Inside this article we will see the concept of Laravel 9 How to seed database using seeder file. Article contains classified information about database seeding concept. If we have a large scale application in laravel and for testing we want huge amount of data then this concept is very useful to seed test data into table.
In Laravel there are several ways by which we can seed fake data to database for testing purpose. Here, we will see how to seed a simple table using a set of test data in a very simple way.
Additionally, we can use the concept of Faker library to generate data in bulk instead of creating a limited set of fake data.
Learn More –
- Laravel 9 Markdown Mailable Email Template Components
- Laravel 9 One to Many Eloquent Relationship Tutorial
- Laravel 9 Call MySQL Stored Procedure Tutorial
- Laravel 9 FullCalendar Ajax CRUD Tutorial Example
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Migration
We will create a events table using migration and then we seed test data inside it.
Open project into terminal and run this command to create migration file.
$ php artisan make:migration create_events_table
It will create 2022_04_17_031027_create_events_table.php file inside /database/migrations folder. Open migration file and write this following code into it.
The code is all about for the schema of events table.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('events', function (Blueprint $table) { $table->id(); $table->string('title', 50); $table->date('start'); $table->date('end'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('events'); } };
Run Migration
Back to terminal and run this command.
$ php artisan migrate
It will create events table inside database.
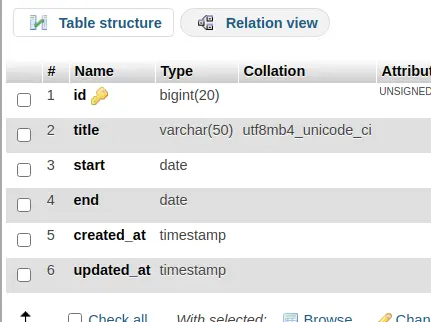
Create Data Seeder
Next,
Copy this command and run into terminal to create a data seeder for events table.
$ php artisan make:seeder FakeEventSeeder
It will create a file FakeEventSeeder.php inside /database/seeders folder. Open file and write this following code into it.
<?php namespace Database\Seeders; use Illuminate\Database\Console\Seeds\WithoutModelEvents; use Illuminate\Database\Seeder; use App\Models\Event; class FakeEventSeeder extends Seeder { /** * Run the database seeds. * * @return void */ public function run() { $data = [ ['title' => 'Fake Event-1', 'start' => '2021-08-11', 'end' => '2021-08-12'], ['title' => 'Fake Event-2', 'start' => '2021-08-11', 'end' => '2021-08-13'], ['title' => 'Fake Event-3', 'start' => '2021-08-14', 'end' => '2021-08-14'], ['title' => 'Fake Event-3', 'start' => '2021-08-17', 'end' => '2021-08-17'], ]; foreach ($data as $key => $value) { Event::create($value); } } }
Run Seeder To Database
We have multiple options available to execute a seeder file to database.
We will discuss about these two –
- Use DatabaseSeeder to run seeder file.
- Without DatabaseSeeder to run seeder.
Use DatabaseSeeder to run seeder file
We load seeder class into DatabaseSeeder.php which should be inside /database/seeders folder.
Open file and load FakeEventSeeder.php into it.
<?php namespace Database\Seeders; use Illuminate\Database\Console\Seeds\WithoutModelEvents; use Illuminate\Database\Seeder; use Database\Seeders\FakeEventSeeder; class DatabaseSeeder extends Seeder { /** * Seed the application's database. * * @return void */ public function run() { $this->call(FakeEventSeeder::class); // Run Seeder Class } }
Run Seeder
Back to terminal and run this command.
$ php artisan db:seed
Without DatabaseSeeder to run seeder
Here, we run FakeEventSeeder.php file directly instead of loading it to DatabaseSeeder.php file.
$ php artisan db:seed --class=FakeEventSeeder
We specify the class flag with seeder class name. It runs specified seeder class name.
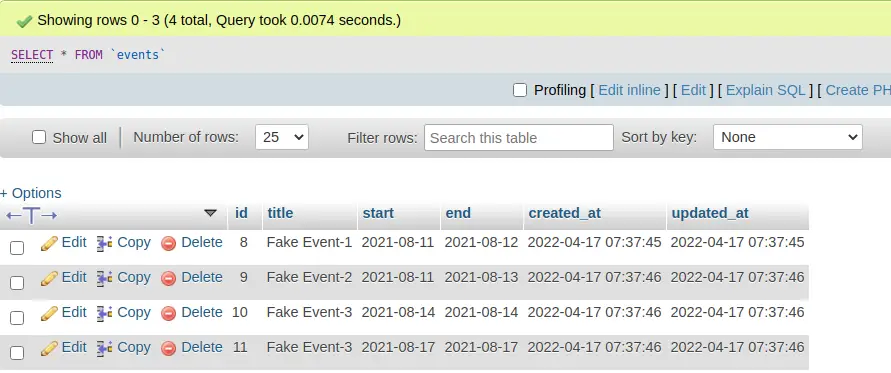
We hope this article helped you to learn about Laravel 9 How To Seed Database Using Seeder File Tutorial in a very detailed way.
[do_widget “buy me a coffee”]
Online Web Tutor invites you to try Skillshare free for 1 month! Learn CakePHP 4, Laravel APIs Development, CodeIgniter 4, Node Js, etc into a depth level. Master the Coding Skills to Become an Expert in Web Development. So, Search your favourite course and enroll now. Click here to join.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.