Inside this article, we will see the concept of Line Chart Integration in CodeIgniter 4. This article will be step by step graph integration.
Line graph represents the information in very graphical view which provides the complete idea about data. We will use jQuery Highcharts to add Line chart into CodeIgniter 4 application.
To Learn about Pie Chart Integration in CodeIgniter 4, Click here.
Learn More –
- MySQL Group By in CodeIgniter 4 Query Builder Tutorial
- MySQL Like Operator in CodeIgniter 4 Query Builder Tutorial
- MySQL Order By in CodeIgniter 4 Query Builder Tutorial
- Number From International System To Indian System
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Create Database
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
We will use MySQL command to create database. Run this command into Sql tab of PhpMyAdmin.
CREATE DATABASE codeigniter4_app;
Successfully, we have created a database.
Create Database Table
Next, we need a table. That table will be responsible to store data.
Let’s create table with some columns.
CREATE TABLE `students` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL,
`term1_marks` int(11) NOT NULL,
`term2_marks` int(11) NOT NULL,
`term3_marks` int(11) NOT NULL,
`term4_marks` int(11) NOT NULL,
`remarks` varchar(50) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Successfully, we have created a table.
Database Connection
Open .env file from project root.
Search for DATABASE. You should see the connection environment variables into it. Put your updated details of database connection string values.
#-------------------------------------------------------------------- # DATABASE #-------------------------------------------------------------------- database.default.hostname = localhost database.default.database = codeigniter4_app database.default.username = admin database.default.password = admin database.default.DBDriver = MySQLi database.default.DBPrefix = database.default.port = 3306
Now, database successfully connected with the application.
Create Model
Open project into terminal and run this spark command to create model.
$ php spark make:model Student --suffix
It will create StudentModel.php at /app/Models folder.
Open StudentModel.php and write this code.
<?php namespace App\Models; use CodeIgniter\Model; class StudentModel extends Model { protected $DBGroup = 'default'; protected $table = 'students'; protected $primaryKey = 'id'; protected $useAutoIncrement = true; protected $insertID = 0; protected $returnType = 'array'; protected $useSoftDelete = false; protected $protectFields = true; protected $allowedFields = [ "name", "term1_marks", "term2_marks", "term3_marks", "term4_marks", "remarks", ]; // Dates protected $useTimestamps = false; protected $dateFormat = 'datetime'; protected $createdField = 'created_at'; protected $updatedField = 'updated_at'; protected $deletedField = 'deleted_at'; // Validation protected $validationRules = []; protected $validationMessages = []; protected $skipValidation = false; protected $cleanValidationRules = true; // Callbacks protected $allowCallbacks = true; protected $beforeInsert = []; protected $afterInsert = []; protected $beforeUpdate = []; protected $afterUpdate = []; protected $beforeFind = []; protected $afterFind = []; protected $beforeDelete = []; protected $afterDelete = []; }
Create Data Seeder
Open project in terminal and run this spark command.
$ php spark make:seeder Student --suffix
It will create a file with name StudentSeeder.php at /app/Database/Seeds folder.
Open StudentSeeder.php and write this following code into it.
<?php namespace App\Database\Seeds; use App\Models\StudentModel; use CodeIgniter\Database\Seeder; use Faker\Factory; class StudentSeeder extends Seeder { public function run() { $data = []; for ($i = 0; $i < 5; $i++) { $data[] = $this->generateTestStudent(); } $student_obj = new StudentModel(); $student_obj->insertBatch($data); } public function generateTestStudent() { $faker = Factory::create(); return [ "name" => $faker->name(), "term1_marks" => $faker->numberBetween(100, 150), "term2_marks" => $faker->numberBetween(100, 150), "term3_marks" => $faker->numberBetween(100, 150), "term4_marks" => $faker->numberBetween(100, 150), "remarks" => $faker->randomElement([ "Good Work", "Presented smartness in learning", "Amazing learning power" ]), ]; } }
Run Seeder File
Run seeder file by this spark command.
$ php spark db:seed StudentSeeder
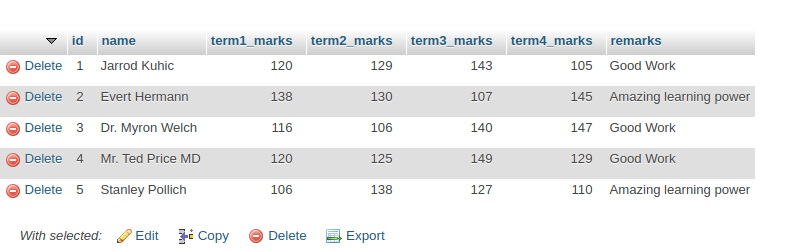
Create Route
Open Routes.php from /app/Config folder. Add this route into it.
