Inside this article we will see the concept of Laravel 9 HTTP cURL DELETE Request. This tutorial will be easy to understand and implement. It will give you the complete idea of Http curl request integration in laravel 9.
In laravel we will use Http facade to work with curl request and it’s methods. Curl request is very useful to send data in various request types. How to send a delete request to delete a resource we also see in this article.
We will see cURL request with DELETE using HTTP and GuzzleHTTP. To understand cURL we have taken fake data api urls from here Fake API URL.
cURL is a tool to transfer data from or to a server, using one of the supported protocols (HTTP, HTTPS, FTP, FTPS, GOPHER, DICT, TELNET, LDAP or FILE)
Learn More –
- Laravel 9 How To Work with Ajax Post Request
- Laravel 9 How To Store Data in Cache Tutorial
- Laravel 9 Http cURL Get Request Tutorial
- Laravel 9 HTTP cURL POST Request with Headers Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Controller & Add cURL Concept
Next,
We need to create a controller file.
$ php artisan make:controller SiteController
It will create a file SiteController.php inside /app/Http/Controllers folder.
Open SiteController.php and write this complete code into it.
cURL Request with DELETE Using HTTP –
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Http; class SiteController extends Controller { public function index() { $deleteId = 1; // URL $apiURL = 'https://api.onlinewebtutorblog.com/employees/'.$deleteId; $response = Http::delete($apiURL); $statusCode = $response->status(); $responseBody = json_decode($response->getBody(), true); echo "Status code: ". $statusCode; // status code dd($responseBody); // body response } }
In the above example we have used Http facade.
cURL Request with DELETE Using GuzzleHttp –
To work with GuzzleHttp, we need to install guzzle package into laravel application. Here is the given command to install guzzlehttp package into application –
Install guzzlehttp/guzzle Package
Open project into terminal run this command.
$ composer require guzzlehttp/guzzle
This package will install guzzlehttp and also updates composer.json file at root.
"require": {
//...
"guzzlehttp/guzzle": "^7.2",
//...
},
Let’s use GuzzleHttp package work with Http request.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class SiteController extends Controller { public function index() { $deleteId = 1; // URL $apiURL = 'https://api.onlinewebtutorblog.com/employees/' . $deleteId; $client = new \GuzzleHttp\Client(); $response = $client->request("DELETE", $apiURL); $statusCode = $response->getStatusCode(); $responseBody = json_decode($response->getBody(), true); echo "Status code: ". $statusCode; // status code dd($responseBody); // body response } }
Output
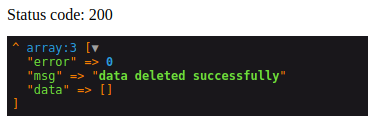
We hope this article helped you to learn Laravel 9 Http cURL DELETE Request Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.