Inside this article we will see the concept i.e CodeIgniter 4 How To Work with Server Side DataTable. Article contains the classified information about How to Implement and Use DataTables in CodeIgniter 4.
Here, we will use SSP(server side processing) a third party Library in CodeIgniter 4 to list data from server and cover the topic i.e CodeIgniter 4 How To Work with Server Side DataTable.
CodeIgniter 4 itself has a service of pagination for server side data i.e Pager service. But we will not use this service for now.
Learn More –
- Server Side Datatable in CodeIgniter 4
- How to Install CodeIgniter 4 in Ubuntu
- How To Load Database in CodeIgniter 4 Custom Library
- How To Read CSV File in CodeIgniter 4 Tutorial
Let’s get started.
What is SSP Library?
SSP stands for Server Side Processing. It is a class, which basically a Datatable Library. There are some methods & configuration we need to do and then we can easily use this library to list server side data.
This is syntax of SSP class to call simple method which brings all data and converting to json encoded format.
echo json_encode( \SSP::simple($_GET, $dbDetails, $table, $primaryKey, $columns) );
Let’s see step by step SSP library integration to CodeIgniter 4.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Create Database
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
We will use MySQL command to create database. Run this command into Sql tab of PhpMyAdmin.
CREATE DATABASE codeigniter4_app;
Successfully, we have created a database.
Create Database Table
Next, we need a table. That table will be responsible to store data.
Let’s create table with some columns.
CREATE TABLE `tbl_members` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(120) DEFAULT NULL,
`email` varchar(120) DEFAULT NULL,
`mobile` varchar(45) DEFAULT NULL,
`designation` varchar(50) DEFAULT NULL,
`gender` enum('male','female') NOT NULL,
`status` int(11) NOT NULL DEFAULT '1',
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=latin1;
Successfully, we have created a table.
Database Connection
Open .env file from project root.
Search for DATABASE. You should see the connection environment variables into it. Put your updated details of database connection string values.
#-------------------------------------------------------------------- # DATABASE #-------------------------------------------------------------------- database.default.hostname = localhost database.default.database = codeigniter4_app database.default.username = admin database.default.password = admin database.default.DBDriver = MySQLi database.default.DBPrefix = database.default.port = 3306
Now, database successfully connected with the application.
Create Data Seeder
Next, we need dummy data set to demonstrate this article. If you have real time data, it will be good else continue with this Fake data.
To generate fake data – Need to create a seeder file and then will use Faker Library.
By default Faker library is available in CodeIgniter 4.
To create seeder file, back to terminal and run this spark command.
$ php spark make:seeder Member --suffix
It will create a file MemberSeeder.php into /app/Database/Seeds folder.
Open MemberSeeder.php file and write this complete code into it.
<?php namespace App\Database\Seeds; use CodeIgniter\Database\Seeder; use Faker\Factory; class MemberSeeder extends Seeder { public function run() { $data = []; for ($i = 0; $i < 50; $i++) { $data[] = $this->generateTestMember(); } $this->db->table("tbl_members")->insertBatch($data); } public function generateTestMember() { $faker = Factory::create(); return [ "name" => $faker->name(), "email" => $faker->email, "mobile" => $faker->phoneNumber, "designation" => $faker->randomElement([ "Wordpress Developer", "IOS Developer", "HR", "Designer", "PHP Developer", "Project Manager", "SEO", "Android Developer" ]), "gender" => $faker->randomElement([ "male","female","other", ]), "status" => $faker->randomElement([1, 0]), ]; } }
Run Seeder File
To seed dummy data, we need to run the above created seeder file. Run this spark command to terminal.
