While working in any application, sometimes we need lots of dummy data to test the workflow of entire application. Inside this article we will see how to generate random name of person in PHP with or without using faker library.
Faker library needs to be install by using composer. Once install, there are several properties, methods available to generate various type of fake data like Name, Email, Phone number etc.
This article is super easy to understand and implement in php programming. To use Faker library, your system must have composer installed. If don’t, please follow this article to install in Linux Operating System. How To Install Composer in Ubuntu 18.04 ?
Learn More –
- How To Set Sublime Text Editor to FileZilla as Editor ?
- How to Work with vi Editor – Ubuntu
- jQuery Ajax File Upload with Form Data using PHP
To do for this article –
- Generate Fake data for Name without using any library
- Generate Names using Faker Library
Let’s get started.
Random Names without Using Any Library
Let’s create an application in PHP.
Create a folder with name php-random. Inside this folder create a file like index.php.
Open index.php and write this complete code into it.
<?php // Function to return the name of a person on every call function generateRandomName() { $firstname = array( 'Johnathon', 'Anthony', 'Erasmo', 'Raleigh', 'Nancie', 'Tama', 'Camellia', 'Augustine', 'Christeen', 'Luz', 'Diego', 'Lyndia', 'Thomas', 'Georgianna', 'Leigha', 'Alejandro', 'Marquis', 'Joan', 'Stephania', 'Elroy', 'Zonia', 'Buffy', 'Sharie', 'Blythe', 'Gaylene', 'Elida', 'Randy', 'Margarete', 'Margarett', 'Dion', 'Tomi', 'Arden', 'Clora', 'Laine', 'Becki', 'Margherita', 'Bong', 'Jeanice', 'Qiana', 'Lawanda', 'Rebecka', 'Maribel', 'Tami', 'Yuri', 'Michele', 'Rubi', 'Larisa', 'Lloyd', 'Tyisha', 'Samatha', ); $lastname = array( 'Mischke', 'Serna', 'Pingree', 'Mcnaught', 'Pepper', 'Schildgen', 'Mongold', 'Wrona', 'Geddes', 'Lanz', 'Fetzer', 'Schroeder', 'Block', 'Mayoral', 'Fleishman', 'Roberie', 'Latson', 'Lupo', 'Motsinger', 'Drews', 'Coby', 'Redner', 'Culton', 'Howe', 'Stoval', 'Michaud', 'Mote', 'Menjivar', 'Wiers', 'Paris', 'Grisby', 'Noren', 'Damron', 'Kazmierczak', 'Haslett', 'Guillemette', 'Buresh', 'Center', 'Kucera', 'Catt', 'Badon', 'Grumbles', 'Antes', 'Byron', 'Volkman', 'Klemp', 'Pekar', 'Pecora', 'Schewe', 'Ramage', ); $name = $firstname[rand(0, count($firstname) - 1)]; $name .= ' '; $name .= $lastname[rand(0, count($lastname) - 1)]; return $name; } // for single name echo generateRandomName(); // for 10 person names for ($i = 0; $i < 10; $i++) { echo generateRandomName() . "<br/>"; }
To use function, simply call it by it’s name as –
echo generateRandomName();
Random Names Using Faker Library
Create a folder with name php-faker. Inside this folder create these files –
- composer.json
- index.php
We need composer.json file because we will install faker library. Write this curly pairs in composer.json file.
{}
If you don’t write this, probably you will get this error on terminal when we install composer package.
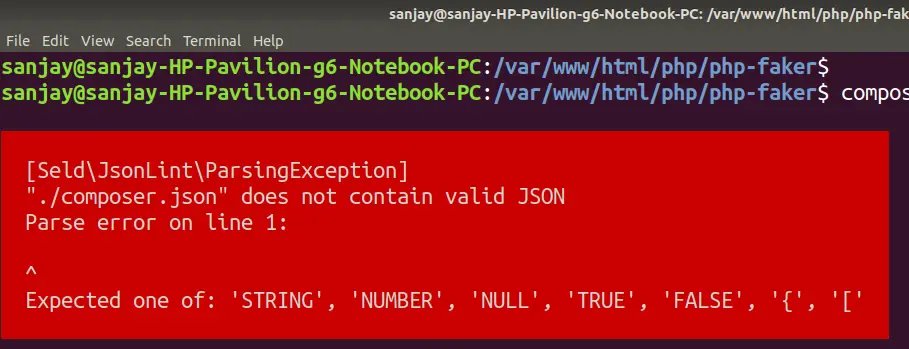
Install Faker Library
Open project in terminal and run this command.
$ composer require fzaninotto/faker
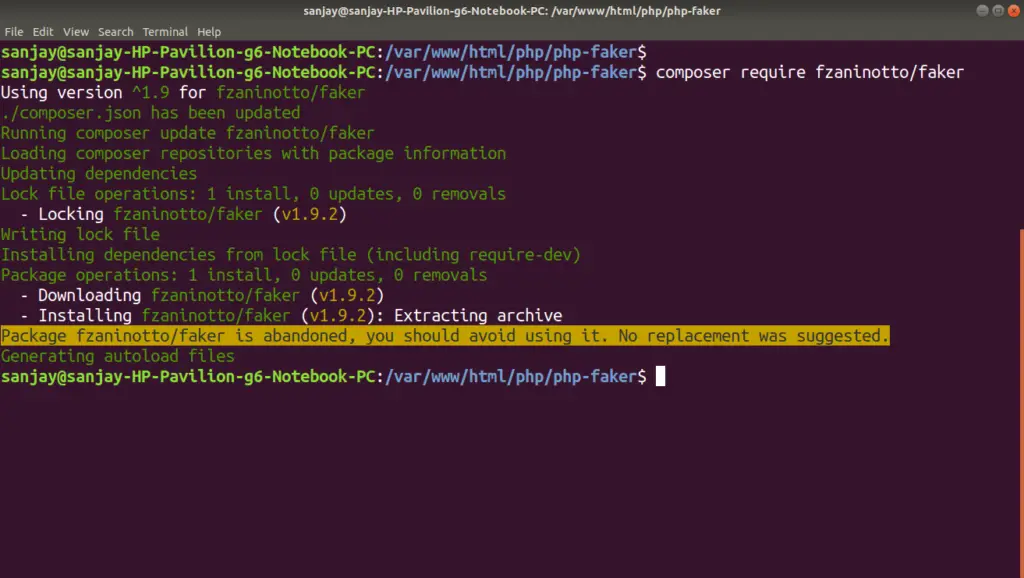
After installation of this package, automatically composer.json file get updated.
{ "require": { "fzaninotto/faker": "^1.9" } }
Also you will see inside your application setup, you have now a vendor folder. This is package installed folder, don’t do anything with that.
Load Faker Library to Application
Open index.php file and use require_once php function to include faker library.
<?php require_once 'vendor/autoload.php'; // use the factory to create a Faker\Generator instance $faker = Faker\Factory::create(); ...
Use Faker\Factory::create()
to create and initialize a faker generator.
Using Faker Library Property
Open index.php and write this code into it. This is for getting single name of person
<?php require_once 'vendor/autoload.php'; // use the factory to create a Faker\Generator instance $faker = Faker\Factory::create(); // generate data by accessing properties echo $faker->name; // 'Lucy Cechtelar';
Generate 100 Test Users
<?php require_once 'vendor/autoload.php'; // use the factory to create a Faker\Generator instance $faker = Faker\Factory::create(); for ($i = 0; $i < 100; $i++) { echo $faker->name . "<br/>"; }
We hope this article helped you to learn How to Generate Random Name of Person in PHP in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.