Inside this article we will see how to build a simple web server in node js. Article contains classified information about creating a web server in node programming.
Every application has a way to start it’s own in-built development server. Here, in the same way we will in Node js. To understand this concept we will create a small code snippet in node js and execute into a simple web server of it.
Node JS is an environment where we can deploy and execute javascript based applications. You need to remember that NodeJS is not a framework, and it’s not a programming language. Node.js is mostly used in server-side programming.
To execute application in node js environment, make sure Node & NPM installed in your system
Learn More –
- Create Models in Node Js Sequelize ORM
- How To Connect MySQL Database in Node Js Application
- How to Download Image from URL in Node Js Tutorial
- How to Generate QR Code in Node JS Tutorial
Let’s get started.
What is a Web Server?
A web server is a computer that runs websites. It’s a computer program that distributes web pages as they are requisitioned. The basic objective of the web server is to store, process and deliver web pages to the users.
In Node Js we have two ways to build a web server to run application –
- Using http inbuilt module
- Using express third party module
We will see both of these to create a web server.
Web Server: Using http inbuilt module
In this we have HTTP and HTTPS, these two inbuilt modules of node js are used to create a simple web server.
Create a folder node-http in your localhost directory. Open this folder into terminal or command prompt.
Next, we need package.json file. Run this given command into terminal
$ npm init -y
The given command will auto generate package.json file with default values. It will look like this –
{ "name": "node-http", "version": "1.0.0", "description": "", "main": "index.js", "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "keywords": [], "author": "", "license": "ISC" }
Create a file index.js and write this following code into it.
// Importing the http module const http = require("http") // Creating server const server = http.createServer((req, res) => { // Sending the response res.write("This is the response from the server") res.end(); }) // Server listening to port 3000 server.listen((3000), () => { console.log("Server is Running"); })
Here,
We can see we have used http module. Started server to listen at 3000 port. Back to terminal and run this command into it.
$ node index.js
Output
URL: http://localhost:3000/
This is the response from the server
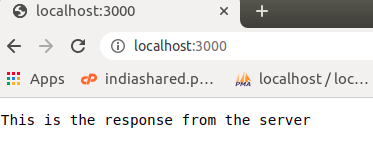
Web Server: Using express third party module
Create a folder node-express in your localhost directory. Open this folder into terminal or command prompt.
Next, we need package.json file. Run this given command into terminal
$ npm init -y
The given command will auto generate package.json file with default values.
Let’s install express third party NPM package.
$ npm install express
It installs into application and create a node_modules folder into it.
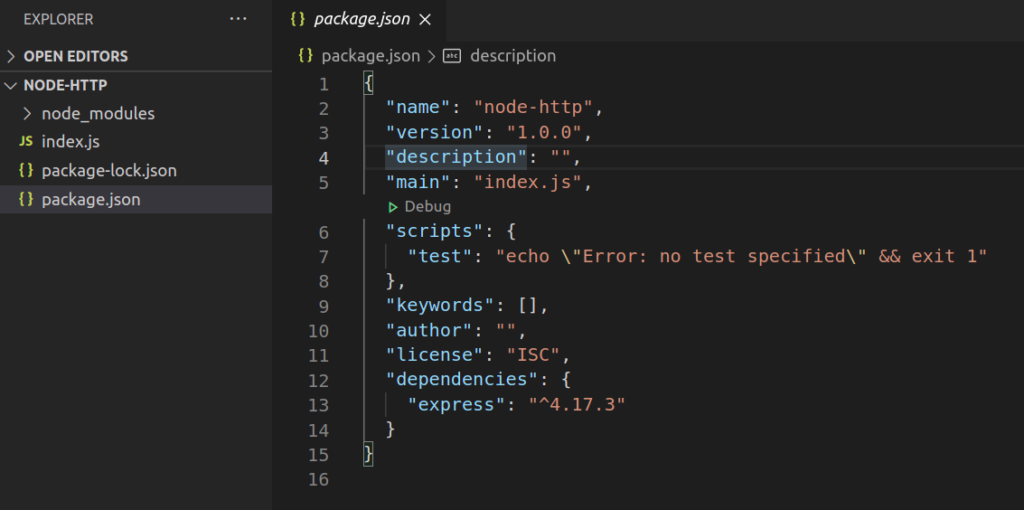
Open index.js and write this code into it.
// Importing express module const express = require("express") const app = express() // Handling GET / request app.use("/", (req, res, next) => { res.send("This is the express server") }) // Handling GET /hello request app.get("/hello", (req, res, next) => { res.send("This is the hello response"); }) // Server setup app.listen(3000, () => { console.log("Server is Running") })
Here,
We can see we have used express module. Started server to listen at 3000 port. Back to terminal and run this command into it.
$ node index.js
Output
URL: http://localhost:3000/
This is the express server
We hope this article helped you to learn How to Build a Simple Web Server with Node js Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.