Inside this article we will see How to add social media share buttons in laravel 9. Social share media button means the button through which we can share content to social platforms like Facebook, Twitter, Instagram etc.
This tutorial will guide you to learn laravel 9 add social media share buttons into a great detail. This is very interesting to see and also super easy to implement.
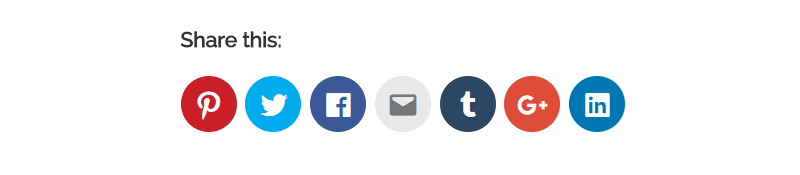
Laravel Share is a composer package. We will use this composer package to work and add social share buttons.
Learn More –
- Laravel 9 How To Connect Multiple Database Connections Tutorial
- Laravel 9 How to Create Custom Blade Directive
- Laravel 9 How to Create Custom Log File Tutorial
- Laravel 9 How to Create Custom Route File Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Model & Migration
Open project into terminal and run this command to create model & migration file.
$ php artisan make:model Post -m
It will create two files –
- Model – Post.php inside /app/Models folder
- Migration file – 2022_04_25_123805_create_post_table.php inside /database/migrations folder.
Open Migration file and write this complete code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('posts', function (Blueprint $table) { $table->id(); $table->string("title", 120); $table->string("url", 225); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('posts'); } };
Open model file Post.php and write this complete code into it.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Post extends Model { use HasFactory; public $timestamps = false; }
Run Migration
Next,
We need to create tables inside database. Run this command to migrate migrations.
$ php artisan migrate
This command will create tables inside database.
Table: posts
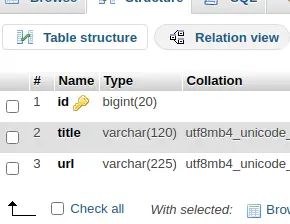
Dummy Data for Application
Here,
You need to copy the given mysql query and run into sql tab of phpmyadmin.
-- -- Dumping data for table `posts` -- INSERT INTO `posts` (`id`, `title`, `url`) VALUES (1, 'Concept of Route Middleware in Laravel 9 Tutorial', 'https://onlinewebtutorblog.com/concept-of-route-middleware-in-laravel-9-tutorial/'), (2, 'Concept of Trait in Laravel 9 Tutorial with Example', 'https://onlinewebtutorblog.com/concept-of-trait-in-laravel-9-tutorial-with-example/'), (3, 'Create Signature Pad & Save Using jQuery in Laravel 9', 'https://onlinewebtutorblog.com/create-signature-pad-save-using-jquery-in-laravel-9/'), (4, 'File or Image Upload Using Livewire Laravel 9 Tutorial', 'https://onlinewebtutorblog.com/file-or-image-upload-using-livewire-laravel-9-tutorial/');
You will see something like this after this test data insertion.
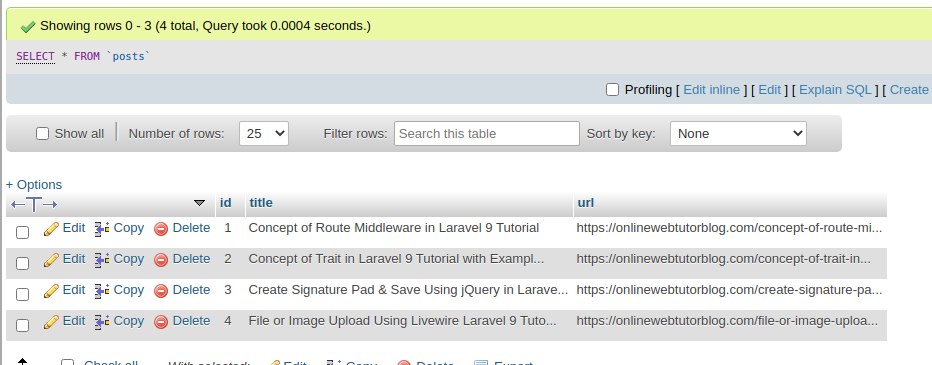
Install Share Package
Open project into terminal and run this command to install Share package.
