Inside this article we will see how to get client ip address in node js. When we building an application which can be run from anywhere then if we want to get client ip address this tutorial will help you to understand.
Article is very easy to understand. We will cover this topic i.e How to get client IP Address in Node js in several methods. How to use getClientIp function in request-ip also we will understand.
The IP address is a 32-bit number that uniquely identifies a network interface on a machine. An IP address is typically written in decimal digits, formatted as four 8-bit fields separated by periods. Each 8-bit field represents a byte of the IP address.
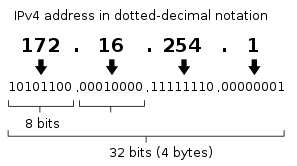
Learn More –
- Create Models in Node Js Sequelize ORM
- How To Connect MySQL Database in Node Js Application
- Node Express Sequelize ORM CRUD APIs with MySQL
Let’s get started.
Node Js Application Setup
Please make sure you have node and npm both installed. To check available versions use these commands.
To check node version
$ node -v
To check npm version
$ npm -v
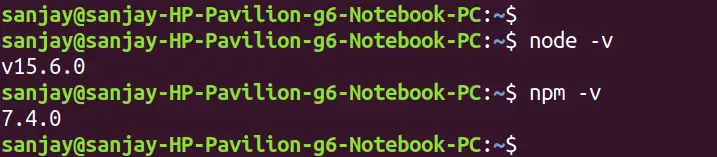
Create a folder with any name say find-ip. Open this folder into terminal or command prompt.
Next, we need package.json file. Run this given command into terminal
$ npm init -y
The given command will auto generate package.json file with default values.
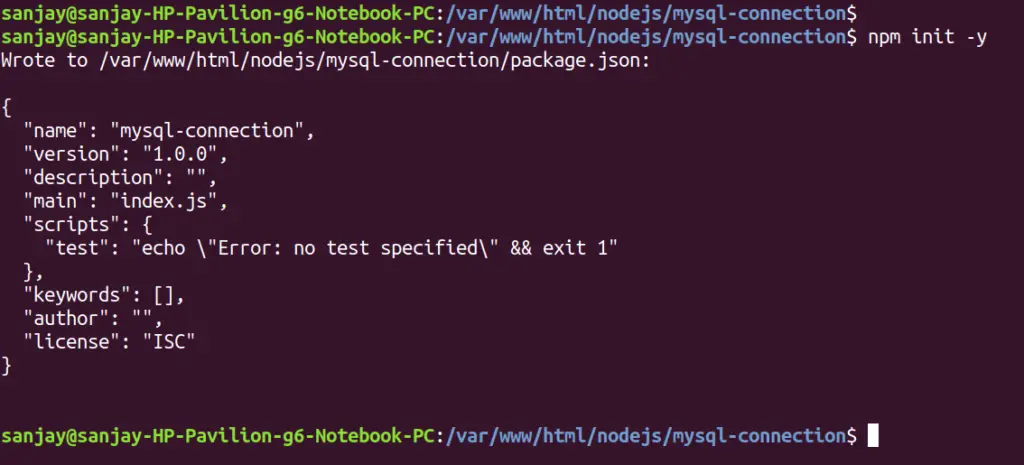
Next we need to create a file say server.js into node application.
Method #1 Client IP Address
Open project into terminal and install this package.
$ npm install express
Open server.js file and write this complete code into it.
var express = require('express'); var app = express(); app.get('/', function(request, response) { var idAddress = request.connection.remoteAddress; console.log(idAddress); // Find IP Address }); app.listen(3000, () => console.log(`App listening on port 3000`))
Concept
var idAddress = request.connection.remoteAddress; console.log(idAddress); // Find IP Address
How To Test
Back to project terminal and start server
$ node server.js
Open browser – http://localhost:3000
Check terminal, it will print IP address.
Method #2 Client IP Address
Open project into terminal and install this package.
$ npm install express
Open server.js file and write this complete code into it.
var express = require('express'); var app = express(); app.get('/', function(request, response) { var idAddress = request.header('x-forwarded-for') || request.connection.remoteAddress; console.log(idAddress); }); app.listen(3000, () => console.log(`App listening on port 3000`))
Concept
var idAddress = request.header('x-forwarded-for') || request.connection.remoteAddress; console.log(idAddress);
How To Test
Back to terminal and start server
$ node server.js
Open browser – http://localhost:3000
Check terminal, it will print IP address.
Method #3 Client IP Address
Open project into terminal and install this package.
$ npm install express $ npm install request-ip
Open server.js file and write this complete code into it.
var express = require('express'); var app = express(); var requestIp = require('request-ip'); app.get('/', function(request, response) { var clientIp = requestIp.getClientIp(request); console.log(clientIp); }); app.listen(3000, () => console.log(`App listening on port 3000`))
Concept
var clientIp = requestIp.getClientIp(request); console.log(clientIp);
How To Test
Back to project terminal and start server
$ node server.js
Open browser – http://localhost:3000
Check terminal, it will print IP address.
We hope this article helped you to learn How to Get Client IP Address in Node JS Application in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.