This post will teach you all you need to know about working with emails, including how to send emails with GMail SMTP and PHPMailer 6 in Laravel 10.
Every website requires communication, and the ability to send emails in a reliable and effective manner is crucial for user engagement and notification systems.
In this post, we will walk you through the process of integrating GMail’s SMTP (Simple Mail Transfer Protocol) server with Laravel 10 and PHPMailer 6 (a sophisticated PHP email-sending tool).
Read More: CodeIgniter 4 Send Emails via GMail SMTP and PHPMailer 6
By implementing GMail SMTP and PHPMailer 6 into your Laravel 10 project, you can ensure seamless email delivery and take advantage of full email features.
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
How Do I Configure A Google App Password?
Google App Password is a 16-digit alphanumeric number that allows you to sign in to your Google Account from apps that do not support two-factor authentication.
If you enable app password, you will never have to reveal your primary account password again. App Passwords are only available to accounts with 2-Step Verification enabled.
Here’s how to create an app password so you can send and receive emails on your server with PHPMailer.
Step #1 Login to Google Account
Go to myaccount.google.com (if not signed in, you will be asked to sign-in to your account)
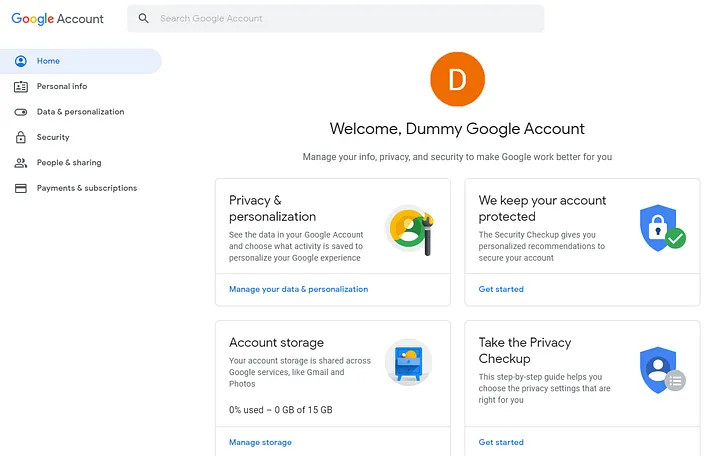
Step #2 Enable 2-Step Verification
Before you can set up the app password, you must first enable 2-Step Verification on your account.
Read More: Create Automated Logs Every 2 hours in CodeIgniter 4 Tutorial
To proceed to the Security page, click on the Security tab on the left-hand side and then on 2-Step Verification under the section Signing in to Google.
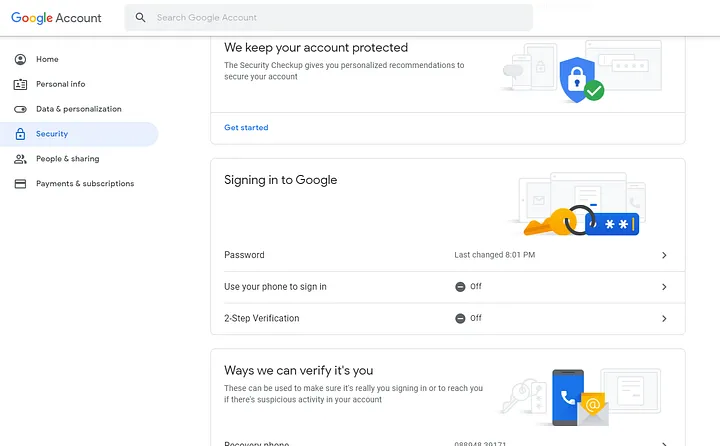
Click on Get Started and on the next page enter your password to verify your account and hit Next
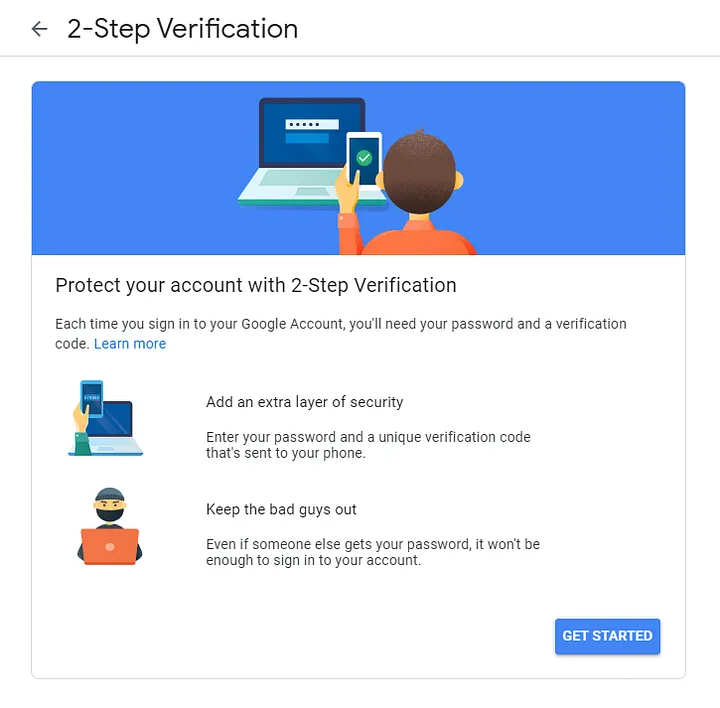
You must now configure your phone.
Enter the phone number you want to use for verification, then choose how you want to get your code. It might be a text or a phone call. I’ll keep it as a text message and press Next.
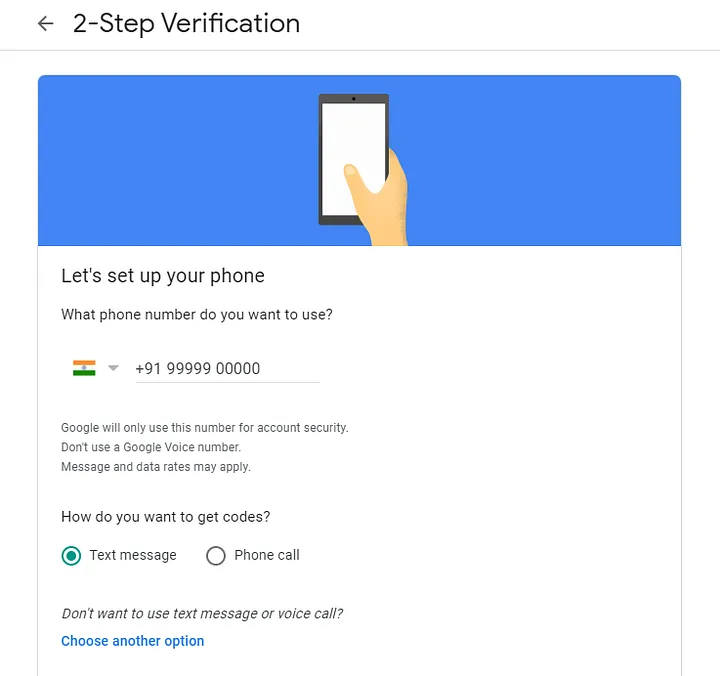
Google will give you a verification code through text message. Enter the verification code and then click Next.
