Metabox purpose is to provide information to user or allow the user to do some operation. In this tutorial we will see how to save form elements values to wordpress database table.
save_post action hook available to save post data into table. So when we save metabox values then by using this action hook we can save it our values as well.
When we save metabox values either a defined metabox of wordpress or it will be a custom metabox it saves each value to wp_postmeta table with post ID.
We are considering the same metabox what we have created in the last tutorial. Metabox with two input fields like Author name & Description. We will save these values.
Example
/**
* Save textbox content
*/
function owt_save_custom_meta_box_content($post_id)
{
$author_name = $_POST['author_name'];
$author_desc = $_POST['author_desc'];
update_post_meta($post_id, 'owt_author_name', $author_name);
update_post_meta($post_id, 'owt_author_description', $author_desc);
}
add_action('save_post', 'owt_save_custom_meta_box_content');
You need to add this code script to save your custom metabox value. For two fields we have two $_POST data. According to your data inputs you can add more.
We have used a action hook save_post which calls when we save post data. Also we have used update_post_meta wordpress function to save / update input values to wp_postmeta table.
update_post_meta function takes post_id, key and value to be saved.
Here, we have used owt_author_name & owt_author_description keys to save values to table.
Complete Code
Here is the complete which add a metabox with two input fields into Create Post / Edit Post page. Also it saves value along with post data into wp_postmeta table.
You can add this code into your custom plugin code or add inside activated theme functions.php file.
/**
* Register meta box(es).
*/
function owt_register_post_meta_box()
{
add_meta_box('meta-box-id', __('My Meta Box', 'textdomain'), 'owt_my_display_callback', 'post');
}
add_action('add_meta_boxes', 'owt_register_post_meta_box');
/*
* Custom metabox callback function
*/
function owt_my_display_callback($post)
{
?>
<p>
<input type="text" placeholder="Author name" name="author_name" class="widefat" size="20">
</p>
<p>
<textarea class="widefat" name="author_desc" placeholder="Add your description"></textarea>
</p>
<?php
}
/**
* Save metabox inputs
*/
function owt_save_custom_meta_box_content($post_id)
{
$author_name = $_POST['author_name'];
$author_desc = $_POST['author_desc'];
update_post_meta($post_id, 'owt_author_name', $author_name);
update_post_meta($post_id, 'owt_author_description', $author_desc);
}
add_action('save_post', 'owt_save_custom_meta_box_content');
Provide Input Values
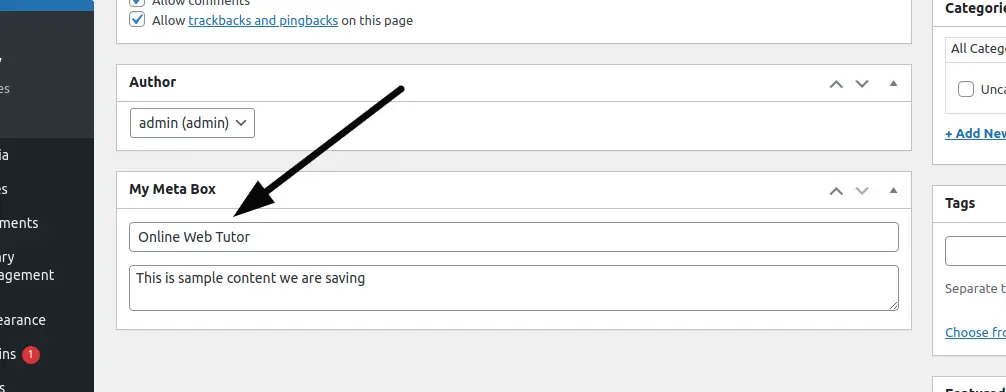
“wp_postmeta” Database Table
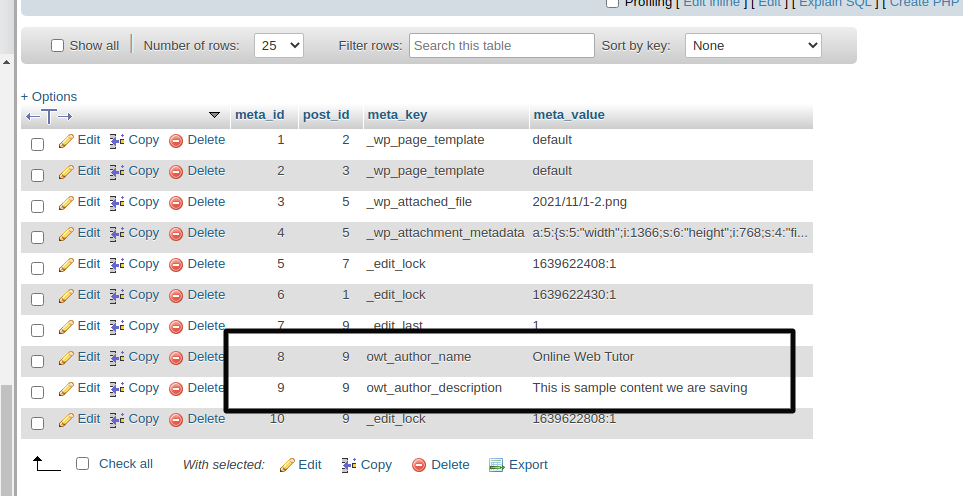
In the next tutorial we will see how to render saved value to custom fields of metabox.