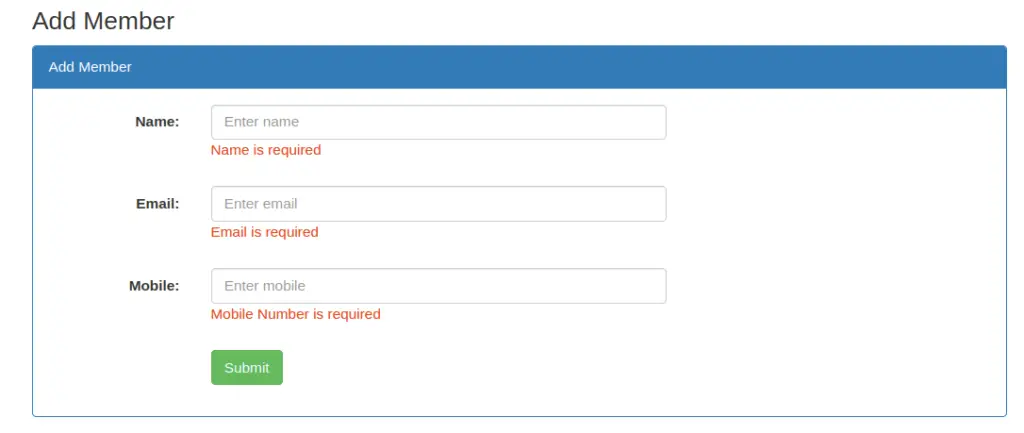
Form submitted with values
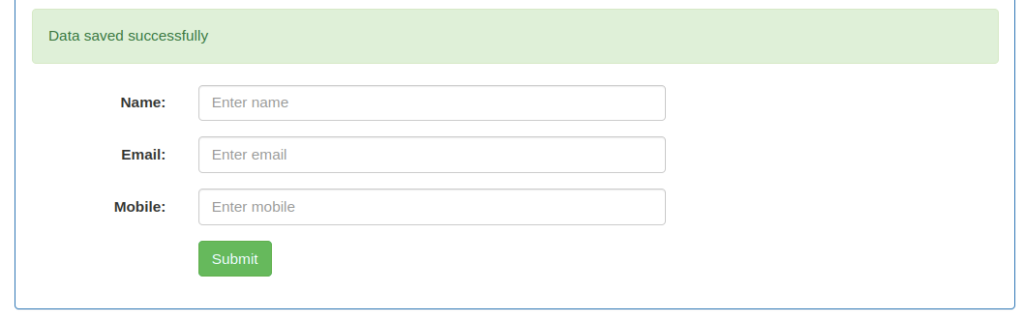
In database table,
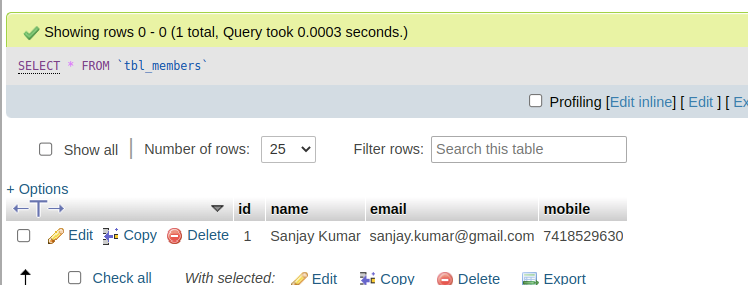
That’s it.
We hope this article helped you to learn about CodeIgniter 4 How To Work with Form Validation Library in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.