$ composer require jorenvanhocht/laravel-share
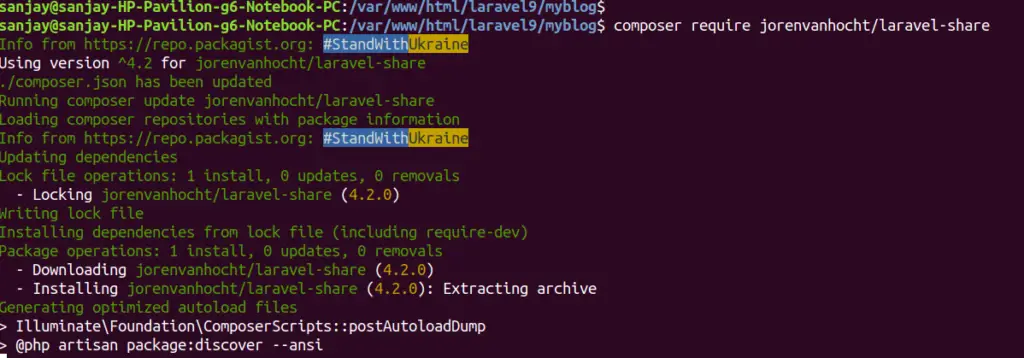
Next,
Publish package
$ php artisan vendor:publish --provider="Jorenvh\Share\Providers\ShareServiceProvider"
Create Controller
Next,
We need to create a controller file.
$ php artisan make:controller SiteController
It will create a file SiteController.php inside /app/Http/Controllers folder.
Open SiteController.php and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Post; use Jorenvh\Share\Share; class SiteController extends Controller { public function sharePosts() { $shareButtons = \Share::page( 'https://onlinewebtutorblog.com/', 'What you are writing, just share to world to learn!!', ) ->facebook() ->twitter() ->linkedin() ->telegram() ->whatsapp() ->reddit(); $posts = Post::get(); return view("share-post", compact('shareButtons', 'posts')); } }
Create Blade Layout File
Go to /resources/views folder and create a file with name share-post.blade.php
Open share-post.blade.php and write this complete code into it.
<!DOCTYPE html> <html lang="en"> <head> <title>Laravel 9 Add Social Media Share Buttons</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.3/css/all.min.css" /> <style> #social-links li { display: inline-block; padding: 4px; text-align: center; list-style: none; } </style> </head> <body> <div class="container"> <h3>Laravel 9 Add Social Media Share Buttons</h3> <div class="panel panel-primary"> <div class="panel-heading">Laravel 9 Add Social Media Share Buttons</div> <div class="panel-body"> {!! $shareButtons !!} <table class="table table-striped"> <thead> <tr> <th>#ID</th> <th>Post</th> <th>URL</th> </tr> </thead> <tbody> @foreach ($posts as $post) <tr> <td> {{ $post->id }} </td> <td> {{ $post->title }} </td> <td> {!! Share::page($post->url, $post->title)->facebook()->twitter()->whatsapp() !!} </td> </tr> @endforeach </tbody> </table> </div> </div> </div> </body> </html>
Add Route
Open web.php from /routes folder and add this route into it.
//... use App\Http\Controllers\SiteController; Route::get("share-posts", [SiteController::class, "sharePosts"]);
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/share-posts
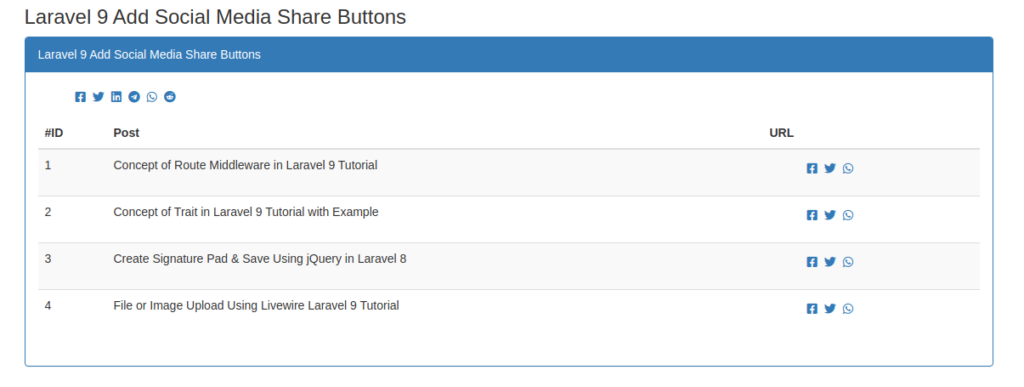
We hope this article helped you to Laravel 9 Add Social Media Share Buttons in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.