In this article, we’ll delve into another vital concept of Laravel: Laravel 11 REST API Development Using Sanctum. Here, we’ll provide a comprehensive, step-by-step guide to crafting RESTful services from the ground up.
Representational State Transfer (REST) serves as an architectural style delineating a set of principles for designing web services. REST API, in turn, offers a straightforward and adaptable means of accessing web services without complex processing.
Within this article, our focus will be on establishing a secure suite of REST APIs utilizing Laravel and Sanctum. Sanctum stands as a Laravel composer package pivotal to this process.
Read More: Laravel 11 RESTful APIs with JWT Authentication Tutorial
Here, we will see to create these APIs:
- Register API
- Login API
- Profile API
- Refresh Token API
- Logout API
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Install Sanctum Auth Package and Create “api.php”
Open project terminal and run this command,
php artisan install:api
After installation you will find this package into composer.json file,
"laravel/sanctum": "^4.0",
It will create “api.php” into /routes folder as well as it setup sanctum auth package.
Update “User.php” model file
Add this line,
use Laravel\Sanctum\HasApiTokens;
next, Use this HasApiTokens
Here, is the complete code of model class file.
<?php namespace App\Models; // use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasApiTokens, HasFactory, Notifiable; /** * The attributes that are mass assignable. * * @var array<int, string> */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for serialization. * * @var array<int, string> */ protected $hidden = [ 'password', 'remember_token', ]; /** * Get the attributes that should be cast. * * @return array<string, string> */ protected function casts(): array { return [ 'email_verified_at' => 'datetime', 'password' => 'hashed', ]; } }
Run Migrations (If any pending)
php artisan migrate
It will migrate all pending migrations of application.
Successfully, you have setup Sanctum auth package into application.
Now, you have a middleware which you can use to protect api routes i.e “auth:sanctum”
API Controller Settings
Run this command to create API controller class,
php artisan make:controller Api/ApiController
It will create a file named ApiController.php inside /app/Http/Controllers folder.
Open file and write this complete code into it,
<?php namespace App\Http\Controllers\Api; use App\Http\Controllers\Controller; use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; use App\Models\User; class ApiController extends Controller { // Register (POST - name, email, password) public function register(Request $request){ // Validation $request->validate([ "name" => "required|string", "email" => "required|string|email|unique:users", "password" => "required|confirmed" // password_confirmation ]); // User model to save user in database User::create([ "name" => $request->name, "email" => $request->email, "password" => bcrypt($request->password) ]); // Response return response()->json([ "status" => true, "message" => "User registered successfully" ]); } // Login (POST - email, password) public function login(Request $request){ // Validation $request->validate([ "email" => "required|string|email", "password" => "required" ]); // Check user by email $user = User::where("email", $request->email)->first(); // Check user by password if(!empty($user)){ if(Hash::check($request->password, $user->password)){ // Login is ok $tokenInfo = $user->createToken("myToken"); $token = $tokenInfo->plainTextToken; // Token value return response()->json([ "status" => true, "message" => "Login successful", "token" => $token ]); }else{ return response()->json([ "status" => false, "message" => "Password didn't match." ]); } }else{ return response()->json([ "status" => false, "message" => "Invalid credentials" ]); } } // Profile (GET, Auth Token) public function profile(){ $userData = auth()->user(); return response()->json([ "status" => true, "message" => "Profile information", "data" => $userData ]); } // Logout (GET, Auth Token) public function logout(){ // To get all tokens of logged in user and delete that request()->user()->tokens()->delete(); return response()->json([ "status" => true, "message" => "User logged out" ]); } // Refresh Token (GET, Auth Token) public function refreshToken(){ $tokenInfo = request()->user()->createToken("myNewToken"); $newToken = $tokenInfo->plainTextToken; // Token value return response()->json([ "status" => true, "message" => "Refresh token", "acccess_token" => $newToken ]); } }
ApiController class contains the api methods for,
- Register
- Login
- Profile
- Refresh Token
- Logout
Setup API Routes
Open api.php file from /routes folder. Add these routes into it,
//... use App\Http\Controllers\Api\ApiController; Route::post("register", [ApiController::class, "register"]); Route::post("login", [ApiController::class, "login"]); // Protected Routes Route::group([ "middleware" => ["auth:sanctum"] ], function(){ Route::get("profile", [ApiController::class, "profile"]); Route::get("logout", [ApiController::class, "logout"]); Route::get("refresh-token", [ApiController::class, "refreshToken"]); });
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Register API
URL – http://127.0.0.1:8000/api/register
Method – POST
Header –
Accept:application/json
Form data –
{
"name": "Sanjay Kumar",
"email": "sanjay.owt@gmail.com",
"password": 123456
"password_confirmation": 123456
}
Screenshot –
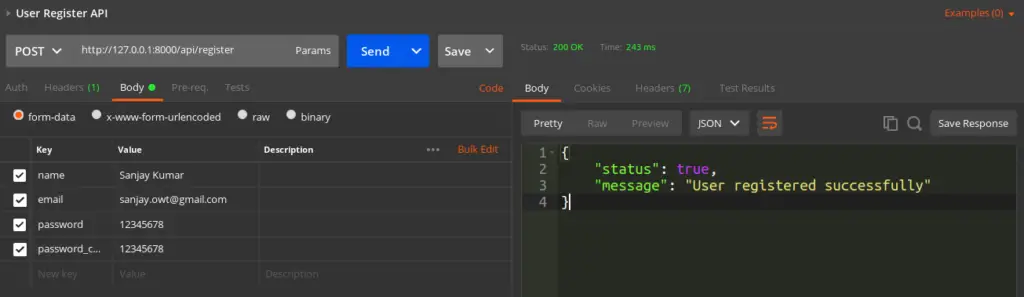
Login API
URL – http://127.0.0.1:8000/api/login
Method – POST
Header –
Accept:application/json
Form data –
{
"email": "sanjay.owt@gmail.com",
"password": 123456
}
Screenshot –
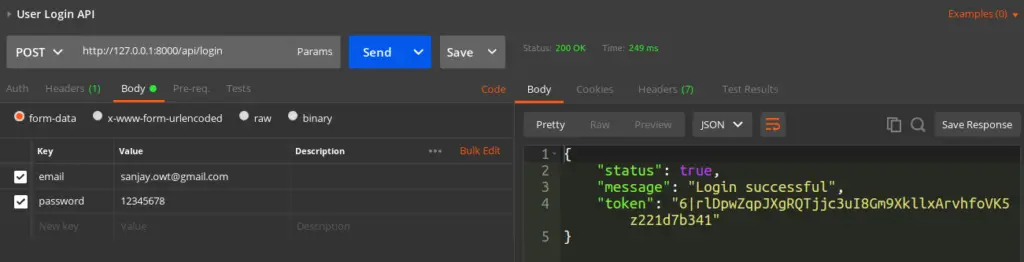
Profile API
URL – http://127.0.0.1:8000/api/profile
Method – GET
Header –
Accept:application/json
Authorization:Bearer <token>
Screenshot –
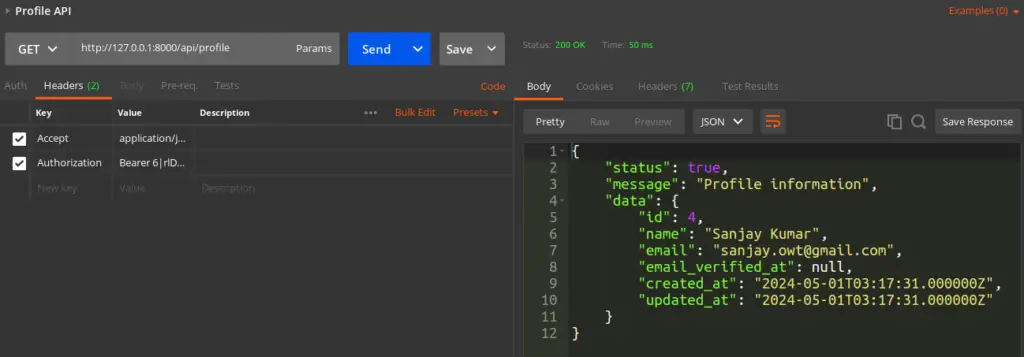
Refresh Token API
URL – http://127.0.0.1:8000/api/refresh-token
Method – GET
Header –
Accept:application/json
Authorization:Bearer <token>
Screenshot –
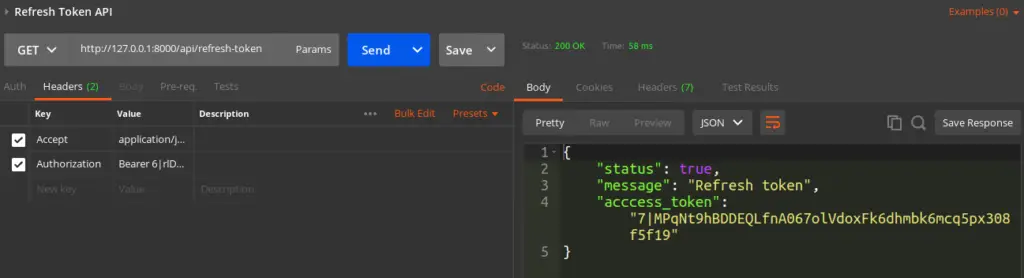
Logout API
URL – http://127.0.0.1:8000/api/logout
Method – GET
Header –
Accept:application/json
Authorization:Bearer <token>
That’s it.
We hope this article helped you to learn about Laravel 11 RESTful APIs with Sanctum Authentication in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.