Inside this article we will see the concept of find and extract all images from a HTML string in php or from a web page. Concept of this article will provide very classified information to understand the things.
This PHP tutorial is based on how to extract all images and their attributes from a web page or from a HTML string. In this guide, we will see how to fetch the HTML content of a web page by URL and then extract the images from it. To do this, we will be use PHP’s DOMDocument class.
DOMDocument of PHP also termed as PHP DOM Parser. We will see step by step concept to find and extract all images from a html using DOM parser.
Learn More –
- Find and Extract All links From a HTML String in PHP
- How To Generate Fake Data in PHP Using Faker Library
- How to Generate Fake Image URLs in PHP Using Faker
- How to Generate Random Name of Person in PHP
Let’s get started.
Example 1: Get All Images From HTML String Value
Inside this example we will consider a HTML string value. From that html value we will extract all Images and their attributes.
Create file index.php inside your application.
Open index.php and write this complete code into it.
<?php $htmlString = "<html> <head></head> <body> <img src='/images/abc1.png' title='sample image 1' alt='alt sample image 1'/> <img src='/images/abc2.png' title='sample image 2' alt='alt sample image 2'/> <img src='/images/abc3.png' title='sample image 3' alt='alt sample image 3'/> </body> </html>"; //Create a new DOMDocument object. $htmlDom = new DOMDocument; //Load the HTML string into our DOMDocument object. @$htmlDom->loadHTML($htmlString); //Extract all images elements / tags from the HTML. $imageTags = $htmlDom->getElementsByTagName('img'); //Create an array to add extracted images to. $extractedImages = array(); //Loop through the images tags that DOMDocument found. foreach($imageTags as $imgTag){ //Get the src attribute of the img tag. $imgSrc = $imgTag->getAttribute('src'); //Get the title attribute of the img tag. $imgTitle = $imgTag->getAttribute('title'); //Get the alt attribute of the img tag. $imgAlt = $imgTag->getAttribute('alt'); //Add the image details to $extractedImages array. $extractedImages[] = array( 'src' => $imgSrc, 'title' => $imgTitle, 'alt' => $imgAlt ); } echo "<pre>"; //print_r our array of images. print_r($extractedImages);
Concept

Output
When we run index.php. Here is the output
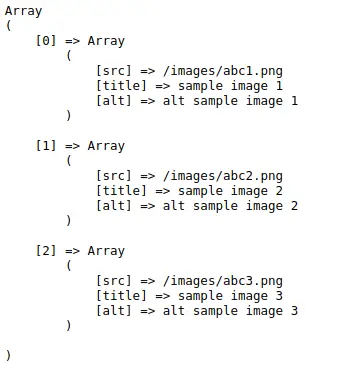
Example 2: Get All Images From a Web Page
Inside this example we will use web page URL to get all images and it’s attributes.
Create file index.php inside your application.
Open index.php and write this complete code into it.
<?php $htmlString = file_get_contents('https://onlinewebtutorblog.com/'); //Create a new DOMDocument object. $htmlDom = new DOMDocument; //Load the HTML string into our DOMDocument object. @$htmlDom->loadHTML($htmlString); //Extract all images elements / tags from the HTML. $imageTags = $htmlDom->getElementsByTagName('img'); //Create an array to add extracted images to. $extractedImages = array(); //Loop through the anchors tags that DOMDocument found. foreach($imageTags as $imgTag){ //Get the src attribute of the img tag. $imgSrc = $imgTag->getAttribute('src'); //Get the title attribute of the img tag. $imgTitle = $imgTag->getAttribute('title'); //Get the alt attribute of the img tag. $imgAlt = $imgTag->getAttribute('alt'); //Add the image details to $extractedImages array. $extractedImages[] = array( 'src' => $imgSrc, 'title' => $imgTitle, 'alt' => $imgAlt ); } echo "<pre>"; //print_r our array of images. print_r($extractedImages);
Output
When we run index.php. Here is the output
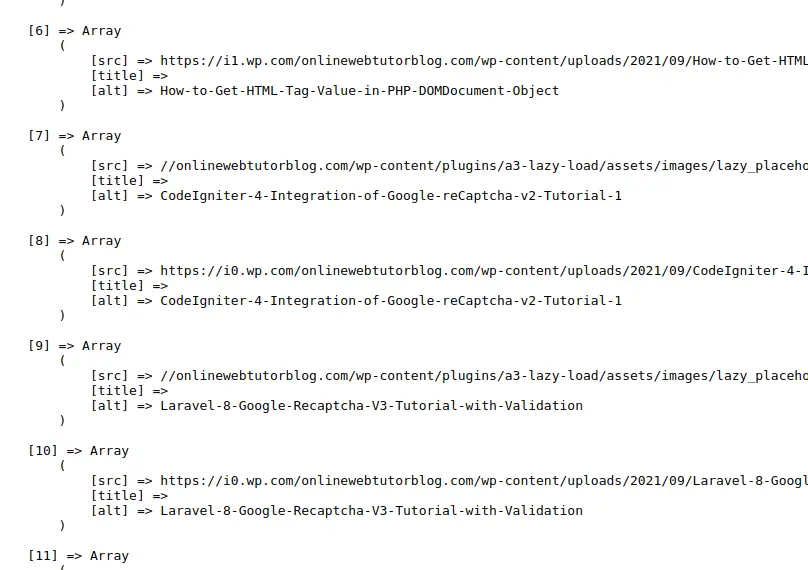
We hope this article helped you to Find and Extract All Images From a Web Page in PHP Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.