//... $routes->get("line-chart", "StudentController::index"); //...
Create Controller
Back to terminal and run this spark command to create application controller.
$ php spark make:controller Student --suffix
It will create StudentController.php file at /app/Controllers folder.
<?php namespace App\Controllers; use App\Controllers\BaseController; use App\Models\StudentModel; class StudentController extends BaseController { public function index() { $student_obj = new StudentModel(); $students = $student_obj->findAll(); $dataPoints = []; foreach ($students as $student) { $dataPoints[] = array( "name" => $student['name'], "data" => [ intval($student['term1_marks']), intval($student['term2_marks']), intval($student['term3_marks']), intval($student['term4_marks']), ], ); } return view("line-chart", [ "data" => json_encode($dataPoints), "terms" => json_encode(array( "Term 1", "Term 2", "Term 3", "Term 4", )), ]); } }
Create View File & Render
Next, create a view file with name line-chart.php at /app/Views folder. Open line-chart.php and write this following code into it.
<!DOCTYPE html> <html lang="en"> <head> <title>Line Chart in CodeIgniter 4 - Online Web Tutor</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <h2 style="text-align: center;">Line Chart in CodeIgniter 4 - Online Web Tutor</h2> <div class="panel panel-primary"> <div class="panel-heading">Line Chart in CodeIgniter 4</div> <div class="panel-body"> <div id="line-chart"></div> </div> </div> </div> <script src="https://code.highcharts.com/highcharts.js"></script> <script src="https://code.highcharts.com/modules/series-label.js"></script> <script src="https://code.highcharts.com/modules/exporting.js"></script> <script src="https://code.highcharts.com/modules/export-data.js"></script> <script src="https://code.highcharts.com/modules/accessibility.js"></script> <script> $(function(){ Highcharts.chart('line-chart', { title: { text: 'Students Term Wise Marks' }, yAxis: { title: { text: 'Number of Marks' } }, xAxis: { categories: <?= $terms ?> }, legend: { layout: 'vertical', align: 'right', verticalAlign: 'middle' }, series: <?= $data ?>, responsive: { rules: [{ condition: { maxWidth: 500 }, chartOptions: { legend: { layout: 'horizontal', align: 'center', verticalAlign: 'bottom' } } }] } }); }); </script> </body> </html>
Application Testing
Open project terminal and start development server via command:
php spark serve
URL: http://localhost:8080/line-chart
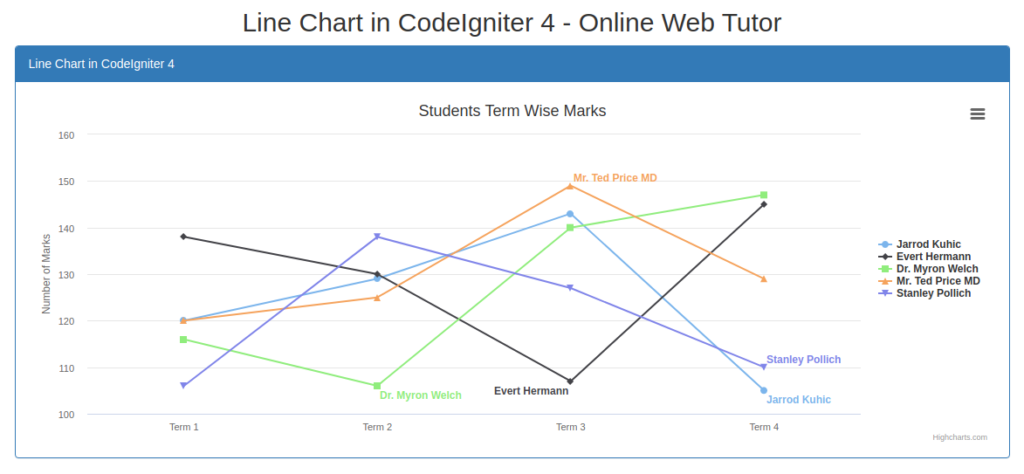
We hope this article helped you to learn Line Chart Integration with CodeIgniter 4 Tutorial in a very detailed way.
To Learn about Bar Chart Integration in CodeIgniter 4 using jQuery Highchart, Click here.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.