Inside this article we will see the concept i.e Laravel 9 YajraBox Datatable Custom Filter and Search Tutorial. Article contains the classified information about yajrabox datatable implementation in Laravel 9 with custom search filter.
If you are looking for a solution to use yajrabox datatable of laravel 9 for custom search filter then this article will help you a lot for this. Tutorial is super easy to understand and implement it in your code as well.
Yajrabox datatable in laravel is a composer package which installs server side datatable with all features like Search, Sort, Pagination, etc.
Learn More –
- Laravel 9 to_route() and str() New Helper Function Tutorial
- Laravel 9 Redirect to Route From Controller Example Tutorial
- Javascript Show Hide Div on Select Option Example Tutorial
- Laravel 9 How To Add Conditional Class in Blade Template
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Generate Fake Data
To generate fake data for datatable, we will use the concept of Tinker shell. We will generate fake data for users table.
Assuming you have users table created in your application database. And also your application contains User.php model file inside /app/Http/Models folder.
Open Tinker Shell:
$ php artisan tinker
Generate 200 fake rows for users table:
>>> User::factory()->count(200)->create()
users table data view
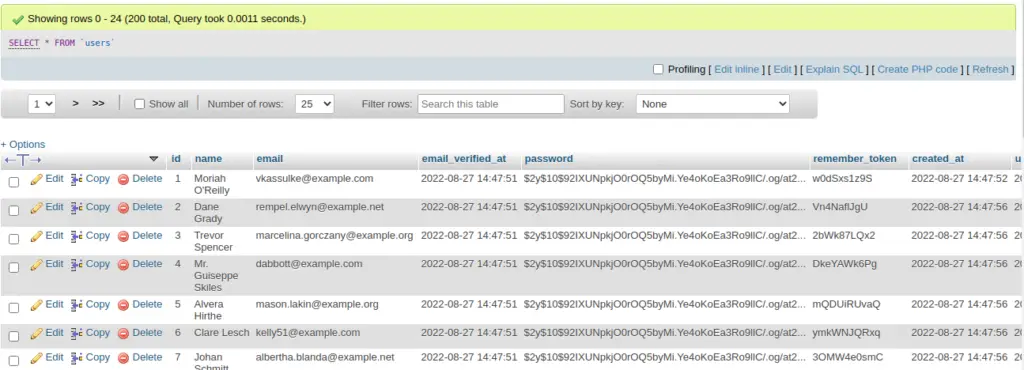
Install YajraBox Datatable
To install yajrabox, we need to run a composer command. Open up the project terminal and type this command and execute.
$ composer require yajra/laravel-datatables-oracle
While installation of this package, you will get such type of screen over terminal.
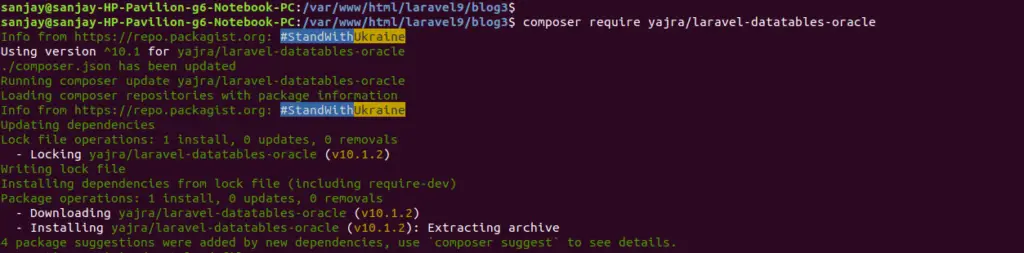
Create Controller
Open project into terminal and run this command into it.
$ php artisan make:controller UserController
It will create UserController.php file inside /app/Http/Controllers folder. Open controller file and write this code into it.
