Many web applications and document management systems require the conversion of Word documents (Docx files) into PDF files. This conversion enables easy sharing, printing, and document integrity.
In this tutorial, we will show you how to convert Word Docx files to PDF files in Laravel 10, the most recent version of the popular PHP framework.
PDF files are a widely accepted and consistent format for exchanging and printing documents. Converting Word documents to PDF ensures that they retain their original formatting, fonts, and layout, making them accessible and legible across all platforms.
Read More: Laravel 10 Model Create Events Example Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Installation of Laravel DomPDF and PhpWord Package
Here, we will install a pdf and phpword package to laravel application.
Open project terminal and run this command,
DomPDF Package
composer require barryvdh/laravel-dompdf
PhpWord Package
composer require phpoffice/phpword
Setup Word To PDF Controller
Back to project terminal and run this command into it,
php artisan make:controller WordToPDFController
It will create a WordToPDFController.php file inside /app/Http/Controllers folder.
Read More: Laravel 10 How to Add Qr Code in PDF Using DomPDF
Open file and write this code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\View\View; class WordToPDFController extends Controller { public function index(): View { return view('word-pdf'); } public function store(Request $request) { $fileName = "doc_".time().'.'.$request->file->extension(); $request->file->move(public_path('uploads'), $fileName); $domPdfPath = base_path('vendor/dompdf/dompdf'); \PhpOffice\PhpWord\Settings::setPdfRendererPath($domPdfPath); \PhpOffice\PhpWord\Settings::setPdfRendererName('DomPDF'); $Content = \PhpOffice\PhpWord\IOFactory::load(public_path('uploads/'.$fileName)); $PDFWriter = \PhpOffice\PhpWord\IOFactory::createWriter($Content,'PDF'); $pdfFileName = "doc_".time().'.pdf'; $PDFWriter->save(public_path('uploads/'.$pdfFileName)); return response()->download(public_path('uploads/'.$pdfFileName)); } }
Create Blade Template
Create a file named as word-pdf.blade.php inside /resources/views folder.
This view file will display an upload button where docx file will be uploaded with a submit button.
Open file and write this code into it,
<!DOCTYPE html> <html lang="en"> <head> <title>Laravel 10 Convert Word Doc File to PDF File Example</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.7.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <h3>Laravel 10 Convert Word Doc File to PDF File Example</h3> <div class="panel panel-primary"> <div class="panel-heading">Word Document to PDF</div> <div class="panel-body"> <form action="{{ route('word.to.pdf.store') }}" method="post" enctype="multipart/form-data"> @csrf <div class="form-group"> <label for="file">Upload Word File:</label> <input type="file" class="form-control (docx)" id="file" name="file"> </div> <button type="submit" class="btn btn-success">Submit</button> </form> </div> </div> </div> </body> </html>
Add Route
Open web.php from /routes folder. Add this route into it,
//... use App\Http\Controllers\WordToPDFController; Route::get('word-to-pdf', [WordToPDFController::class, 'index']); Route::post('word-to-pdf', [WordToPDFController::class, 'store'])->name('word.to.pdf.store'); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/word-to-pdf
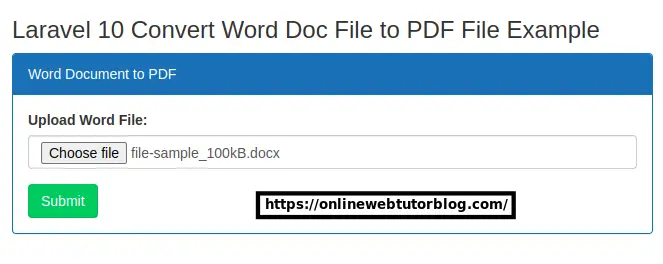
Read More: How To Convert an Image to Webp in Laravel 10 Tutorial
Once you upload and click on Submit button, it will download a PDF version of your uploaded document.
Additionally, your doc file and pdf file both will be uploaded inside /public/uploads folder.
PDF file as,
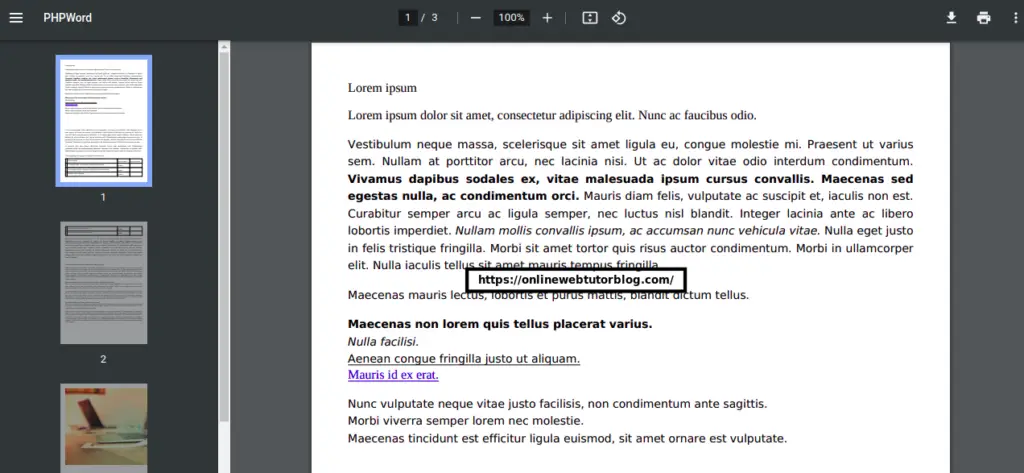
That’s it.
We hope this article helped you to learn about Laravel 10 Convert Word Docx File to PDF File Example Tutorial Example in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.