$ php spark db:seed MemberSeeder
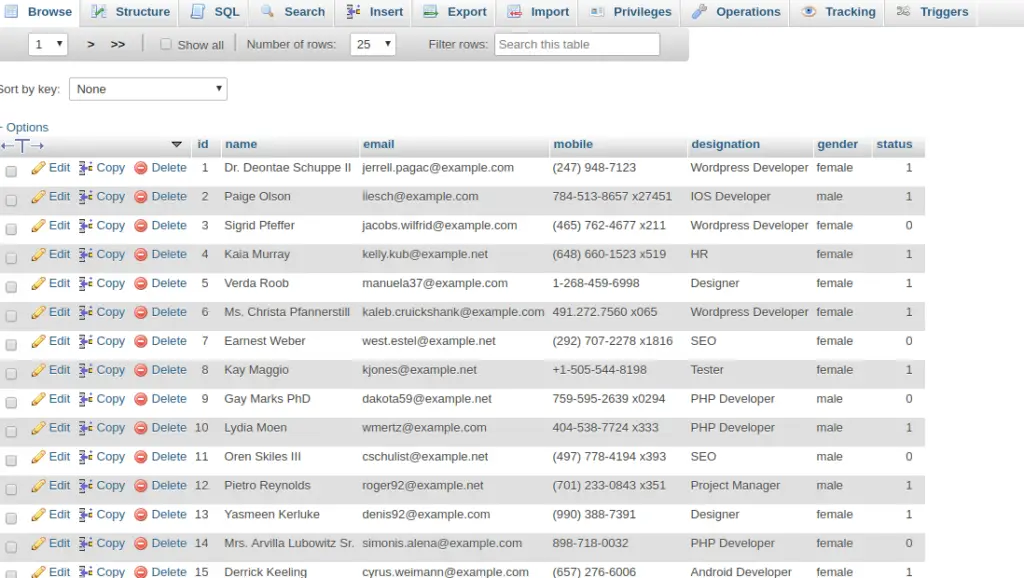
Add Routes
Open Routes.php from /app/Config folder. Add these routes into it.
//... $routes->get('data-layout', 'Site::server_side_table_ssp_listing_layout'); $routes->get('list-data', 'Site::list_data_using_ssp_ajax'); //...
First route is to render the layout of DataTable and second one is for render data into it.
Create Controller
Before creating application Controller we need SSP Library. This library helps to send data from server to AJAX request as a response. Ajax request we will create from View file.
When you download this zipped folder, unzip that. Copy the file and place it inside /app/ThirdParty/ssp.class.php. This class file we have placed at ThirdParty folder.
Next, create a controller and add few methods into it.
- Open the DataTable Layout (Method 1)
- Process Ajax Request (Method 2)
$ php spark make:controller Site
It will creates Site.php file at /app/Controllers folder.
Open Site.php and write this complete code into it.
<?php namespace App\Controllers; use App\Controllers\BaseController; class Site extends BaseController { public function __construct() { require_once APPPATH . 'ThirdParty/ssp.class.php'; $this->db = db_connect(); } public function server_side_table_ssp_listing_layout() { return view("data"); } public function list_data_using_ssp_ajax() { // this is database details $dbDetails = array( "host" => $this->db->hostname, "user" => $this->db->username, "pass" => $this->db->password, "db" => $this->db->database, ); $table = "tbl_members"; //primary key $primaryKey = "id"; $columns = array( array( "db" => "id", "dt" => 0, ), array( "db" => "name", "dt" => 1, ), array( "db" => "email", "dt" => 2, ), array( "db" => "mobile", "dt" => 3, ), array( "db" => "designation", "dt" => 4, ), array( "db" => "gender", "dt" => 5, "formatter" => function ($value, $row) { return ucfirst($value); }, ), array( "db" => "status", "dt" => 6, "formatter" => function ($value, $row) { return $value == 1 ? "Active" : "Inactive"; }, ) ); echo json_encode( \SSP::simple($_GET, $dbDetails, $table, $primaryKey, $columns) ); } }
- require_once APPPATH . ‘ThirdParty/ssp.class.php’; This is to include SSP Library file from ThirdParty folder. APPPATH is CodeIgniter 4 Constant.
- $this->db = db_connect(); – Creating database instance
- $this->db->hostname – It returns the hostname of database.
- $this->db->username – Username of Database PhpMyAdmin
- $columns = array() Inside this array we need to pass the columns of table from where we are going to fetch data.
- \SSP::simple($_GET, $dbDetails, $table, $primaryKey, $columns) Passing all the details to simple method, it fetches all data and converts into json encoded format. This encoded data then sent back to client side.
Create View Layout File
We will create a view file. This view file is the layout of DataTable for end users. We will use some bootstrap files, datatable files to make it in some proper layout.
View file we will create inside /app/Views folder. For bootstrap files and others you need to download the libraries from here.
When you click into it, it will download a zipped folder. Simply you need to unzip that, copy assets folder and place into /public folder of CodeIgniter 4 Setup.
Create data.php file inside /app/Views folder.
Open file and write this complete code into it.