<?php namespace App\Http\Controllers; use App\Models\User; use Illuminate\Http\Request; use Yajra\DataTables\DataTables; use Illuminate\Support\Str; class UserController extends Controller { /** * Display a listing of the resource. * * @return \Illuminate\Http\Response */ public function index(Request $request) { if ($request->ajax()) { $data = User::latest()->get(); return Datatables::of($data) ->addIndexColumn() ->filter(function ($instance) use ($request) { if (!empty($request->get('email'))) { $instance->collection = $instance->collection->filter(function ($row) use ($request) { return Str::contains($row['email'], $request->get('email')) ? true : false; }); } if (!empty($request->get('search'))) { $instance->collection = $instance->collection->filter(function ($row) use ($request) { if (Str::contains(Str::lower($row['email']), Str::lower($request->get('search')))) { return true; } else if (Str::contains(Str::lower($row['name']), Str::lower($request->get('search')))) { return true; } return false; }); } }) ->addColumn('action', function ($row) { $btn = '<a href="javascript:void(0)" class="edit btn btn-primary btn-sm">View</a>'; return $btn; }) ->rawColumns(['action']) ->make(true); } return view('users'); } }
Create Template
Create a fie users.blade.php inside /resources/views folder.
Open users.blade.php and write this content into it.
<!DOCTYPE html> <html> <head> <title>Laravel 9 YajraBox Datatable Custom Filter and Search Tutorial</title> <meta name="csrf-token" content="{{ csrf_token() }}"> <link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/datatables/1.10.21/css/jquery.dataTables.min.css" integrity="sha512-1k7mWiTNoyx2XtmI96o+hdjP8nn0f3Z2N4oF/9ZZRgijyV4omsKOXEnqL1gKQNPy2MTSP9rIEWGcH/CInulptA==" crossorigin="anonymous" referrerpolicy="no-referrer" /> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery-validate/1.19.0/jquery.validate.js"></script> <script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.min.js" integrity="sha384-cVKIPhGWiC2Al4u+LWgxfKTRIcfu0JTxR+EQDz/bgldoEyl4H0zUF0QKbrJ0EcQF" crossorigin="anonymous"> </script> <script src="https://cdnjs.cloudflare.com/ajax/libs/datatables/1.10.21/js/jquery.dataTables.min.js"></script> <style type="text/css"> body { background-color: #b4d7bd; } </style> </head> <body> <div class="container py-5"> <div class="card"> <div class="card-header text-center"> <h3 style="font-size: 19px;padding: 10px;">Laravel 9 YajraBox Datatable Custom Filter and Search Tutorial</h3> </div> <div class="card-body"> <input type="text" name="email" class="form-control searchEmail" placeholder="Search for Email Only..."> <br> <table class="table table-bordered data-table"> <thead> <tr> <th>No</th> <th>Name</th> <th>Email</th> <th width="100px">Action</th> </tr> </thead> <tbody> </tbody> </table> </div> </div> </div> </body> <script type="text/javascript"> $(function() { var table = $('.data-table').DataTable({ processing: true, serverSide: true, ajax: { url: "{{ route('users.index') }}", data: function(d) { d.email = $('.searchEmail').val(), d.search = $('input[type="search"]').val() } }, columns: [{ data: 'DT_RowIndex', name: 'DT_RowIndex' }, { data: 'name', name: 'name' }, { data: 'email', name: 'email' }, { data: 'action', name: 'action', orderable: false, searchable: false }, ] }); $(".searchEmail").keyup(function() { table.draw(); }); }); </script> </html>
Add Route
Open web.php file from /routes folder. Add this route into it.
//... use App\Http\Controllers\UserController; //... Route::get('users', [UserController::class, 'index'])->name("users.index"); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/users

Search data inside table
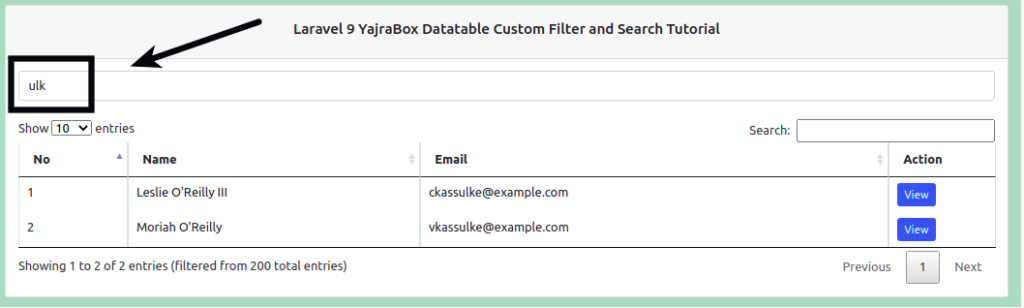
We hope this article helped you to learn Laravel 9 YajraBox Datatable Custom Filter and Search Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.