Inside this article we will see how to copy entire contents of a directory to another directory in PHP. Given a directory and the task is to copy the content of the directory to another directory using PHP functions.
Following PHP functions will be used to copy the contents from one folder to another –
- PHP copy() Function: The copy() function is used to make a copy of a specified file. Syntax –
bool copy($source, $dest)
It makes a copy of the source file to the destination file. It returns boolean value means on success returns true and when failed returns false value.
- PHP opendir() Function: The opendir() function is used to open a directory handle. Syntax –
opendir($path, $context)
- PHP is_dir() Function: The is_dir() function is used to check whether the specified file is a directory or not. Syntax –
is_dir($file)
- PHP scandir( ) Function: The scandir() function is used to return an array of files and directories of the specified directory.
scandir(directory, sorting_order, context)
- PHP readdir() Function: The readdir() function is used to return the name of the next entry in a directory. Syntax –
readdir(dir_handle)
Learn More –
- How To Generate Fake Data in PHP Using Faker Library
- How to Generate Random Name of Person in PHP
- How To Install Composer in Ubuntu 18.04 ?
- How To Set Sublime Text Editor to FileZilla as Editor ?
Let’s get started.
Folders Setup – Example
Suppose we have a folder with name folder-1 which contains few files and folders inside it.
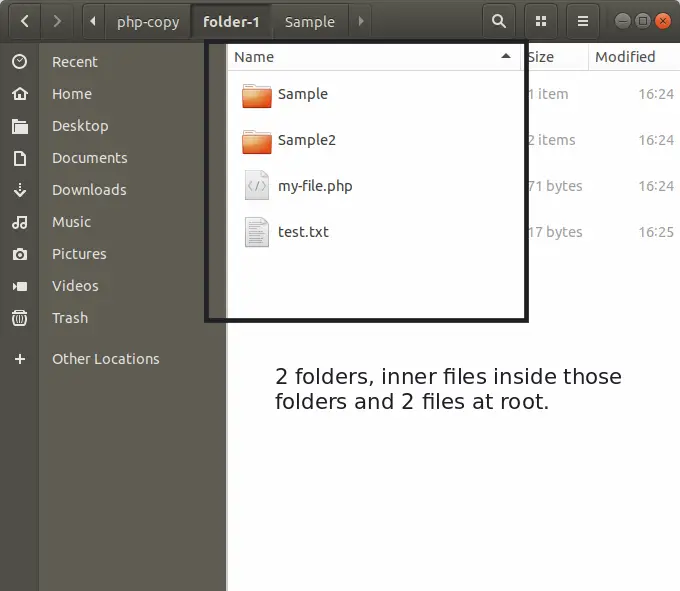
Now, we need to copy the entire files and folders from folder-1 to folder-2(will be created dynamically).
Create a PHP Application
Let’s create a folder with name php-copy. Inside this folder create a file with name index.php.
Example #1
Open this file and write this complete code into it.
<?php function copy_folder($src, $dst) { // open the source directory $dir = opendir($src); // Make the destination directory if not exist @mkdir($dst); // Loop through the files in source directory while( $file = readdir($dir) ) { if (( $file != '.' ) && ( $file != '..' )) { if ( is_dir($src . '/' . $file) ) { // Recursively calling custom copy function // for sub directory copy_folder($src . '/' . $file, $dst . '/' . $file); } else { copy($src . '/' . $file, $dst . '/' . $file); } } } closedir($dir); } // Make sure source folder have sufficient permission to read files $src = "/var/www/html/php/php-copy/folder-1"; $dst = "/var/www/html/php/php-copy/folder-2"; copy_folder($src, $dst); ?>
When we execute this application, it will copy contents of folder-1 folder to folder-2 folder.
Here, we have used $file = readdir($dir),
We have also other option to use scandir() PHP function to do same task.
Example #2
<?php function copy_folder($src, $dst) { // open the source directory $dir = opendir($src); // Make the destination directory if not exist @mkdir($dst); // Loop through the files in source directory foreach (scandir($src) as $file) { if (( $file != '.' ) && ( $file != '..' )) { if ( is_dir($src . '/' . $file) ) { // Recursively calling custom copy function // for sub directory copy_folder($src . '/' . $file, $dst . '/' . $file); } else { copy($src . '/' . $file, $dst . '/' . $file); } } } closedir($dir); } // Make sure source folder have sufficient permission to read files $src = "/var/www/html/php/php-copy/folder-1"; $dst = "/var/www/html/php/php-copy/folder-2"; copy_folder($src, $dst); ?>
Here, we have used the scandir($src) to scan all files and folders from source directory.
In case, if you want to give some permission to created folder. You need to use PHP chmod() function.
chmod($destination, 0755 or 0777 or ...);
Note*: Source & Destination folders should have all proper permissions to read and write. If folders don’t have, Copy will not be done.
We hope this article helped you to learn Copy Entire Contents of a Directory to Another Directory in PHP in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.