Every application nowadays must have an authenticated system. In most cases application have several user roles and their level of access. For example user roles like editor, author, admin etc. We are using CodeIgniter 4 in this article. So accordingly we are going to create Codeigniter 4 Multi Auth User Role.
Easy & simple step by step guide to implement in CodeIgniter 4. The modules we are going to cover to develop this system as – Filters, Controller, Routes, Database, Model, View, Custom Rule.
Learn for Step by step CodeIgniter 4 Login & Registration Click here.
Learn More –
- CodeIgniter 4 Send Push Notification to IOS Using Firebase
- CodeIgniter 4 Server Side DataTable Tutorial
- CodeIgniter 4 Server Side DataTable Using SSP Library
- CodeIgniter 4 Session Library or Service
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Now, let’s configure database and application connectivity.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Create Database
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
We will use MySQL command to create database. Run this command into Sql tab of PhpMyAdmin.
CREATE DATABASE codeigniter4_app;
Successfully, we have created a database.
Create Database Table
Next, we need a table. That table will be responsible to store data.
Let’s create table with some columns.
CREATE TABLEtbl_users
(id
int(11) NOT NULL AUTO_INCREMENT,name
varchar(120) DEFAULT NULL,phone_no
varchar(120) DEFAULT NULL,role
enum('admin','editor') NOT NULL,password
varchar(120) DEFAULT NULL,created_at
timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP, PRIMARY KEY (id
) ) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Successfully, we have created a table.
Let’s connect with the application.
Database Connection
Open .env file from project root.
Search for DATABASE. You should see the connection environment variables into it. Put your updated details of database connection string values.
#-------------------------------------------------------------------- # DATABASE #-------------------------------------------------------------------- database.default.hostname = localhost database.default.database = codeigniter4_app database.default.username = admin database.default.password = admin database.default.DBDriver = MySQLi database.default.DBPrefix = database.default.port = 3306
Now, database successfully connected with the application.
Create Model
Model is the face of application with the database. We need a User Model which will do some basic model configuration.
Models are created at /app/Models. We are going to create UserModel.php at this location.
$ php spark make:model User --suffix
<?php namespace App\Models; use CodeIgniter\Model; class UserModel extends Model { protected $DBGroup = 'default'; protected $table = 'tbl_users'; protected $primaryKey = 'id'; protected $useAutoIncrement = true; protected $insertID = 0; protected $returnType = 'array'; protected $useSoftDelete = false; protected $protectFields = true; protected $allowedFields = [ "name", "email", "phone_no", "password", "role" ]; // Dates protected $useTimestamps = false; protected $dateFormat = 'datetime'; protected $createdField = 'created_at'; protected $updatedField = 'updated_at'; protected $deletedField = 'deleted_at'; // Validation protected $validationRules = []; protected $validationMessages = []; protected $skipValidation = false; protected $cleanValidationRules = true; // Callbacks protected $allowCallbacks = true; protected $beforeInsert = []; protected $afterInsert = []; protected $beforeUpdate = []; protected $afterUpdate = []; protected $beforeFind = []; protected $afterFind = []; protected $beforeDelete = []; protected $afterDelete = []; }
- $table – Passing table name where we will store user data
- $allowedFields – User fields for mass assignment.
Create Data Seeder – User Roles
Open project into terminal run this spark command.
$ php spark make:seeder User --suffix
It will create a file with name UserSeeder.php at /app/Database/Seeds folder.
Open file and write this code.
<?php namespace App\Database\Seeds; use CodeIgniter\Database\Seeder; use App\Models\UserModel; class UserSeeder extends Seeder { public function run() { $user_object = new UserModel(); $user_object->insertBatch([ [ "name" => "Rahul Sharma", "email" => "rahul_sharma@mail.com", "phone_no" => "7899654125", "role" => "admin", "password" => password_hash("12345678", PASSWORD_DEFAULT) ], [ "name" => "Prabhat Pandey", "email" => "prabhat@mail.com", "phone_no" => "8888888888", "role" => "editor", "password" => password_hash("12345678", PASSWORD_DEFAULT) ] ]); } }
Run Seeder
Back to terminal and run this spark command to seed the test user into database table.
