By applying advanced natural language processing capabilities, integrating the ChatGPT API into CodeIgniter 4 improves the usefulness of your online apps. CodeIgniter, a sophisticated PHP framework, provides a seamless solution to integrating external APIs, and we’ll walk you through the step-by-step process of integrating the ChatGPT API into CodeIgniter 4 in this tutorial.
Before proceeding with the practical implementation, it is critical to comprehend the significance of implementing ChatGPT into your CodeIgniter projects. ChatGPT, created by OpenAI, enables your applications to understand and answer intelligently to natural language queries, allowing you to create dynamic and engaging user interactions.
Read More: How To Connect CodeIgniter 4 with Multiple Databases?
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
What is ChatGPT?
ChatGPT is a language model developed by OpenAI. It is part of the GPT (Generative Pre-trained Transformer) series, specifically GPT-3.5-turbo. ChatGPT is designed to generate human-like responses based on the input it receives.
Key features of ChatGPT include:
- Natural Language Understanding
- Contextual Understanding
- Versatility
- Large-scale Pre-training
Create an Account and Generate OpenAI API Key
To make use of ChatGPT, create an account on OpenAI platform to generate API keys.
You have to pass an email address and password. It will send you a verification email and later on asks for basic information like Name, Phone number, DOB etc.
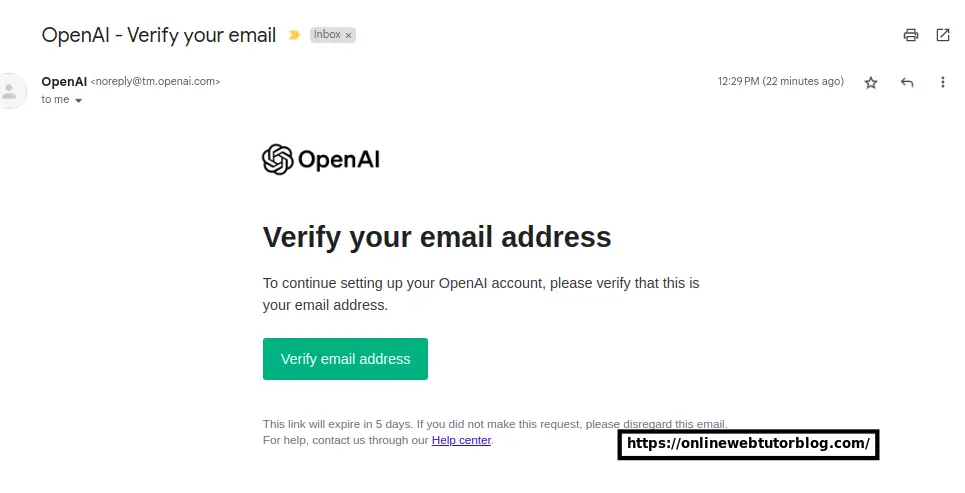
Once your account will be set successfully,
Click on Create New Secret Key
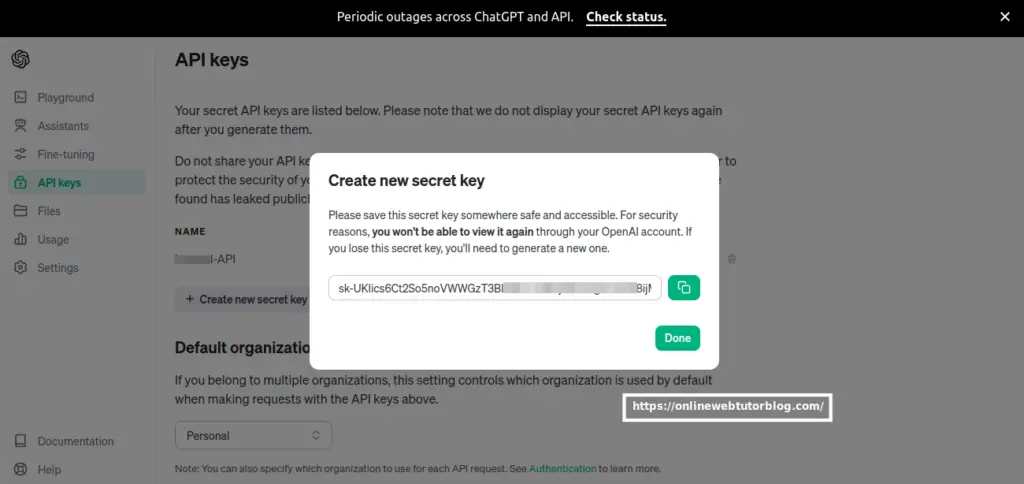
Copy generated secret key.
