Inside this article we will see the concept i.e Laravel 10 Scout How To Add Full Text Search in Table. Article contains the classified information i.e Full-text search implementation in Laravel 10 with Scout.
Laravel 10 Scout is a full-text search package for Laravel.
Scout makes it easy to implement powerful search functionality into Laravel applications by providing a simple and intuitive API for integrating with various search engines such as Algolia, Elasticsearch, and Meilisearch.
With Laravel 10 Scout, developers can quickly and easily add full-text search capabilities to their applications, allowing users to search through large datasets with ease.
Read More: Laravel 10 YajraBox Server Side Datatable Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Laravel Migration
We will create a students table using migration and then we seed test data inside it.
Open project into terminal and run this command to create migration file.
$ php artisan make:migration create_students_table
It will create 2023_03_17_031027_create_students_table.php file inside /database/migrations folder. Open migration file and write this following code into it.
The code is all about for the schema of students table.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('students', function (Blueprint $table) { $table->id(); $table->string("name", 120); $table->string("email", 50)->nullable(); $table->string("mobile", 50)->nullable(); $table->integer("age"); $table->enum("gender", ["male", "female", "others"]); $table->text("address_info"); $table->timestamp("created_at")->useCurrent(); $table->timestamp("updated_at")->useCurrent(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('students'); } };
Run Migration
Back to terminal and run this command.
$ php artisan migrate
It will create students table inside database.
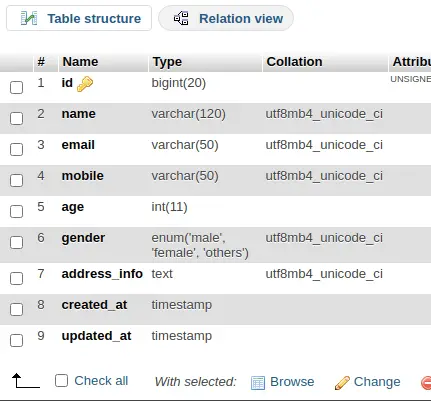
Read More: Laravel 10 Step By Step Stub Customization Tutorial
Create Model
Back to project terminal and run this command to create model.
$ php artisan make:model Student
It will create Student.php file inside /app/Models folder. This file will help when we generate data using factory and insert into table.
Next,
Create Data Factory
Copy this command and run into terminal to create a factory file.
$ php artisan make:factory StudentFactory
It will create a file StudentFactory.php inside /database/factories folder.
Open file and write this following code into it.
<?php namespace Database\Factories; use Illuminate\Database\Eloquent\Factories\Factory; /** * @extends \Illuminate\Database\Eloquent\Factories\Factory<\App\Models\Student> */ class StudentFactory extends Factory { /** * Define the model's default state. * * @return array<string, mixed> */ public function definition() { return [ "name" => $this->faker->name(), "email" => $this->faker->safeEmail, "mobile" => $this->faker->phoneNumber, "age" => $this->faker->numberBetween(25, 45), "gender" => $this->faker->randomElement([ "male", "female", "others" ]), "address_info" => $this->faker->address ]; } }
How To Call Model Factory
Open DatabaseSeeder.php from /database/seeders folder. Load model and factory.
<?php namespace Database\Seeders; use Illuminate\Database\Console\Seeds\WithoutModelEvents; use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { /** * Seed the application's database. * * @return void */ public function run() { \App\Models\Student::factory(100)->create(); } }
Concept
\App\Models\Student::factory(100)->create();
It will generate 100 fake rows of student using StudentFactory.php file and save into students table.
Read More: How To Work with Laravel 10 Model Events Tutorial
Run Data Seeder
Back to project terminal and run this command to run factory to seed fake data.
$ php artisan db:seed
It will generate and save fake data into students table.
