Node.js is a popular runtime for building scalable and efficient online applications. Express.js, a Node.js web application framework, makes it easier to create HTTP servers and handle web requests.
We will walk you through the process of constructing an HTTP web server in Node.js using Express.js, allowing you to develop strong and configurable online apps.
Before we get into the details, it’s necessary to understand the importance of creating an HTTP web server. An HTTP server is a critical component of online applications that handles incoming requests and serves responses, allowing users to interact with your application via the web.
Read More: Axios HTTP POST Request in Node Js Tutorial
Let’s get started.
What is HTTP Web Server and Why it is Important?
An HTTP web server, often known as a web server, is software or hardware that acts as the backbone of the World Wide Web. Its principal job is to handle incoming HTTP requests from clients (usually web browsers) and react by serving web pages, files, or other resources.
The following are the main components and functions of an HTTP web server:
- Request Handling: When a client (such as a web browser) sends an HTTP request for a specific resource (such as a web page or an image), the request is received by the web server.
- Resource Retrieval: The web server locates the requested resource on its file system or, if applicable, retrieves it from a database.
- Response Generation: After obtaining the resource, the web server generates an HTTP response. This answer contains an HTTP status code (showing whether the request was successful or unsuccessful), headers (information about the response), and the actual content of the resource.
Importance of HTTP Web Servers,
- Content Delivery: Web servers function as the gatekeepers for web content. They are in charge of providing users with web pages, graphics, stylesheets, scripts, and other resources. Accessing websites and online resources would be impossible without web servers.
- Availability: Web servers ensure that web content is available to users 24 hours a day, seven days a week, allowing users to access websites and web applications at any time. This kind of accessibility is critical for enterprises, e-commerce, and information dissemination.
- Performance: High-performance web servers, such as Nginx and LiteSpeed, can manage a huge number of concurrent connections while also delivering content swiftly. This helps to provide a seamless and responsive user experience.
- Scalability: To meet growing user traffic, web servers can be extended horizontally (by adding more servers) or vertically (by increasing server resources). This scalability is essential for dealing with traffic spikes and expansion.
- Security: Web servers are essential for securing web applications. To protect data during transmission, they can impose security measures such as SSL/TLS encryption. They also aid in the filtering and blocking of harmful requests, which aids in the defence against cyberattacks.
What is Node js and Express js?
Node.js is an open-source server-side runtime environment that allows developers to use JavaScript to create fast and scalable network applications. Node.js, developed by Google on the V8 JavaScript engine, has achieved broad popularity due to its efficiency, speed, and diversity.
Express.js, also known simply as Express, is a lightweight and adaptable Node.js online application framework. It has a comprehensive collection of features and tools for developing web and API apps. Express speeds the building of RESTful APIs and simplifies basic web development chores.
Setup Web Server in Node js Using Express
Create an application folder.
Step #1 (Initialize NPM)
Run this command to create package.json file,
npm init -y
It will a file which manages your application packages.
Step #2 (Install Express Js Library)
Now, let’s install express into it.
npm install express
Step #3 (Application Setup)
Create app.js file,
Load “express” Module,
const express = require('express');
Read More: How To Read and Write Json File in Node Js Tutorial
Create express “Instance”,
Let’s create a new variable to store our Express application i.e app.
const app = express();
Define Web Server “Port”,
const PORT = 8087;
Once, we run this application it will listen application’s request at this given port 8087.
Setup “Home Page” Route
Let’s stay with a simple text response for now. Simply displaying text in the browser
// Welcome page route
app.get("/", (req, res) => {
res.json({
status: true,
message: "Welcome to Landing page of application"
})
});
Listen to Http Web Server on PORT
The server is currently not operational. If we ran the application, we would never be able to view it in the browser.
To start the server, we must utilise one more method on the app that will only be used once in our application, app.listen().
// Listen application requests
app.listen(PORT, () => {
console.log("Application started");
});
Start the Node Server
We can start up the server by using node. Go to project terminal and run this command.
node app.js
Here, are all the steps explained to create and run application on HTTP web server.
Run Node application on HTTP Web Server
Here, is the complete code.
const express = require("express"); const app = express(); const PORT = 8087; // Welcome page route app.get("/", (req, res) => { res.json({ status: true, message: "Welcome to Landing page of application" }) }); // Listen application requests app.listen(PORT, () => { console.log("Application started"); });
Start server,
node app.js
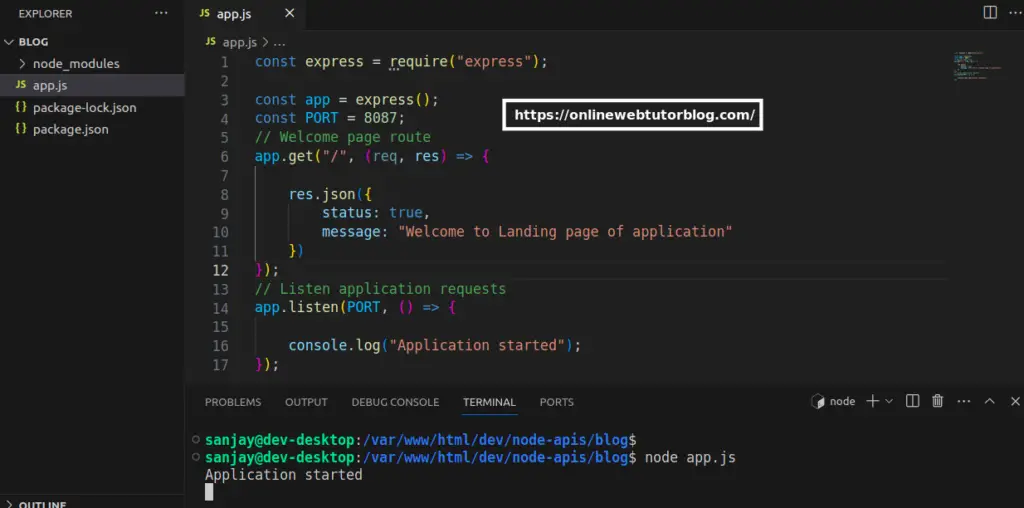
Output
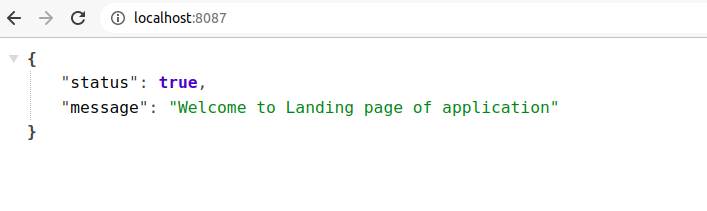
That’s it.
We hope this article helped you to learn Create HTTP Web Server in Node js Using Express js in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.