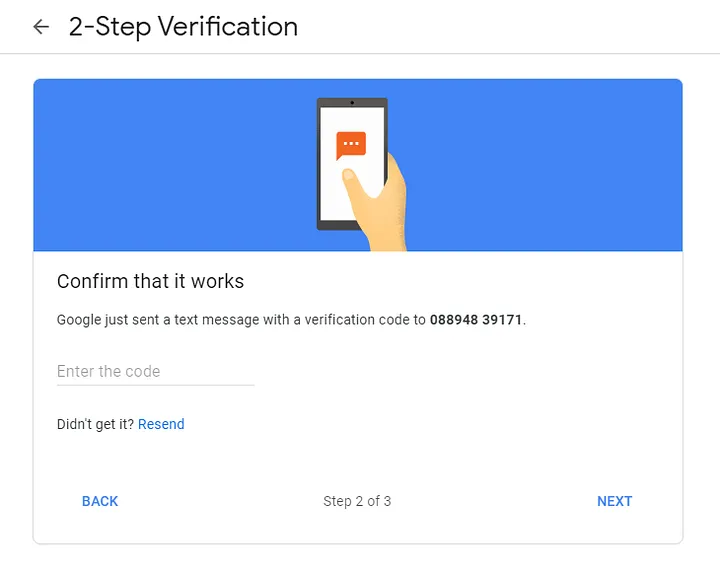
Google will confirm that 2-Step Verification is enabled on the next page.
Read More: CodeIgniter 4 RESTful API Using Shield Authentication
To return to the Security page, click the back arrow next to the header.
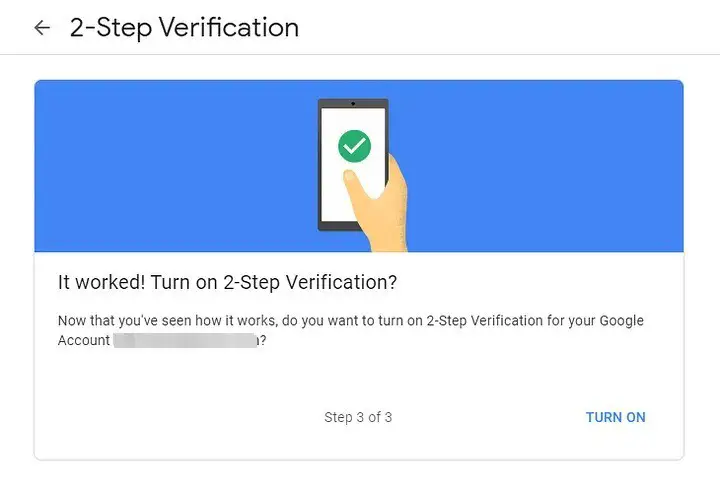
Step #3 Setup Application password
Within the section Signing in to Google, you will notice a new option that says App passwords.
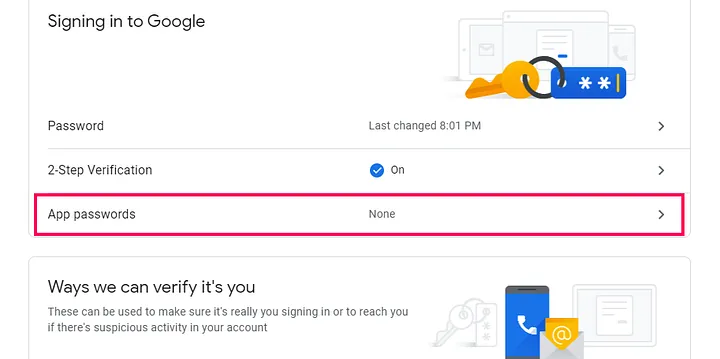
In case, if you are not able to see this App password there. Click on 2-Step Verification.
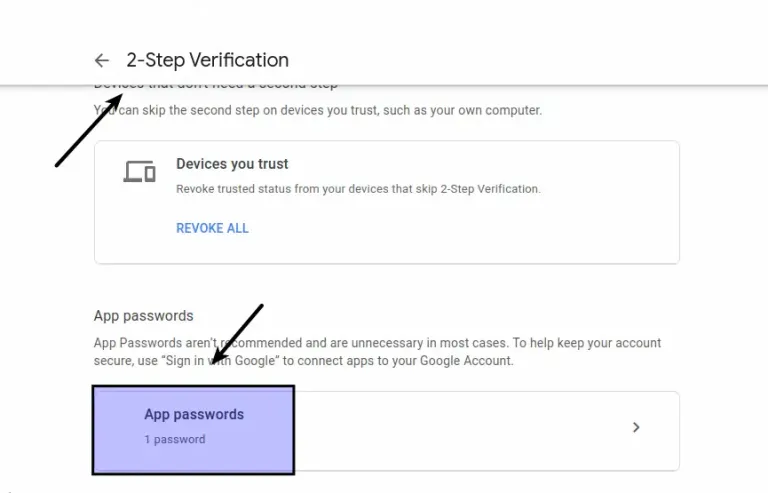
Verify your account by clicking on App passwords. You will be able to create app passwords for any app and device after verification.
