This is very common to every web application i.e Passing parameters to URLs. Also called as parameterized routing of application.
Inside this article we will see the concept of Parameterized Routing in CodeIgniter 4. Already we have articles over Group routes in CodeIgniter 4 and Routing Tutorial in CodeIgniter 4. Please check.
We will cover these following topics –
- URL Placeholders & With Parameters
- URL with Query String
- Optional parameter in URL
Learn More –
- Codeigniter 4 Multi Auth User Role Wise Login
- CodeIgniter 4 Multiple Databases And Connection Groups
- CodeIgniter 4 Pagination Service or Library
- CodeIgniter 4 PDF Generate Tutorial
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
URL Placeholders & With Parameters
In CodeIgniter 4 application, there are several types of placeholder available to pass value to URL. Parameters as like of type Integer value, String value, Hashed value, Alpna numeric etc.
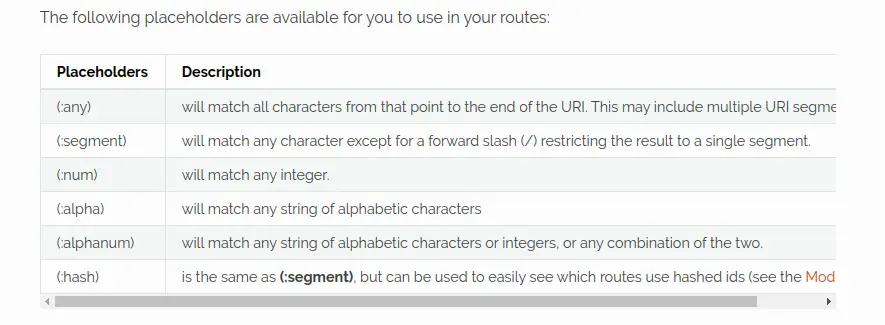
Let’s take an example to see How can we pass and access value to controller?
Open Routes.php file from /app/Config folder.
//... $routes->get("my-route/(:num)", "MyController::myRoute/$1"); $routes->get("sample-route/(:any)/(:num)", "MyController::sampleRoute/$1/$2"); //...
Here, we have configured few routes.
- First route will take a numeric value into URL as (:num) placeholder. We can pass any value there is no restriction with this placeholder i.e (:num) means only integer value. But I suggest, while developing any kind of application all things should be meaningful. That’s why use (:num) only when pass numeric value into URLs.
- $1 in first route – Passing url value to myRoute() method.
- Second route will take 2 values – one is any type means integer, string etc and other is for numeric value.
- $1 is for (:any) value and $2 is for numeric value. These two values $1 & $2 we are passing inside sampleRoute() method.
Example URLs
- /my-route/12
- /my-route/45 … etc
- /sample-route/hi/40
- /sample-route/256/120
Access URL values to Controller
<?php namespace App\Controllers; use App\Controllers\BaseController; class MyController extends BaseController { public function myRoute($id) { // .. code here } public function sampleRoute($key, $id) { // .. code here } }
- myRoute($id) – Get (:num) value to method. This $id variable contains the URL value.
- sampleRoute($key, $id) – Get (:any) & (:num) value. These two variables contains the URL value.
URL with Query String
For passing query string value, we don’t need to do anything with the route configuration.
Let’s add a simple route.
Open Routes.php file from /app/Config folder.
//... $routes->get("my-route", "MyController::myRoute"); //...
Example URLs
- /my-route?name=sanjay&role=auth
- /my-route?message=success&error=0
Access URL values to Controller
<?php namespace App\Controllers; use App\Controllers\BaseController; class MyController extends BaseController { public function myRoute() { $all_query_values = $this->request->getVar(); // It will return an array of all values // get query variable - name $name = $this->request->getVar("name"); // get query variable - role $role = $this->request->getVar("role"); } }
- $this->request->getVar() – It returns all query variables with values into an array format.
- $this->request->getVar(“name”); – It will return the single query variable value i.e name
Optional parameter in URL
In CodeIgniter 4, there is no such type documentation available which handles the situation for optional parameters.
But we can do this way to implement the concept of optional parameter into URLs.
Open Routes.php file from /app/Config folder.
//... $routes->get("my-route(:any)", "MyController::myRoute$1"); //...
We need to use (:any) placeholder, keep in mind else any other placeholders like (:num), (:alpha) returns 404 Page not found error.
Example URLs
- /my-route/some-value
- /my-route
Access URL values to Controller
<?php namespace App\Controllers; use App\Controllers\BaseController; class MyController extends BaseController { public function myRoute($value = null) { // $value will be available if we pass into URL // Else no value in it. // Same route we can use for passing parameter or with no value. } }
We hope this article helped you to learn Parametrized Routes in CodeIgniter 4 Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.