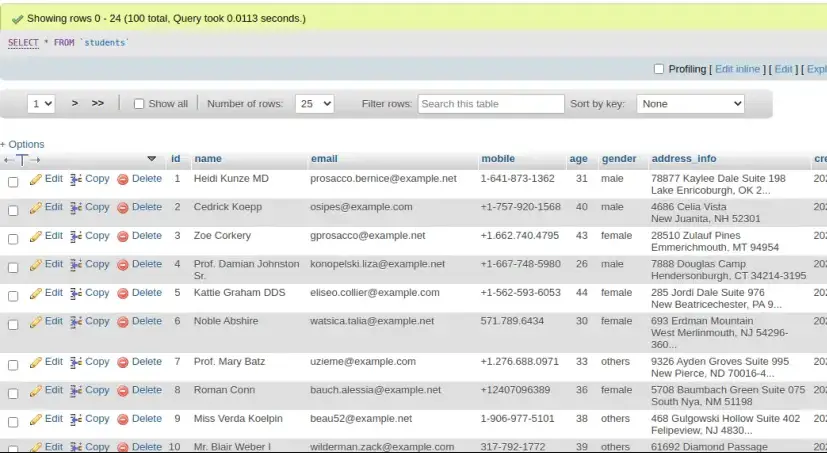
Install Laravel Scout (a Composer Package)
To install scout, we need to run a composer command. Open up the project terminal and type this command and execute.
Package Installation
$ composer require laravel/scout
While installation of this package, you will get such type of screen over terminal.
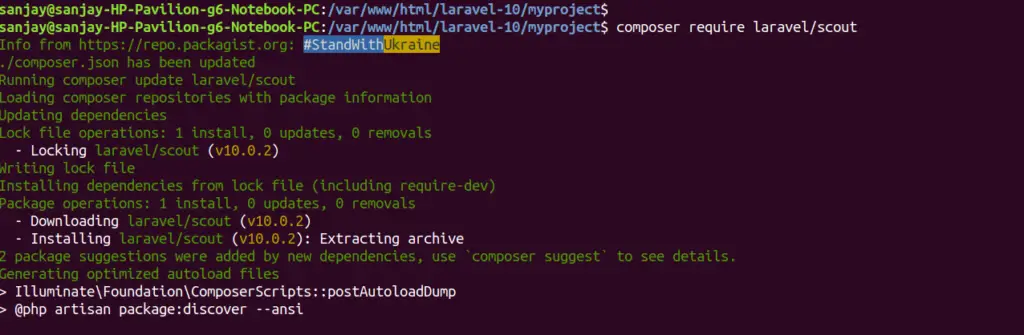
After installing Scout, you must publish the Scout configuration file using the vendor:publish Artisan command. This command will publish the scout.php configuration file to your application’s config directory.
Package Configuration Publish
$ php artisan vendor:publish --provider="Laravel\Scout\ScoutServiceProvider"
Add to .env
Add this line to .env file.
SCOUT_DRIVER=database
Update Model File
Open Student.php from /app/Models folder. Add these following settings for scout search.
Import scout package
use Laravel\Scout\Searchable;
Then,
use Searchable;
Add searchable keys settings,
public function toSearchableArray()
{
return [
'name' => $this->name,
'email' => $this->email,
'gender' => $this->gender,
];
}
This method will tell the search process that searching will be from the value of name, email and gender. If you add more keys into it then search process will be more flexible.
Here, is the complete code of Student.php model file –
<?php namespace App\Models; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; use Laravel\Scout\Searchable; class Student extends Model { use HasFactory, Searchable; public $timestamps = false; public function toSearchableArray() { return [ 'name' => $this->name, 'email' => $this->email, 'gender' => $this->gender, ]; } }
Create Controller
Open project into terminal and run this command into it.
$ php artisan make:controller StudentController
It will create StudentController.php file inside /app/Http/Controllers folder. Open controller file and write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Student; class StudentController extends Controller { public function index(Request $request) { if ($request->filled('search')) { $students = Student::search($request->search)->get(); // search by value } else { $students = Student::get()->take('10'); // list 10 rows } return view('students', compact('students')); } }
Create Template
Create a fie students.blade.php inside /resources/views folder.
Open students.blade.php and write this content into it.
