CodeIgniter provides a fully-localized, immutable, date/time class that is built on PHP’s DateTime object, but uses the Intl extension’s features to convert times across timezones and display the output correctly for different locales.
This class is the Time class and lives in the CodeIgniter\I18n namespace. Inside this article we will see the concept of Date and Time in CodeIgniter 4. Also we will see available methods of Time Class in great detail.
Learn More –
- Javascript Auto Logout in CodeIgniter 4 Tutorial
- jQuery UI Autocomplete Database Search CodeIgniter 4
- Learn CodeIgniter 4 Tutorial From Beginners To Advance
- Line Chart Integration with CodeIgniter 4 – HighCharts Js
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Instantiating Time Class
To use Time Class in CodeIgniter 4 application, we need to do these things –
- Import CodeIgniter\I18n\Time
- Create Instance of Time()
Here, we have few examples to load and use Time() class.
use CodeIgniter\I18n\Time;
$myTime = new Time('+3 week');
$myTime = new Time('now');
$myTime = new Time('now', 'America/Chicago', 'en_US');
Available Methods of Time Class
There are several methods of Time class available to use to create Date & Time values. Here we will see about methods in two sections. First those methods which creates instance of Time Class and Second which is used to display date and time values to end users.
Also we will see some getter methods of Time class.
Methods – Time Class Object
- now()
- parse()
- today()
- yesterday()
- tomorrow()
- createFromDate()
- createFromTime()
- create()
Methods – Display Value
- toLocalizedString()
- toDateTimeString()
- toDateString()
- toTimeString()
- humanize()
Let’s see all these methods in complete detail.
Details About Time Class Methods
All methods what we discuss is all about for Date & Time value.
now()
now() method that returns a new instance set to the current time. We can pass in strings representing the timezone and the locale in the second and parameters, respectively.
If no locale or timezone is provided, the application defaults will be used.
Example
$myTime = Time::now('America/Chicago', 'en_US');
parse()
This method is a static version of the default constructor. It takes a string acceptable as DateTime’s constructor.
$myTime = Time::parse('next Tuesday', 'America/Chicago', 'en_US');
today()
Returns a new instance with the date set to the current date, and the time set to midnight.
$myTime = Time::today('America/Chicago', 'en_US');
yesterday()
Returns a new instance with the date set to the yesterday’s date and the time set to midnight.
$myTime = Time::yesterday('America/Chicago', 'en_US');
tomorrow()
Returns a new instance with the date set to tomorrow’s date and the time set to midnight.
$myTime = Time::tomorrow('America/Chicago', 'en_US');
createFromDate()
Given separate inputs for year, month, and day, will return a new instance.
$today = Time::createFromDate(); // Uses current year, month, and day
$anniversary = Time::createFromDate(2018);// Uses current month and day
$date = Time::createFromDate(2018, 3, 15, 'America/Chicago', 'en_US');
createFromTime()
createFromDate except it is only concerned with the hours, minutes, and seconds. Uses the current day for the date portion of the Time instance.
$lunch = Time::createFromTime(11, 30) // 11:30 am today
$dinner = Time::createFromTime(18, 00, 00) // 6:00 pm today
$time = Time::createFromTime($hour, $minutes, $seconds, $timezone, $locale);
create()
A combination of the previous two methods, takes year, month, day, hour, minutes, and seconds as separate parameters. Any value not provided will use the current date and time to determine.
$time = Time::create($year, $month, $day, $hour, $minutes, $seconds, $timezone, $locale);
toLocalizedString()
This is the localized version of DateTime’s format() method.
$time = Time::parse('March 9, 2016 12:00:00', 'America/Chicago');
echo $time->toLocalizedString('MMM d, yyyy'); // March 9, 2016
toDateTimeString()
It returns a string formatted as you would commonly use for datetime columns in a database (Y-m-d H:i:s)
$time = Time::parse('March 9, 2016 12:00:00', 'America/Chicago');
echo $time->toDateTimeString(); // 2016-03-09 12:00:00
toDateString()
Displays just the date portion of the Time.
$time = Time::parse('March 9, 2016 12:00:00', 'America/Chicago');
echo $time->toDateString(); // 2016-03-09
toTimeString()
Displays just the time portion of the value.
$time = Time::parse('March 9, 2016 12:00:00', 'America/Chicago');
echo $time->toTimeString(); // 12:00:00
humanize()
This methods returns a string that displays the difference between the current date/time and the instance in a human readable format that is geared towards being easily understood. It can create strings like ‘3 hours ago’, ‘in 1 month’, etc.
// Assume current time is: March 10, 2017 (America/Chicago)
$time = Time::parse('March 9, 2016 12:00:00', 'America/Chicago');
echo $time->humanize(); // 1 year ago
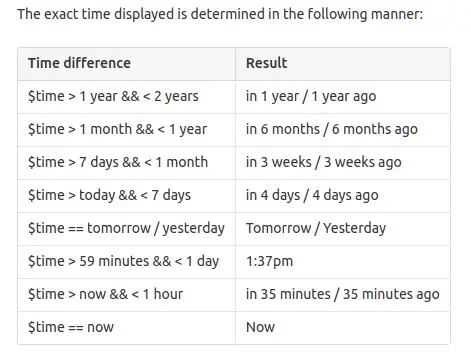
Working with Individual Values – Date Time
The Time object provides a number of methods to allow to get and set individual items, like the year, month, hour, etc, of an existing instance.
$time = Time::parse('August 12, 2016 4:15:23pm');
echo $time->getYear(); // 2016
echo $time->getMonth(); // 8
echo $time->getDay(); // 12
echo $time->getHour(); // 16
echo $time->getMinute(); // 15
echo $time->getSecond(); // 23
echo $time->year; // 2016
echo $time->month; // 8
echo $time->day; // 12
echo $time->hour; // 16
echo $time->minute; // 15
echo $time->second; // 23
We hope this article helped you to learn Date and Time in CodeIgniter 4 Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.