QR codes have evolved into an indispensable tool for connecting the physical and digital worlds, and incorporating them into PDF documents can be extremely beneficial.
We’ll walk you through the process of adding QR codes to PDF documents in Laravel 10 using the DomPDF module in this article. This tool can be used to include contact information, links, or event details in your PDFs, among other things.
QR codes allow users to quickly access digital content by scanning the code with a smartphone or QR code reader. This function is useful for quickly and easily exchanging URLs, contact information, event details, and other information.
Read More: Laravel 10 How to Add Barcode in PDF Using DomPDF
Let’s get started.
What is QR Code?
A QR code, which stands for “Quick Response code” is a two-dimensional matrix barcode that was invented in Japan to track automotive parts. Because of their adaptability and capacity to contain a substantial amount of data, QR codes have now become immensely popular and are utilised for a range of applications.
Example,
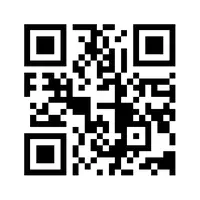
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Installation of Laravel DomPDF and QR Code Package
Here, we will install a pdf and qrcode package to laravel application.
Open project terminal and run this command,
DomPDF Package
composer require barryvdh/laravel-dompdf
QR Code Package
composer require simplesoftwareio/simple-qrcode
Setup PDF Controller
Back to project terminal and run this command into it,
php artisan make:controller PDFController
It will create a PDFController.php file inside /app/Http/Controllers folder.
Read More: How To Convert an Image to Webp in Laravel 10 Tutorial
Open file and write this code into it,
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use SimpleSoftwareIO\QrCode\Facades\QrCode; use PDF; class PDFController extends Controller { public function generatePDF() { $qrcode = base64_encode(QrCode::format('svg')->size(200)->errorCorrection('H')->generate('string')); $data = [ 'title' => 'Welcome to Online Web Tutor', 'qrcode' => $qrcode ]; $pdf = PDF::loadView('my-pdf', $data); return $pdf->download('onlinewebtutor.pdf'); } }
Create Blade Template
Create a file named as my-pdf.blade.php inside /resources/views folder.
This template file will be the layout for pdf file with qr code image in it.
Open file and write this code into it,
<!DOCTYPE html> <html> <head> <title>Laravel 10 How to Add QR Code in PDF Using DomPDF</title> </head> <body> <div> <h1>Laravel PDF with QR Code Example</h1> <p> Phasellus nonummy? Porta venenatis magnam, tortor? Vivamus elementum hac magna mi ut magni praesent fugit per! Aute molestias sapiente recusandae adipiscing imperdiet cupidatat temporibus per nibh duis etiam? Illo malesuada! Exercitation cum recusandae iaculis vel, proident faucibus deleniti minus diamlorem, quisque suscipit? Nunc rerum, ligula felis ullamcorper posuere, anim, vehicula sollicitudin iusto maxime perferendis curae? Voluptate metus excepturi ultricies cum! Viverra vero libero ratione interdum rerum. Nemo debitis commodi expedita, esse rutrum? Faucibus nulla eos, wisi ab adipiscing? Ligula, hendrerit, porttitor laoreet purus molestiae, sodales augue eius perspiciatis, interdum parturient? Error odit? Vel, ullamcorper netus occaecati odio! Eos minima expedita. </p> <img src="data:image/png;base64,{{ $qrcode }}"> </div> </body> </html>
Add Route
Open web.php from /routes folder. Add this route into it,
//... use App\Http\Controllers\PDFController; Route::get('generate-pdf', [PDFController::class, 'generatePDF']); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Read More: Laravel 10 How To Use Multiple Database Connection
URL: http://127.0.0.1:8000/generate-pdf
When we type this URL, it will download a pdf file into system,
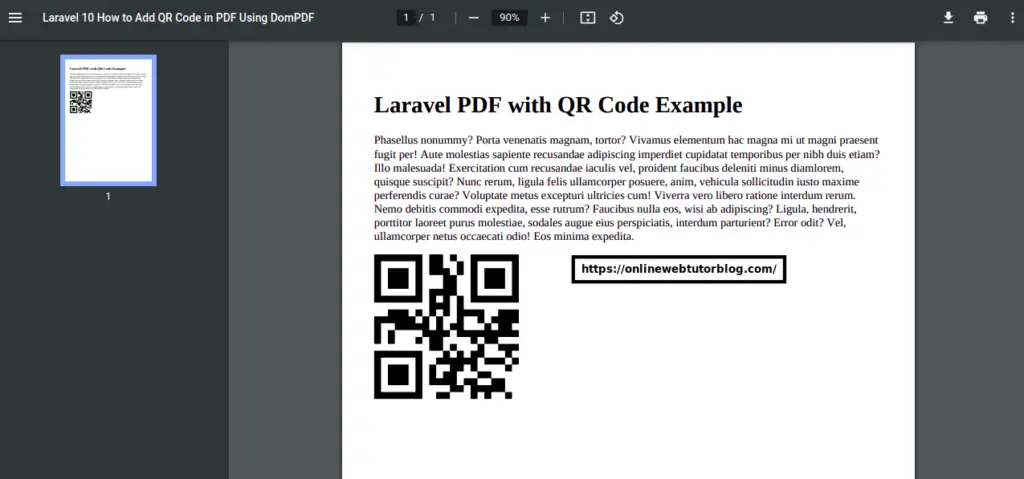
That’s it.
We hope this article helped you to learn about Laravel 10 How to Add Qr Code in PDF Using DomPDF Tutorial Example in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.