This tutorial will show you how to get unique values in Laravel 10 collections. As a Laravel developer, you frequently meet situations in which you must extract different elements from a collection while avoiding duplicates.
In this quality guideline you will see the concept of Step-by-Step How To Get Unique values in Laravel Collection.
The Illuminate\Support\Collection
class provides a fluent, convenient wrapper for working with arrays of data. For example, check out the following code. We’ll use the collect
helper to create a new collection instance from the array.
As mentioned above, the collect
helper returns a new Illuminate\Support\Collection
instance for the given array.
Read More: Step-by-Step To Get First and Last Item in Laravel 10 Collection
So, creating a collection is as simple as:
$collection = collect([1, 2, 3]);
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Example #1: Get Unique (Single Dimensional Collection Array)
In this example we will consider a single dimensional array. We will see how to get only unique values from it.
Read More: Step-by-Step Guide To Merge Eloquents in Laravel 10 Collection
Suppose we have SiteController.php a controller file inside /app/Http/Controllers folder.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class SiteController extends Controller { public function index() { $data = [1,2,2,3,1,4,3,5,6,7,8,7,8,9,9,5]; $data = collect($data)->unique(); dd($data); } }
Output
When we execute this code snippet, then we will get something like this –
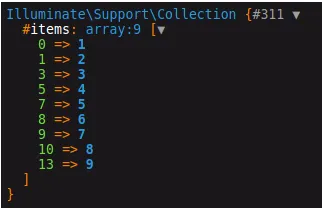
Example #2: Get Unique (Multi Dimensional Collection Array)
In this example we will consider a multi dimensional array. We will see how to get only unique values from it.
Same controller file we are considering for this demo as well.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class SiteController extends Controller { public function index() { $data = collect([ [ ["id" => 1, "name" => "Sanjay", "email" => "sanjay@gmail.com"], ["id" => 2, "name" => "Vijay", "email" => "vijay@gmail.com"], ["id" => 1, "name" => "Sanjay", "email" => "sanjay@gmail.com"], ["id" => 3, "name" => "Ashish", "email" => "ashish@gmail.com"], ["id" => 3, "name" => "Ashish", "email" => "ashish@gmail.com"] ], [ ["id" => 1, "name" => "Sanjay", "email" => "sanjay@gmail.com"], ["id" => 2, "name" => "Vijay", "email" => "vijay@gmail.com"], ["id" => 2, "name" => "Vijay", "email" => "vijay@gmail.com"] ] ]); $data = $data->map(function ($array) { return collect($array)->unique('id')->all(); }); dd($data); } }
Read More: How To Get Last Record of Database Table in Laravel 10
Output
When we execute this code snippet, then we will get something like this –
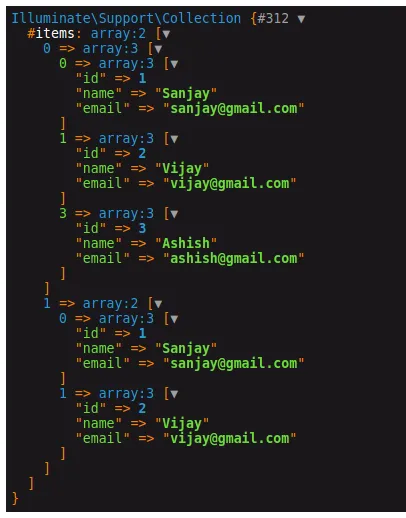
We hope this article helped you to learn How To Get Unique values in Laravel 10 Collection Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.