The ability to generate PDF files from web applications is a typical requirement for operations like creating invoices, reports, or downloaded documents. Laravel, a popular PHP framework, has a number of modules and packages that help to simplify PDF generation.
We will walk you through the process of creating PDF files in Laravel 10 using the TCPDF package, allowing you to generate dynamic and customised PDF documents from your web application.
By the end of this lesson, you’ll be able to create PDF files in Laravel 10, allowing you to add PDF generation capabilities to your web site for a variety of use cases.
Read More: Laravel 10 How To Create Macro with Examples Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
What is TCPDF Library?
TCPDF is a free and open-source PHP library for producing and manipulating PDF documents (TCPDF stands for “TCPDF – PHP class for PDF“). In web development, it is widely used to generate dynamic PDF files from PHP applications. For creating PDFs, TCPDF offers a variety of features and options, including text, graphics, tables, and more.
Here are some of the TCPDF library’s important features and characteristics:
- PDF Creation: With TCPDF, developers may create new PDF documents from scratch or alter existing PDFs. It allows you to create text-based PDFs as well as documents containing graphics, fonts, and different formatting options.
- Text and Font Support: TCPDF supports a variety of typefaces and text formatting choices, such as font embedding, character encoding, and Unicode text. It supports the use of custom fonts in PDF documents.
- Image Integration: Using TCPDF, you may add images to PDF documents, allowing you to include logos, graphics, or other visual elements. It accepts popular image formats like JPEG, PNG, and GIF.
- Page Layout and Formatting: TCPDF allows you to customise your page layout, size, margins, and orientation. Developers can also specify headers, footers, and page numbers.
How To Install TCPDF Libary in Laravel?
Open project terminal and run this command,
composer require elibyy/tcpdf-laravel
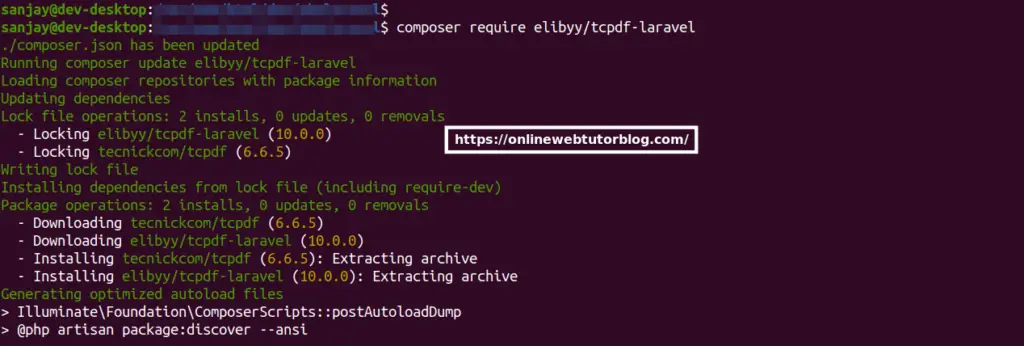
Next,
Setup PDF Controller
Open project into terminal and run this command.
php artisan make:controller PDFController
Read More: Laravel 10 How To Redirect with Form Inputs Tutorial
It will create a PDFController.php file inside /app/Http/Controllers folder.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Elibyy\TCPDF\Facades\TCPDF; class PDFController extends Controller { public function index() { $filename = 'sample.pdf'; $data = [ 'title' => 'Laravel 10 How To Create PDF Files Using TCPDF Library' ]; $html = view()->make('pdf-file', $data)->render(); $pdf = new TCPDF; $pdf::SetTitle('Generate PDF Using Laravel Library'); $pdf::AddPage(); $pdf::writeHTML($html, true, false, true, false, ''); $pdf::Output(public_path($filename), 'F'); return response()->download(public_path($filename)); } }
Create Blade Template File
Create a file pdf-file.blade.php inside /resources/views folder.
Open file and write this code into it,
<!DOCTYPE html> <html> <head> <title>Laravel 10 How To Create PDF Files Using TCPDF Library</title> </head> <body> <h1 style="color:red;">{!! $title !!}</h1> <br> <p> -- PDF Content -- </p> <p> -- PDF Content -- </p> </body> </html>
Add Route
Open web.php file from /routes folder. Add this route into it,
//... use App\Http\Controllers\PDFController; Route::get('pdf', [PDFController::class,'index']); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/pdf
Read More: Laravel 10 How To Integrate Ckeditor Plugin Tutorial
When we hit the above URL, it downloads a PDF file i.e sample.pdf into your system as well as save to your public folder of application.
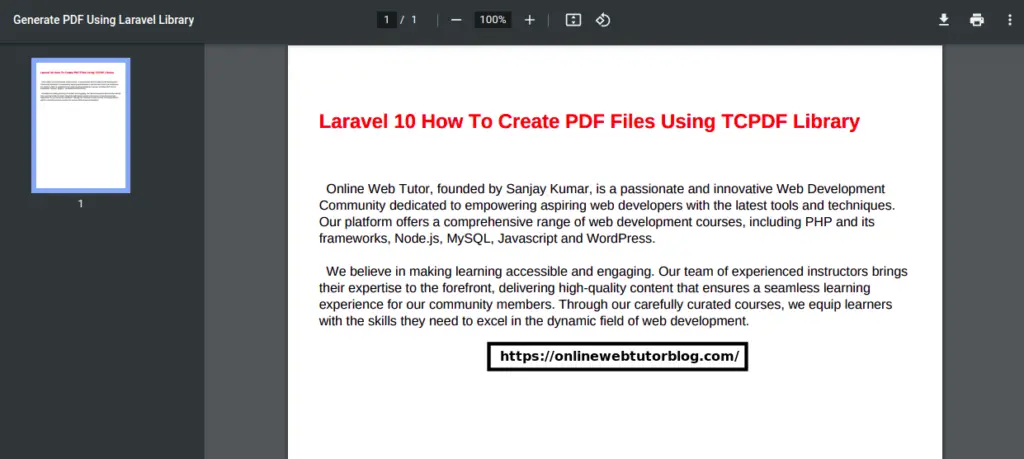
That’s it.
We hope this article helped you to learn Laravel 10 How To Create PDF Files Using TCPDF Library in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.