$ php spark db:seed UserSeeder

Create Routes
To configure application routes, we need to open up the file /app/Config/Routes.php. This is the main routes config file where we will do all routes of application.
Inside this we will have login, dashboard routes role wise etc.
//... $routes->match(['get', 'post'], 'login', 'UserController::login', ["filter" => "noauth"]); // Admin routes $routes->group("admin", ["filter" => "auth"], function ($routes) { $routes->get("/", "AdminController::index"); }); // Editor routes $routes->group("editor", ["filter" => "auth"], function ($routes) { $routes->get("/", "EditorController::index"); }); $routes->get('logout', 'UserController::logout'); //...
Inside these routes, we are using filters. Filters are the sections from where we can check every request to application and do action. We will create two filters – auth & noauth.
Configure Filters
Filters in CodeIgniter 4 are just like guards. They detect request and process what we have created it for. So here, we will create and configure two different filters which we use in application.
To create filters, we will use the folder inside app directory i.e /app/Filters.
$ php spark make:filter Auth
It will create file Auth.php inside /app/Filters folder.
<?php namespace App\Filters; use CodeIgniter\Filters\FilterInterface; use CodeIgniter\HTTP\RequestInterface; use CodeIgniter\HTTP\ResponseInterface; class Auth implements FilterInterface { public function before(RequestInterface $request, $arguments = null) { if (!session()->get('isLoggedIn')) { return redirect()->to(site_url('login')); } } public function after(RequestInterface $request, ResponseInterface $response, $arguments = null) { // Do something here } }
$ php spark make:filter Noauth
It will create Noauth.php inside /app/Filters folder.
<?php namespace App\Filters; use CodeIgniter\Filters\FilterInterface; use CodeIgniter\HTTP\RequestInterface; use CodeIgniter\HTTP\ResponseInterface; class Noauth implements FilterInterface { public function before(RequestInterface $request, $arguments = null) { if (session()->get('isLoggedIn')) { if (session()->get('role') == "admin") { return redirect()->to(base_url('admin')); } if (session()->get('role') == "editor") { return redirect()->to(base_url('editor')); } } } public function after(RequestInterface $request, ResponseInterface $response, $arguments = null) { // Do something here } }
Successfully, we have created filters. Next, we need to configure into application so that we will able to use.
To register these filters.
Open up the file – /app/Config/Filters.php
# Add to Header use App\Filters\Auth; use App\Filters\Noauth; # Update this public $aliases = [ // .. others "auth" => Auth::class, "noauth" => Noauth::class, ];
As, you can see we have added our filter config lines
'auth' => \App\Filters\Auth::class,
'noauth' => \App\Filters\Noauth::class,
Register Custom Rule in Application – validateUser
When we do controller’s code, then at login method we will use a custom rule which checks user is valid or invalid at the time of login. We can actually do this task in several ways, but I have preferred this for neater & cleaner code.
To store custom validation rules file, Validation folder may or may not be present in /app directory.
Let’s create a file with the name of Userrules.php in /app/Validation.
$ php spark make:validation Userrules
Source code of /app/Validation/Userrules.php
<?php namespace App\Validation; use App\Models\UserModel; class Userrules { public function validateUser(string $str, string $fields, array $data) { $model = new UserModel(); $user = $model->where('email', $data['email']) ->first(); if (!$user) { return false; } return password_verify($data['password'], $user['password']); } }
Next, we need to need to register this custom rule to application for use.
Open up the file /app/Config/Validation.php
# Add to Header use App\Validation\Userrules; public $ruleSets = [ // .. other rules Userrules::class, // here we have registered ];
Application Controller Settings
Controller is the functional file. Firstly let’s load some helpers at Parent Controller i.e BaseController.php. This file is in /app/Controllers folder.