<html> <head> <link rel="stylesheet" href="<?php echo base_url() ?>/public/assets/css/bootstrap.min.css"/> <link rel="stylesheet" href="<?php echo base_url() ?>/public/assets/css/jquery.dataTables.min.css"/> <link rel="stylesheet" href="<?php echo base_url() ?>/public/assets/css/font-awesome.min.css"/> </head> <body> <div class="container" style="margin-top:40px;"> <divc class="row"> <div class="panel panel-primary"> <div class="panel-heading">Server Side Listing (Using SSP Library)</div> <div class="panel-body"> <table id="server-side-table-ssp" class="display" style="width:100%"> <thead> <tr> <th>User ID</th> <th>Name</th> <th>Email</th> <th>Mobile</th> <th>Designation</th> <th>Gender</th> <th>Status</th> </tr> </thead> </table> </div> </div> </div> </div> <script src="<?php echo base_url() ?>/public/assets/js/jquery.min.js"></script> <script src="<?php echo base_url() ?>/public/assets/js/bootstrap.min.js"></script> <script src="<?php echo base_url() ?>/public/assets/js/jquery.dataTables.min.js"></script> <script src="https://cdn.datatables.net/buttons/1.5.6/js/dataTables.buttons.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/jszip/3.1.3/jszip.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/pdfmake.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/pdfmake/0.1.53/vfs_fonts.js"></script> <script src="https://cdn.datatables.net/buttons/1.5.6/js/buttons.html5.min.js"></script> <script src="https://cdn.datatables.net/buttons/1.5.6/js/buttons.print.min.js"></script> <script> $(function() { if ($("#server-side-table-ssp").length > 0) { $("#server-side-table-ssp").DataTable({ "processing": true, "serverSide": true, "ajax": "<?php echo site_url('list-data') ?>", dom: 'Bfrtip', buttons: [{ extend: "copy", exportOptions: { columns: [] } }, { extend: "excel", title: "client_side_data" }, { extend: "csv", title: "client_side_table_data" }, { extend: "pdf", exportOptions: { columns: ":visible" } }, 'print' ] }); } }); </script> </body> </html>
This is all about a simple HTML code with link tag, script tag and a Table. Inside this file we have used base_url() helper function which brings the application url which we have configured above.
- if ($(“#server-side-table-ssp”).length > 0) {} This is checking the existence of specified ID into page. If length will greater than 0 then goes inside if block.
- $(“#server-side-table-ssp”).DataTable({}); This code is for attaching DataTable with table ID.
- “ajax”: “” Inside this ajax property, we have added route to get data via AJAX hit.
- buttons: [] This is all about export buttons array. We have specified cop to clipboard, PDF, Print etc button to DataTable.
Application Testing
Open project terminal and start development server via command:
php spark serve
URL – http://localhost:8080/data-layout
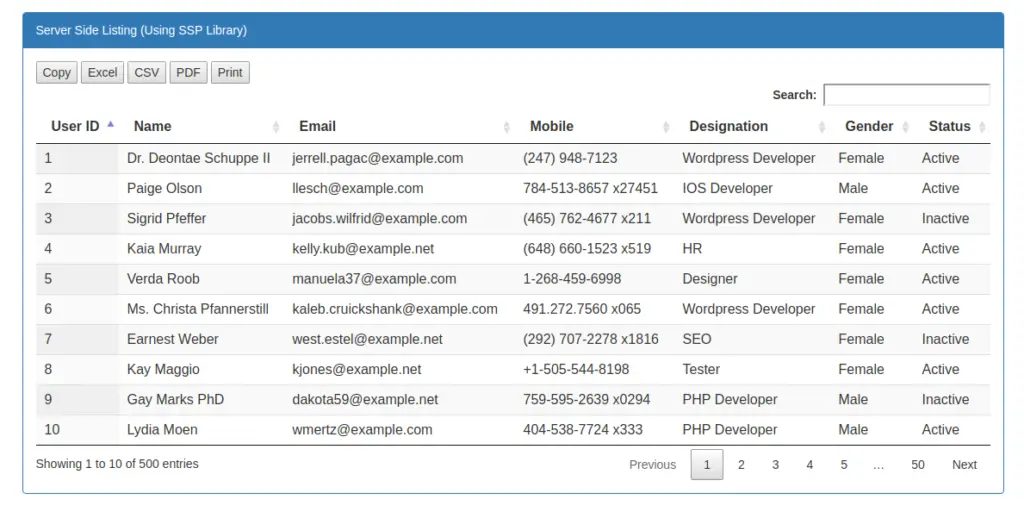
We hope this article helped you to learn about CodeIgniter 4 How To Work with Server Side DataTable in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.
Hello Sanjay Kumar,
I have seen your post and tried to set this up in my own environment.
However I have recognized that you did not follows the MWC-Pattern. So you only build a view and a controller.
Is there a way to move the code in particular the part with the connection to the database and that stuff to the model?
Thanks in advance
Hans peter
Hi, I have given the concept to implement. You need to do followings:
1. Download SSP Library
2. Create Table and dump data
3. Create Routes
4. Create controller and it’s methods
5. Create View layout.
Rest all application should be same.
Done.