Metabox purpose is to provide information to user or allow the user to do some operation. In this tutorial we will see how to render values to form from database table.
In the last tutorial we have saved values to database table.
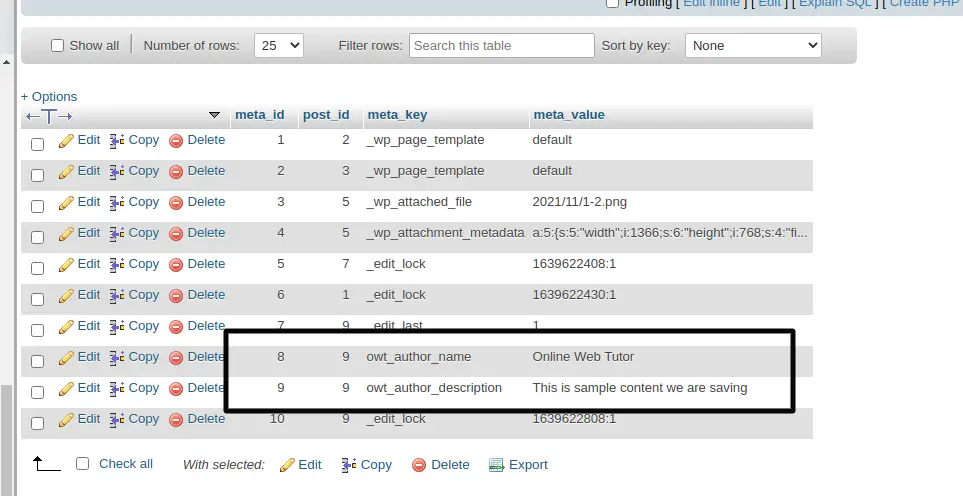
To get values of custom fields we will use get_post_meta() wordpress function.
Syntax
get_post_meta( int $post_id, string $key = '', bool $single = false )
Retrieves a post meta field for the given post ID.
Parameters Detail
$post_id
(int) (Required) Post ID.
$key
(string) (Optional) The meta key to retrieve. By default, returns data for all keys.
Default value: ”
$single
(bool) (Optional) Whether to return a single value. This parameter has no effect if $key
is not specified.
Default value: false
Example
Below code displays input fields within metabox with saved value if any inside wp_postmeta table.
/*
* Custom metabox callback function
*/
function owt_my_display_callback($post)
{
?>
<p>
<input type="text" placeholder="Author name" name="author_name" class="widefat" size="20" value="<?php echo esc_attr( get_post_meta( get_the_ID(), 'owt_author_name', true ) ); ?>">
</p>
<p>
<textarea class="widefat" name="author_desc" placeholder="Add your description"><?php echo esc_attr( get_post_meta( get_the_ID(), 'owt_author_description', true ) ); ?></textarea>
</p>
<?php
}
Explanation
- get_the_ID() is a wordpress function which returns current post ID.
- owt_author_name & owt_author_description are the meta keys saved in wp_postmeta table with values.
- true value at last is for return single value by get_post_meta() function
- The whole line means getting single value from wp_postmeta table on the basis of meta key and post ID.
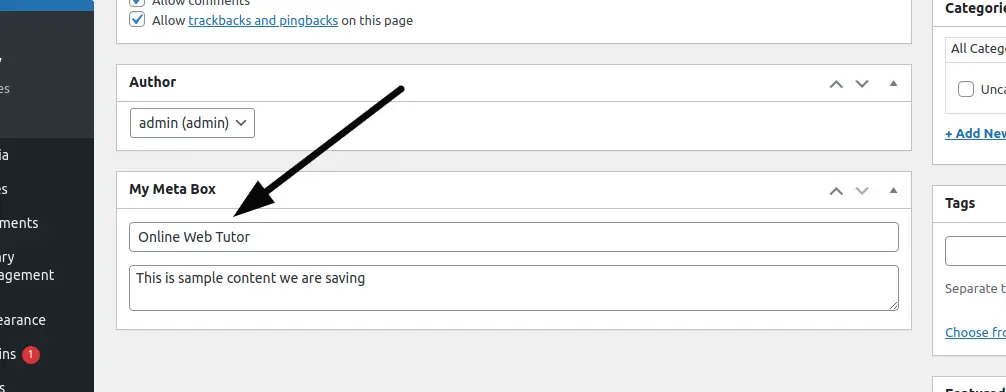
Complete Code
Here is the complete which add a metabox with two input fields into Create Post / Edit Post page. It saves value along with post data into wp_postmeta table.
/**
* Register meta box(es).
*/
function owt_register_post_meta_box()
{
add_meta_box('meta-box-id', __('My Meta Box', 'textdomain'), 'owt_my_display_callback', 'post');
}
add_action('add_meta_boxes', 'owt_register_post_meta_box');
/*
* Custom metabox callback function
*/
function owt_my_display_callback($post)
{
?>
<p>
<input type="text" placeholder="Author name" name="author_name" class="widefat" size="20" value="<?php echo esc_attr( get_post_meta( get_the_ID(), 'owt_author_name', true ) ); ?>">
</p>
<p>
<textarea class="widefat" name="author_desc" placeholder="Add your description"><?php echo esc_attr( get_post_meta( get_the_ID(), 'owt_author_description', true ) ); ?></textarea>
</p>
<?php
}
/**
* Save metabox inputs
*/
function owt_save_custom_meta_box_content($post_id)
{
$author_name = $_POST['author_name'];
$author_desc = $_POST['author_desc'];
update_post_meta($post_id, 'owt_author_name', $author_name);
update_post_meta($post_id, 'owt_author_description', $author_desc);
}
add_action('save_post', 'owt_save_custom_meta_box_content');
Once values saved, render back saved value to metabox inputs.
You can add this code into your custom plugin code or add inside activated theme functions.php file.