Search helpers in BaseController and load “url” into helpers.
protected $helpers = [‘url’];
After loading this url helper, we will able to use site_url() and base_url() in Controllers & Views else we should have some error.
We will create application controller at /app/Controllers. Let’s create UserController.php, AdminController.php (For admin user role) & EditorController.php (for editor user role) inside the given folder.
$ php spark make:controller User --suffix
$ php spark make:controller Admin --suffix
$ php spark make:controller Editor --suffix
Write the following code into /app/Controllers/UserController.php
<?php namespace App\Controllers; use App\Controllers\BaseController; use App\Models\UserModel; class UserController extends BaseController { public function login() { $data = []; if ($this->request->getMethod() == 'post') { $rules = [ 'email' => 'required|min_length[6]|max_length[50]|valid_email', 'password' => 'required|min_length[8]|max_length[255]|validateUser[email,password]', ]; $errors = [ 'password' => [ 'validateUser' => "Email or Password didn't match", ], ]; if (!$this->validate($rules, $errors)) { return view('login', [ "validation" => $this->validator, ]); } else { $model = new UserModel(); $user = $model->where('email', $this->request->getVar('email')) ->first(); // Stroing session values $this->setUserSession($user); // Redirecting to dashboard after login if($user['role'] == "admin"){ return redirect()->to(base_url('admin')); }elseif($user['role'] == "editor"){ return redirect()->to(base_url('editor')); } } } return view('login'); } private function setUserSession($user) { $data = [ 'id' => $user['id'], 'name' => $user['name'], 'phone_no' => $user['phone_no'], 'email' => $user['email'], 'isLoggedIn' => true, "role" => $user['role'], ]; session()->set($data); return true; } public function logout() { session()->destroy(); return redirect()->to('login'); } }
- login() Method which handles both GET & POST request type. Inside this user login function will process with the help of email and password. Inside this method we are using Form Validation service, session, model. After successful login we are setting user data into session by using this $this->setUserSession($user);
- setUserSession($user) This is a private method what we have created. Inside this method simply we are storing user data into session. These session data we can use either in filters and/or at the dashboard page.
- logout() Method used to destroy all user data from session and do user logged out.
Open AdminController.php file and write this following code into it.
Code for /app/Controllers/AdminController.php
<?php namespace App\Controllers; use App\Controllers\BaseController; class AdminController extends BaseController { public function __construct() { if (session()->get('role') != "admin") { echo 'Access denied'; exit; } } public function index() { return view("admin/dashboard"); } }
Open EditorController.php file and write this following code into it.
Code for /app/Controllers/EditorController.php
<?php namespace App\Controllers; use App\Controllers\BaseController; class EditorController extends BaseController { public function __construct() { if (session()->get('role') != "editor") { echo 'Access denied'; exit; } } public function index() { return view("editor/dashboard"); } }
View File Setup in Application
View files generally created inside /app/Views. We need view files for login, admin dashboard, editor dashboard etc.
Let’s create those step by step.
Create Parent Template
Create a folder layouts inside /app/Views. Inside layouts create a file app.php. This is be parent layout.
Open file /app/Views/layouts/app.php and write this code.
<!DOCTYPE html> <html lang="en"> <head> <title>CodeIgniter 4 Multi Auth Tutorial - Online Web Tutor</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> </head> <body> <?= $this->renderSection("body") ?> </body> </html>
We will extend this template file to every view file and dynamically attach page views in it’s body.
Login View File
Create a file with name login.php at /app/Views folder.
Code of /app/Views/login.php
<?= $this->extend("layouts/app") ?> <?= $this->section("body") ?> <div class="container" style="margin-top:20px;"> <div class="row"> <div class="col-sm-6"> <div class="panel panel-primary"> <div class="panel-heading">Login</div> <div class="panel-body"> <?php if (isset($validation)) : ?> <div class="col-12"> <div class="alert alert-danger" role="alert"> <?= $validation->listErrors() ?> </div> </div> <?php endif; ?> <form class="" action="<?= base_url('login') ?>" method="post"> <div class="form-group"> <label for="email">Email</label> <input type="email" class="form-control" name="email" id="email"> </div> <div class="form-group"> <label for="password">Password</label> <input type="password" class="form-control" name="password" id="password"> </div> <button type="submit" class="btn btn-success">Submit</button> </form> </div> </div> </div> </div> </div> <?= $this->endSection() ?>
Admin Dashboard View File
Create a folder with name admin inside /app/Views. This folder is to store all admin view pages.