Read More: Step-by-Step CodeIgniter 4 HMVC Programming Tutorial
Installation of “openai-php/client” Composer Package
Open project terminal and run this command to install “openai-php/client” this composer package.
composer require openai-php/client
Set up OpenAI API Credentials
Open .env file and add this key and it’s value.
OPENAI_API_KEY="sk-UKlics6Ct2So5noVWWGzT3BlbkF....."
Create a ChatGPT Library Class
Create a library class named GeneratorOpenAIService.php inside /app/Libraries folder.
Write this complete code into it,
<?php namespace App\Libraries; use OpenAI; class GeneratorOpenAIService { private $client; public function __construct() { $this->client = OpenAI::client(env('OPENAI_API_KEY')); } public function generateResponseOpenAi(string $question): string { $response = $this->client->completions()->create([ 'model' => 'text-davinci-003', 'temperature' => 0.9, 'top_p' => 1, 'frequency_penalty' => 0, 'presence_penalty' => 0, 'prompt' => $question, 'max_tokens' => 4000, ]); return $response['choices'][0]['text']; } }
Usage of OpenAI Library in a Controller
Use the library class into a controller where you want to use the ChatGPT API,
Create a controller class,
php spark make:controller OpenAIController
It will create a OpenAIController.php file inside /app/Controllers folder.
Open file and write this code into it,
<?php namespace App\Controllers; use App\Controllers\BaseController; use App\Libraries\GeneratorOpenAIService; class OpenAIController extends BaseController { private $openAiService; public function __construct() { $this->openAiService= new GeneratorOpenAIService; } public function chatOpenAi() { $question = "How artificial intelligence is transforming the world?"; if ($question == null) { return redirect("/"); } $response= $this->openAiService->generateResponseOpenAi($question); return $this->response->setJSON([ 'question' => $question, 'response' => $response ]); } }
Read More: How To Create CodeIgniter 4 Custom Library Tutorial
Add Route
Open Routes.php from /app/Config folder. Add this route into it,
//... $routes->get('open-ai', 'OpenAIController::chatOpenAi'); //...
Application Testing
Open project terminal and start development server via command:
php spark serve
URL: http://localhost:8080/open-ai
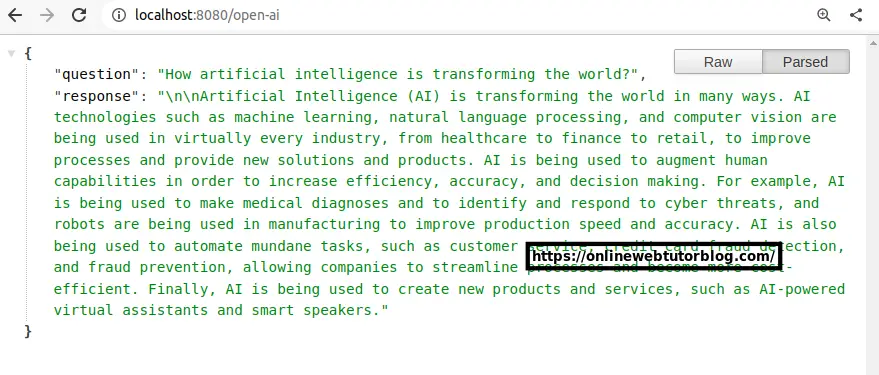
That’s it.
We hope this article helped you to learn about How To Integrate ChatGPT API in CodeIgniter 4 Tutorial Example in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.