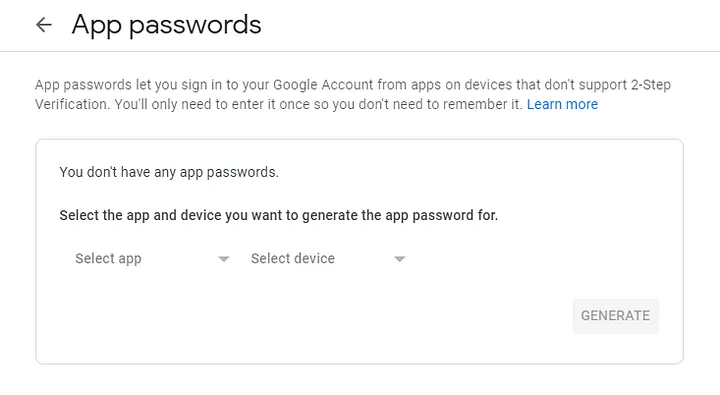
Select Mail from the Select app and Other (custom name) from the Select device, and then give it a name. You can call it whatever you want; I’ll call it PHPMailer and click Generate.
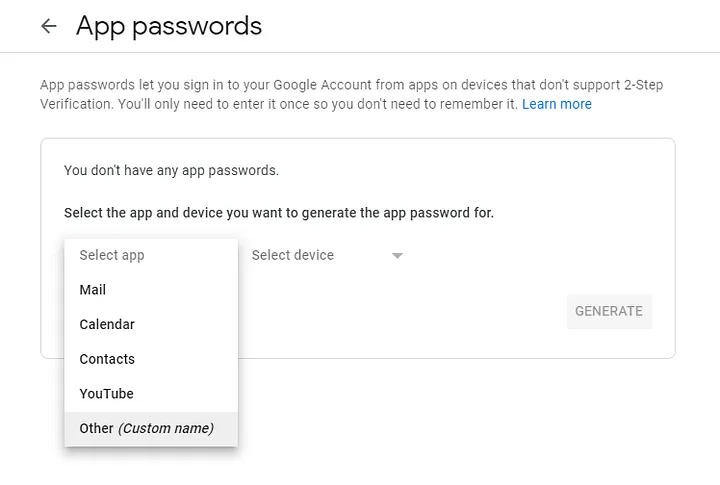
You have now successfully created your app password. Copy and paste this code somewhere safe until you’ve added it to the PHP script.
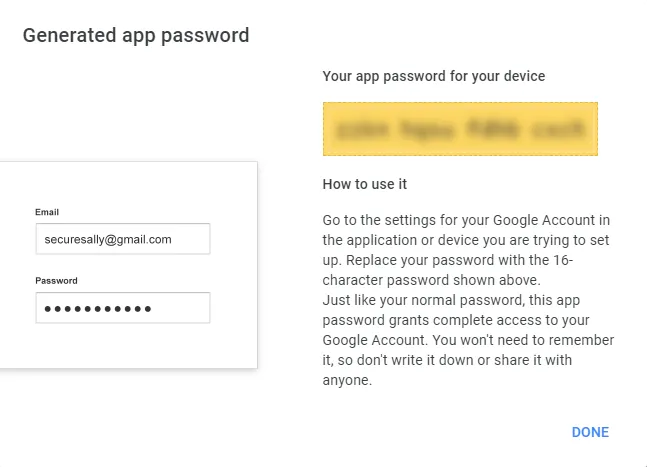
Installation of PHPMailer To Application
Open project into terminal and run this command to install PHPMailer.