Create dashboard.php inside /app/Views/admin
Source code for dashboard.php
<?= $this->extend("layouts/app") ?> <?= $this->section("body") ?> <div class="container" style="margin-top:20px;"> <div class="row"> <div class="col-sm-6"> <div class="panel panel-primary"> <div class="panel-heading">Admin Dashboard</div> <div class="panel-body"> <h1>Hello, <?= session()->get('name') ?></h1> <h3><a href="<?= site_url('logout') ?>">Logout</a></h3> </div> </div> </div> </div> </div> <?= $this->endSection() ?>
Editor Dashboard View File
Create a folder with name editor inside /app/Views. This folder is to store all editor view pages.
Create dashboard.php inside /app/Views/editor
Source code for dashboard.php
<?= $this->extend("layouts/app") ?> <?= $this->section("body") ?> <div class="container" style="margin-top:20px;"> <div class="row"> <div class="col-sm-6"> <div class="panel panel-primary"> <div class="panel-heading">Editor Dashboard</div> <div class="panel-body"> <h1>Hello, <?= session()->get('name') ?></h1> <h3><a href="<?= site_url('logout') ?>">Logout</a></h3> </div> </div> </div> </div> </div> <?= $this->endSection() ?>
Application Testing
Open project terminal and start development server via command:
php spark serve
Login URL: http://localhost:8080/login
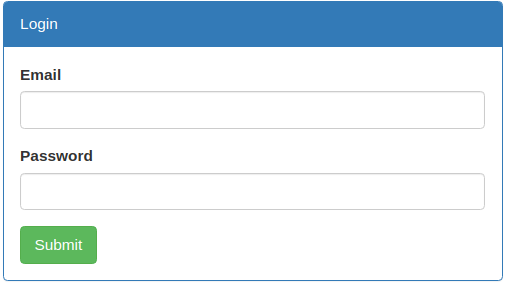
Admin Dashboard URL: http://localhost:8080/admin
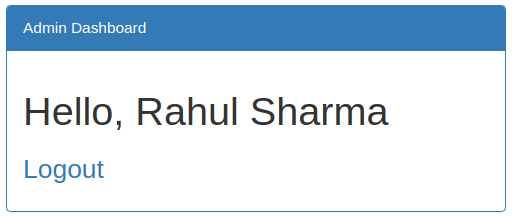
When we have logged in via Admin details, so when we try to open the url of editor like http://localhost:8080/editor
Access deined
Editor Dashboard URL: http://localhost:8080/editor
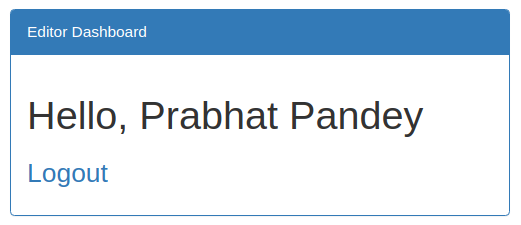
When we have logged in via Editor details, so when we try to open the url of editor like http://localhost:8080/admin
Access denied
We hope this article helped you for Codeigniter 4 Multi Auth User Role Wise Login in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.
Really great post. Thanks for it… Can you also do “role based access control in codeigniter 4” … love what you are doing… keep up the good work…
Thanks
I am not able to access admin page.
this is the url http://localhost:8080/admin.
This page isn’t working
localhost redirected you too many times.
Try clearing your cookies.
ERR_TOO_MANY_REDIRECTS
Let me check with a new setup and if exists then I will update this article asap.