<html> <head> <title>Laravel 10 Scout How To Add Full Text Search</title> <link href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/5.0.1/css/bootstrap.min.css" rel="stylesheet"> </head> <body> <div class="container"> <h3 class="mt-5 mb-5 text-center">Laravel 10 Scout How To Add Full Text Search</h3> <form method="GET" class="mb-5"> <div class="input-group mb-3"> <input type="text" name="search" value="{{ request()->get('search') }}" class="form-control" placeholder="Search..." aria-label="Search" aria-describedby="button-addon2"> <button class="btn btn-primary" type="submit" id="button-addon2">Search</button> </div> </form> <table class="table table-bordered data-table"> <thead> <tr> <th>ID</th> <th>Name</th> <th>Email</th> <th>Gender</th> <th>Mobile</th> </tr> </thead> <tbody> @foreach ($students as $student) <tr> <td>{{ $student->id }}</td> <td>{{ $student->name }}</td> <td>{{ $student->email }}</td> <td>{{ ucfirst($student->gender) }}</td> <td>{{ $student->mobile }}</td> </tr> @endforeach </tbody> </table> </div> </body> </html>
Add Route
Open web.php file from /routes folder. Add this route and code into it.
//... use App\Http\Controllers\StudentController; //... Route::get('students', [StudentController::class, 'index']);
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/students
List all rows with search textbox
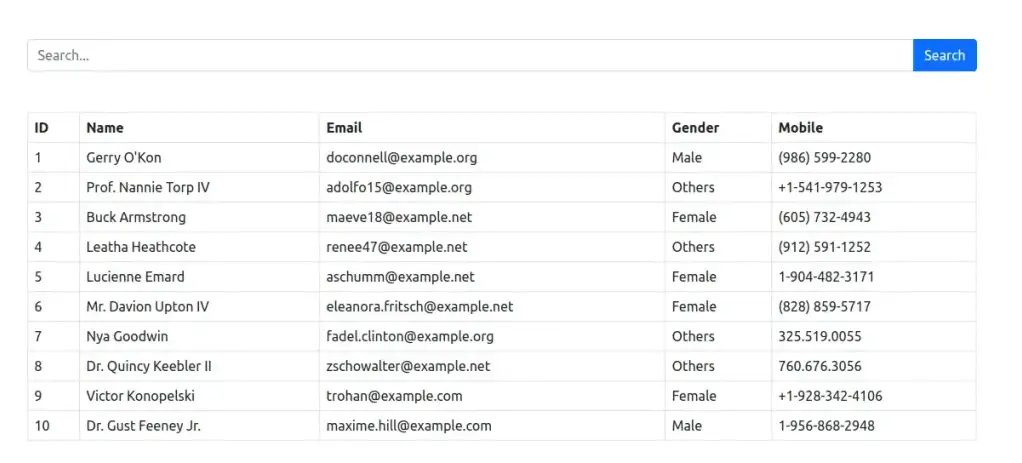
List rows by putting a search value i.e ‘Ny‘
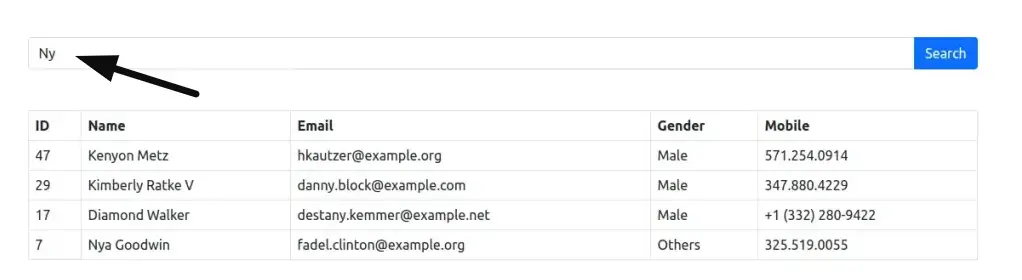
We hope this article helped you to learn about Laravel 10 Scout How To Add Full Text Search in Table in a very detailed way.
Read More: Laravel 10 How To Connect with Multiple Database Tutorial
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.