$ composer require phpmailer/phpmailer
While installation you will see in your terminal as
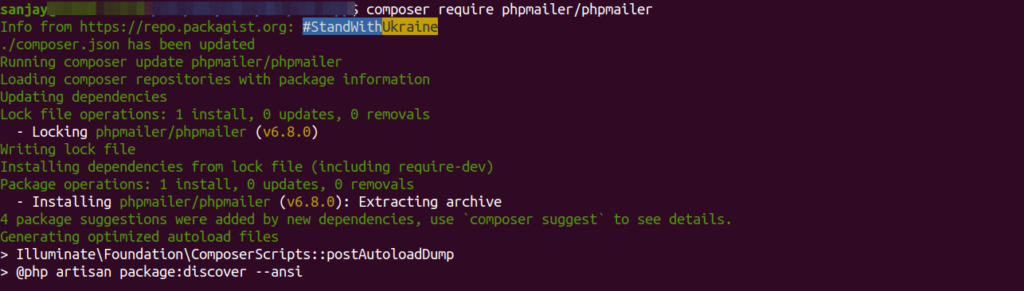
Read More: How To Use Laravel 10 Collection count() and countBy() Methods
Next, we will configure this package and use it to send emails.
Email Controller Setup
Back to terminal and run this command –
$ php artisan make:controller EmailController
It will create EmailController.php file inside /app/Http/Controllers folder.
Open EmailController.php and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use PHPMailer\PHPMailer\PHPMailer; class EmailController extends Controller { public function sendMail() { $mail = new PHPMailer(true); // Server settings $mail->isSMTP(); $mail->SMTPDebug = 0; $mail->Debugoutput = 'html'; $mail->Host = 'smtp.gmail.com'; $mail->SMTPAuth = true; $mail->SMTPSecure = 'tls'; $mail->Port = 587; $mail->Username = 'ENTER YOUR GOOGLE ACCOUNT EMAIL'; $mail->Password = 'ENTER YOUR APP PASSWORD HERE'; // Sender & Recipient $mail->From = 'ENTER YOUR GOOGLE ACCOUNT EMAIL'; $mail->FromName = 'SENDER NAME'; $mail->addAddress('RECIPIENT EMAIL'); // Content $mail->isHTML(true); $mail->CharSet = 'UTF-8'; $mail->Encoding = 'base64'; $mail->Subject = 'My Subject'; $body = 'This is a test message sent by <b>Online Web Tutor</b>'; $mail->Body = $body; if ($mail->send()) { echo 'Mail sent successfully'; exit; } else { echo 'Failed to send email'; exit; } } }
Setup Email Route
Open web.php from /routes folder. Add this route into it –
//... use App\Http\Controllers\EmailController; Route::get("send-email", [EmailController::class, "sendMail"]); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/send-email

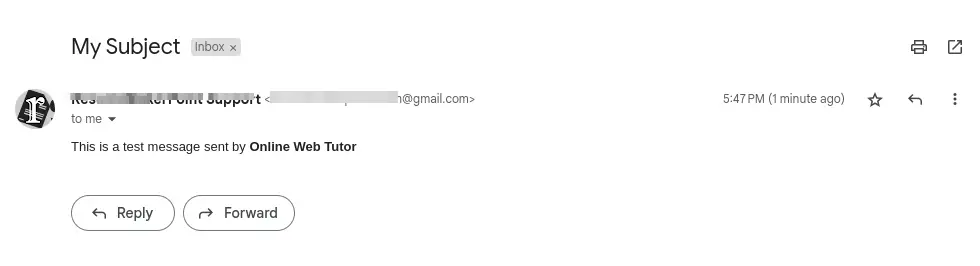
If everything went well, you should be able to send and receive emails directly to your mailbox from your website. There will no longer be any spam emails!
Read More: Learn Laravel 10 Collection filter() Tutorial for Beginners
We hope this article helped you to learn Laravel 10 Send Emails via GMail SMTP and PHPMailer 6 in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.