Inside this article we will see the concept i.e Laravel 9 How to Insert Multiple Records Example Tutorial. Article contains the classified information about How to use laravel eloquent to insert multiple rows.
If you are looking for a solution i.e How to Create Multiple Records in Laravel then this article will help you a lot for this. Tutorial is super easy to understand and implement it in your code as well.
We will see the concept of multiple data rows insert using Eloquent approach as well as Query Builder approach.
Read More: PHP Remove http, https, www and slashes From URL
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Migration
Open project into terminal and run this command to create a migration file.
$ php artisan make:migration create_posts_table
Above command will create 2022_09_29_212520_create_posts_table.php file inside /database/migrations folder.
Read More: Laravel 9 Order By Multiple Columns Example Tutorial
Open xxx_create_posts_table.php file and write this code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('posts', function (Blueprint $table) { $table->id(); $table->string('title'); $table->text('body'); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('posts'); } };
Run Migration
Back to terminal and run this command for migrations.
$ php artisan migrate
It will create posts table into your database.
Create Model
Back to terminal and run this command to create model.
$ php artisan make:model Post
It will create a model file Post.php inside /app/Models folder.
Open Post.php and write this following code into it.
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Post extends Model { use HasFactory; public $timestamps = false; protected $fillable = [ 'title', 'body' ]; }
Insert Multiple Rows – Eloquent approach
Back to project terminal and run this command to create a controller file.
$ php artisan make:controller PostController
Above command will create a file i.e PostController.php inside /app/Http/Controllers folder.
Read More: How to Create and Use Laravel 9 Macro Example Tutorial
Open PostController.php and write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Post; class PostController extends Controller { public function index() { $data = [ ['title' => 'My Title', 'body' => 'Post Body Added'], ['title' => 'My Title 2', 'body' => 'Post Body Added 2'], ['title' => 'My Title 3', 'body' => 'Post Body Added 2'], ['title' => 'My Title 4', 'body' => 'Post Body Added 2'] ]; Post::insert($data); // Eloquent approach } }
Route
Open web.php from /routes folder. Add this route into it.
//... use App\Http\Controllers\PostController; Route::get("insert-data", [PostController::class, "index"]); //...
URL: http://127.0.0.1:8000/insert-data
Output
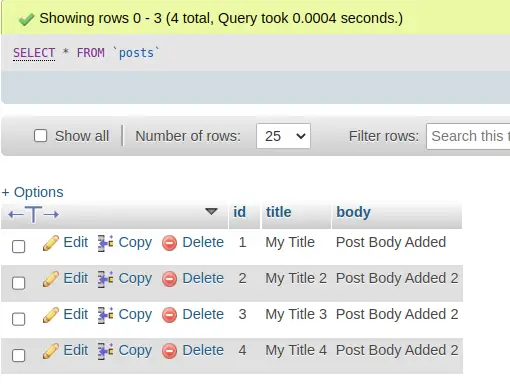
Insert Multiple Rows – Query Builder approach
Open PostController.php and write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\DB; class PostController extends Controller { public function index() { $data = [ ['title' => 'Custom Title', 'body' => 'Post Body New'], ['title' => 'Custom Title 2', 'body' => 'Post Body New 2'], ['title' => 'Custom Title 3', 'body' => 'Post Body New 2'], ['title' => 'Custom Title 4', 'body' => 'Post Body New 2'] ]; DB::table("posts")->insert($data); } }
Route
Open web.php from /routes folder. Add this route into it.
//... use App\Http\Controllers\PostController; Route::get("insert-data", [PostController::class, "index"]); //...
URL: http://127.0.0.1:8000/insert-data
Read More: Laravel 9 How to get Random Database Records Tutorial
Output
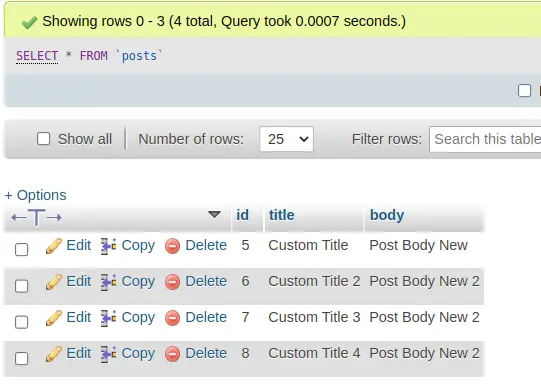
We hope this article helped you to learn Laravel 9 How to Insert Multiple Records Example Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.