Helpers in CodeIgniter are standalone functions which helps in application to complete some specific task. Inside this article, we will cover Custom helper in CodeIgniter 4. We have several helpers by default provided by CodeIgniter 4 itself. But in some cases we need some of our own.
To create a custom helper we need to follow very few simple basis steps.
Learn More –
- CodeIgniter 4 Truncate/Empty Table Methods Tutorial
- CodeIgniter 4 Upload Image using Ajax Method
- CodeIgniter 4 Views And Layouts Tutorial
- CodeIgniter 4 Working With Database Query
Let’s get started.
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Create Custom Helper
Create a file inside /app/Helpers folder with name say custom_helper.php. Inside this naming convention, you can see we have used suffix as _helper.
The first point, which we have to keep in our mind is all about suffix that should be used as – _helper with the file name, custom is only the name of helper.
Open /app/Helpers/custom_helper.php file –
<?php // Function: used to convert a string to revese in order if (!function_exists("reverse_string")) { function reverse_string(string $string) { return strrev($string); } } // Function: used to create slugs if (!function_exists("slugify")) { function slugify($text) { // replace non letter or digits by - $text = preg_replace('~[^\pL\d]+~u', '-', $text); // transliterate $text = iconv('utf-8', 'us-ascii//TRANSLIT', $text); // remove unwanted characters $text = preg_replace('~[^-\w]+~', '', $text); // trim $text = trim($text, '-'); // remove duplicate - $text = preg_replace('~-+~', '-', $text); // lowercase $text = strtolower($text); if (empty($text)) { return 'n-a'; } return $text; } }
We have added two functions inside this custom helper file. Let’s see how to load and use it.
Load Helper & Use in Application
To load helpers in CodeIgniter 4 application we have two options.
- Load in BaseController.php (parent controller file)
- Load in specific controller file
Load in BaseController.php
Search this $helpers variable and add your helper name into array. When we load helper in parent controller, then it will be available to any of the controller inside application.
protected $helpers = ['custom']; //loading helper
Load in specific controller file
Let’s say we have a controller file Home.php. Inside this controller we need to load helper.
<?php namespace App\Controllers; class Home extends BaseController { public function index() { helper("custom"); // loading helper $name = "Online Web Tutor"; echo "<b>Reverse Function:</b> " . reverse_string($name); // helper function echo "<br/><br/>"; $title = "CodeIgniter 4 Custom Helper Article"; echo "<b>Slug Function: </b>" . slugify($title); // helper function } }
Output
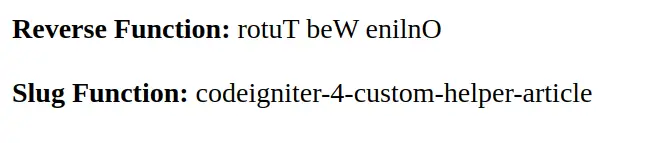
If we have multiple helpers which needs to load then use this way –
helper(["name1", "name2", "name3", ...]);
Helper function after loading via Parent controller is available to use in any file like – views, models, controllers, libraries etc.
We hope this article helped you to learn Custom Helper in CodeIgniter 4 in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.