The ability to generate PDF documents from web applications is useful for tasks such as creating invoices, reports, or printable information. Laravel, a popular PHP framework, provides a number of modules and packages to help in PDF generation.
We will walk you through the process of making PDFs in Laravel 10 using the DomPDF module, allowing you to generate dynamic and configurable PDF documents from your online application.
Throughout this article, we’ll look at how to integrate and use the DomPDF library in Laravel 10, including installation, configuration, and practical examples of dynamically generating PDF documents.
Read More: Laravel 10 Create Seeder for Database Seeding Tutorial
Let’s get started.
What is DomPDF Library?
DomPDF is a free and open-source PHP framework that allows developers to create PDF documents from HTML and CSS. It’s very handy for converting online pages or web-based content to PDF files. DomPDF is written in PHP and can be integrated into PHP applications, making it an attractive solution for projects requiring dynamic PDF creation.
DomPDF’s key features and qualities include:
- HTML to PDF Conversion: DomPDF is capable of converting HTML and CSS material to PDF format. This is useful for generating PDF documents from web pages, reports, invoices, and other web-based content.
- CSS Support: DomPDF supports CSS for formatting PDF content, giving developers control over the layout, fonts, colours, and other aesthetic characteristics of the created PDFs.
- Unicode and Multilingual Support: DomPDF has Unicode support, allowing you to include text in a variety of languages and character sets within PDF documents.
- Image Embedding: Images (such as logos and graphics) can be included in DomPDF PDFs.
- Page Size and Orientation: For the generated PDFs, developers can define page sizes (e.g., A4, Letter) and orientation (portrait or landscape).
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
How To Install DomPDF Package to Laravel?
Here, we will install a pdf package to laravel application.
Open project terminal and run this command
composer require barryvdh/laravel-dompdf
When we install we should see the given image in terminal.
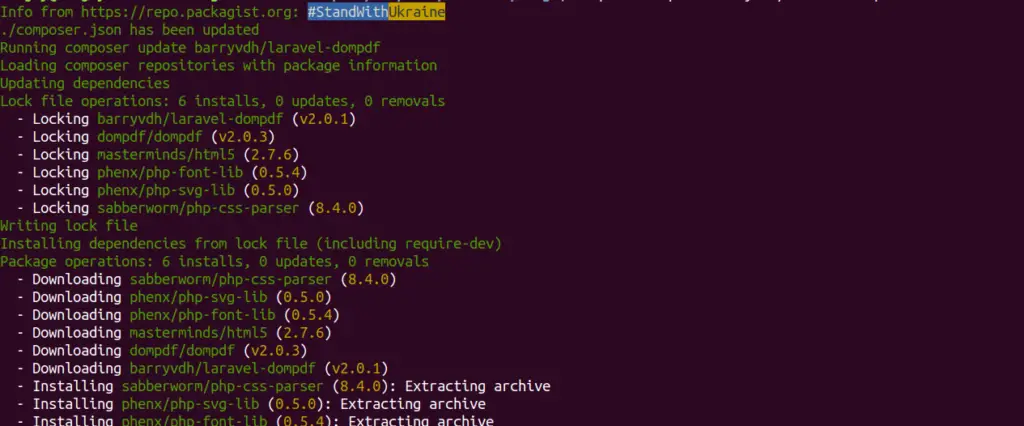
Setup Data Controller
Back to project terminal and run this command into it,
php artisan make:controller SiteController
It will create SiteController.php file inside /app/Http/Controllers folder.
Read More: Laravel 10 Mailable Email Template Components Tutorial
Open file and write this code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Barryvdh\DomPDF\Facade\Pdf; class SiteController extends Controller { public function generatePDF() { $data = [ 'title' => 'Welcome to OnlineWebTutorBlog.com', 'author' => "Sanjay" ]; $pdf = PDF::loadView('my-pdf-file', $data); return $pdf->download('onlinewebtutorblog.pdf'); } }
Concept
Use DomPDF package and it’s method to create pdf file with view.
$pdf = PDF::loadView('my-pdf-file', $data);
return $pdf->download('onlinewebtutorblog.pdf');
It first creates a PDF from a blade template and then download it.
Create Blade Template
Create a file named as my-pdf-file.blade.php inside /resources/views folder.
This template file will be the layout for pdf file.
Open file my-pdf-file.blade.php and write this code into it,
<!DOCTYPE html> <html> <head> <title>Title From OnlineWebTutorBlog</title> </head> <body> <h1>Title: {{ $title }}</h1> <h3>Author: {{ $author }}</h3> <p>ut aliquip ex ea commodoconsequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidata.</p> </body> </html>
Add Route
Open web.php from /routes folder. Add this route into it.
//... use App\Http\Controllers\SiteController; Route::get('generate-pdf', [SiteController::class, 'generatePDF']); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/generate-pdf
Read More: How To Create a Custom 404 Page in Laravel 10 Tutorial
When we type this URL, it will download a pdf file into system.
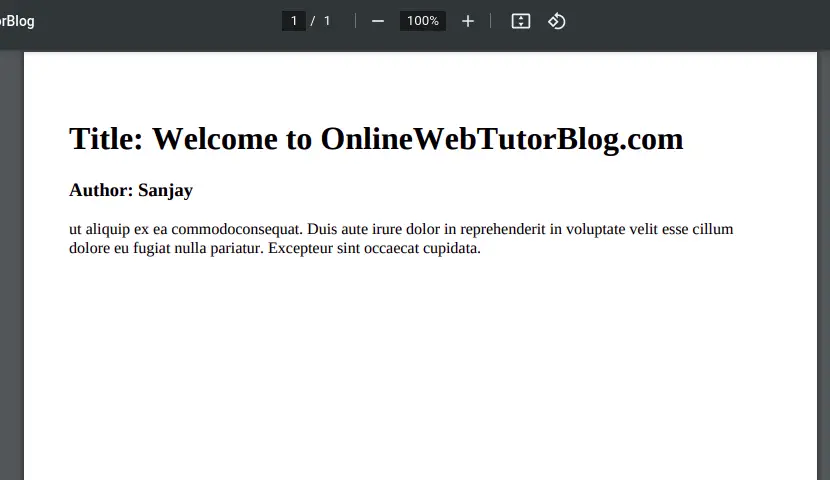
That’s it.
We hope this article helped you to learn about Laravel 10 How To Create PDFs Using DomPDF Library Tutorial Example in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.