Gathering browser information from user interactions in a web application can provide useful insights for analytics, troubleshooting, and improving the user experience. Laravel 10, a sophisticated PHP framework, provides an easy way to get browser information from incoming HTTP requests.
In this tutorial, we will walk you through the process of acquiring browser details in Laravel 10, such as browser name, browser version, and so on, allowing you to capture and use information about the user’s web browser.
By the end of this class, you’ll have the skills and expertise to efficiently extract browser details in your Laravel 10 applications, allowing you to get insights into user behaviour and improve the user experience using browser-specific information.
Read More: Laravel 10 How to Set Application Timezone Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Installation of Browser Agent Package in Laravel
A PHP package desktop/mobile user agent parser with support for Laravel, based on Mobile Detect with desktop support and additional functionality.
Open project terminal and run this command to install it,
composer require jenssegers/agent
It will install above package into your laravel application,
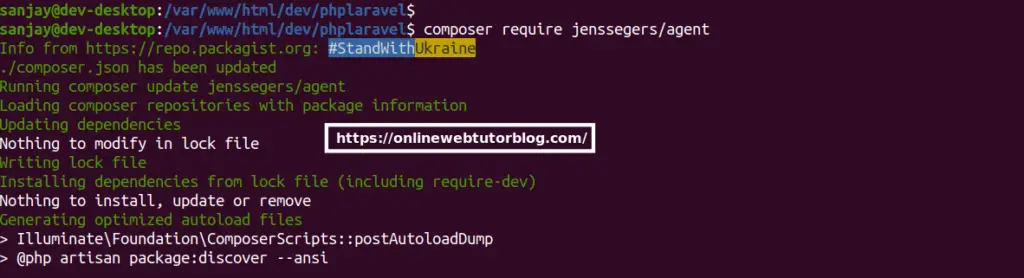
Once, you install this package successfully. You will get Agent facade in your application.
Next,
Let’s use this package to detect device details like browser name, browser version, etc information.
How To Use Browser Agent in Laravel
Load package in class files,
use Jenssegers\Agent\Facades\Agent;
Example,
$browser = Agent::browser();
$version = Agent::version($browser);
Example of getting Information by Agent Package
Open project terminal and run this command to create controller class,
php artisan make:controller DemoController
It will create a file DemoController.php inside /app/Http/Controllers folder.
Read More: Laravel 10 Convert Number To Words Example Tutorial
Example #1: Get Browser Name and Version in Laravel
Open this controller file and write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Jenssegers\Agent\Facades\Agent; class DemoController extends Controller { public function index() { $browser = Agent::browser(); $version = Agent::version($browser); dd($browser, $version); } }
Output
"Chrome"
"116.0.0.0"
Example #2: Get Device Name in Laravel
To get about device you will use device() method of Agent.
$device = Agent::device();
Output
"WebKit"
Example #3: Get Platform Name in Laravel
To get platform detail, you will use platform() method.
$platform = Agent::platform();
Output
"Linux"
Example #4: Check Device is Desktop, Tablet or Phone in Laravel
To check device type,
For mobile,
Agent::isMobile()
For desktop,
Agent::isDesktop()
For tablet,
Agent::isTablet()
For phone tabs,
Agent::isPhone()
Example
if (Agent::isMobile()) {
$result = 'Yes, This is Mobile.';
} else if (Agent::isDesktop()) {
$result = 'Yes, This is Desktop.';
} else if (Agent::isTablet()) {
$result = 'Yes, This is Desktop.';
} else if (Agent::isPhone()) {
$result = 'Yes, This is Phone.';
}
dd($result);
Read More: Laravel 10 Add Custom Search Filter To YajraBox Datatable
Output,
Yes, This is Desktop.
Example #5: User is Robot or Not in Laravel
To check system’s user is robot controlled or by user,
Agent::isRobot()
That’s it.
We hope this article helped you to learn about Laravel 10 How To get Browser Details Example Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.