While doing any operation in any application we want some confirmation alerts like for success, warning, error. Why we need these. It’s because it gives a more readable power to end users to understand what’s happening.
Inside this article we will implement or add bootstrap growl jquery notification to CodeIgniter 4 application. This is not CodeIgniter 4 application specific. You can use in any application of PHP.
To learn about Sweetalert2 jQuery notification plugin to CodeIgniter 4, Click here.
This article will cover about using Bootstrap growl jquery plugin for message notifications to end users. Generally notifications helps user to understand about the steps what they initiated, what’s happening, about next process, etc.
Learn More –
- CodeIgniter 4 Send Push Notification to IOS Using Firebase
- CodeIgniter 4 Server Side DataTable Tutorial
- CodeIgniter 4 Server Side DataTable Using SSP Library
- CodeIgniter 4 Session Library or Service
Step by step we will see their needed plugin files and implementation.
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Required Plugin Files
Bootstrap Growl jQuery plugin file
JS File
https://cdnjs.cloudflare.com/ajax/libs/bootstrap-growl/1.0.0/jquery.bootstrap-growl.min.js
Bootstrap CSS File
https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css
We need a plugin JS file and a CSS file (It’s same as bootstrap css what we need) for its layout styling. After adding these plugin files, we are allowed to use it’s method like –
$.bootstrapGrowl("<Message Here>", {})
Implementing Bootstrap Growl jQuery Plugin
Let’s create a simple application where we will see the step by step implementation of bootstrap growl jQuery plugin files.
Create Route
Routes can be configured into Routes.php which is at /app/Config folder.
//... $routes->get('notification', 'MessageController::showGrowlMessages'); //...
Create Controller
$ php spark make:controller Message --suffix
Open MessageController.php from /app/Controllers
<?php namespace App\Controllers; use App\Controllers\BaseController; class MessageController extends BaseController { public function showGrowlMessages() { // Flash messages settings session()->setFlashdata("success", "This is success message"); session()->setFlashdata("warning", "This is warning message"); session()->setFlashdata("info", "This is information message"); session()->setFlashdata("error", "This is error message"); return view("growl-notification"); } }
Create Blade Template File
Create a file with name growl-notification.php at /app/Views folder. Open file and write this code into it.
<script src="https://code.jquery.com/jquery-3.5.1.min.js"></script> <link href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" rel="stylesheet"> <script src="https://cdnjs.cloudflare.com/ajax/libs/bootstrap-growl/1.0.0/jquery.bootstrap-growl.min.js"></script> <script> $(function(){ <?php if(session()->has("success")) { ?> $.bootstrapGrowl('<?= session("success") ?>',{ type: 'success', delay: 4000, }); <?php } ?> <?php if(session()->has("info")) { ?> $.bootstrapGrowl('<?= session("info") ?>',{ type: 'info', delay: 4000, }); <?php } ?> <?php if(session()->has("error")) { ?> $.bootstrapGrowl('<?= session("error") ?>',{ type: 'danger', delay: 4000, }); <?php } ?> <?php if(session()->has("warning")) { ?> $.bootstrapGrowl('<?= session("warning") ?>',{ type: 'warning', delay: 4000, }); <?php } ?> }); </script>
Right now we are displaying these notification messages without any operations like insert, update or anything but you can use it for your application notifications.
Application Testing
Open project terminal and start development server via command:
php spark serve
URL – http://localhost:8080/notification
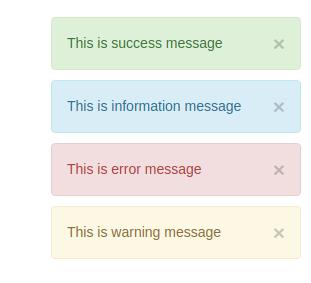
Plugin Error If not Linked Properly
If suppose we missed the plugin files to attach or let’s say not linked properly, then at console tab we will get something.
$.bootstrapGrowl is not a function
We hope this article helped you to learn about i.e Bootstrap Growl jQuery Notification to CodeIgniter 4 Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.