Inside this article we will see the concept i.e How To Check a Prime Number in PHP Tutorial. Article contains the classified information about a program which checks a number is prime or not using PHP.
If you are looking for a solution to a PHP Program To Check Whether a Number is Prime or Not then this article will help you a lot for this. Tutorial is super easy to understand and implement it in your code as well.
What is a Prime Number?
A prime number is a whole number greater than 1 whose only factors are 1 and itself. A factor is a whole number that can be divided evenly into another number. The first few prime numbers are 2, 3, 5, 7, 11, 13, 17, 19, 23 and 29.
Learn More –
- Laravel 9 to_route() and str() New Helper Function Tutorial
- Laravel 9 Redirect to Route From Controller Example Tutorial
- Laravel 9 YajraBox Datatable Custom Filter and Search Tutorial
- Laravel 9 How To Add Conditional Class in Blade Template
Let’s get started.
Example: Using PHP To Check Prime Numbers
Here,
We will use the concept of PHP i.e How To Check a Prime Number in PHP.
Create a file with name index.php inside your localhost directory. Open file and write this code into it.
<?php function IsPrimeOrNot($n) { for($i=2; $i<$n; $i++) { if ($n % $i ==0) { return 0; } } return 1; } $result = IsPrimeOrNot(5); if ($result==0){ echo 'This is not a Prime Number.'; }else{ echo 'This is a Prime Number.'; }
Output
This is a Prime Number.
Example: Check Prime Number in PHP using a Form
Here we will create a PHP program with PHP Forms for how to check a whether a given number is prime or not.
<html> <head> <title>Prime Number using Form in PHP</title> </head> <body> <form action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>" method="post"> Enter a Number: <input type="text" name="input"><br><br> <input type="submit" name="submit" value="Submit"> </form> <?php if ($_POST) { $input = $_POST['input']; for ($i = 2; $i <= $input - 1; $i++) { if ($input % $i == 0) { $value = True; } } if (isset($value) && $value) { echo 'The Number ' . $input . ' is not prime'; } else { echo 'The Number ' . $input . ' is prime'; } } ?> </body> </html>
Outputb
We entered a value 5 into input and submit:
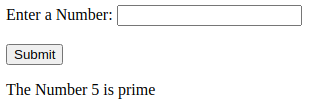
Example: Get Prime Numbers Between 1 and 100
Here,
We have created a function checkPrime() to check whether the number is prime or not.
We loop over the number range (1 to 100) and pass each as a parameter to the function to check whether a number is prime or not.
<?php function checkPrime($num) { if ($num == 1) return 0; for ($i = 2; $i <= $num / 2; $i++) { if ($num % $i == 0) return 0; } return 1; } echo '<h2>Prime Numbers between 1 and 100</h2> '; for ($num = 1; $num <= 100; $num++) { $flag = checkPrime($num); if ($flag == 1) { echo $num . " "; } }
Output

We hope this article helped you to learn How To Check a Prime Number in PHP Example Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.