Inside this article we will see the concept of Laravel 9 Http cURL Get Request Tutorial. We will see by both using Http GET and GuzzleHttp of laravel to process JSON data.
The cURL stands for ‘Client for URLs‘, originally with URL spelled in uppercase to make it obvious that it deals with URLs. Since curl uses libcurl, it supports a range of common internal protocols, currently including HTTP, HTTPS, FTP, FTPS, GOPHER, TELNET, DICT, and FILE.
This article is very easy to learn and implement in your development. We will look at example of laravel curl get request. Concept is useful when you work with APIs and use HTTP GET request. Here, we will consider a fake api url and try to fetch data from it.
Learn More –
- Laravel 9 How To Send Email To Multiple Users Tutorial
- Laravel 9 How To Store Data in Cache Tutorial
- Laravel 9 How To Work with Ajax Post Request
- Laravel 9 HTTP cURL DELETE Request Tutorial
Let’s get started.
Laravel Installation
Open terminal and run this command to create a laravel project.
composer create-project laravel/laravel myblog
It will create a project folder with name myblog inside your local system.
To start the development server of laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Install guzzlehttp/guzzle Package
Open project into terminal run this command.
$ composer require guzzlehttp/guzzle
This package will install guzzlehttp and also updates composer.json file at root.
"require": {
//...
"guzzlehttp/guzzle": "^7.2",
//...
},
Create Controller & Add HTTP cURL
Open project into terminal and run this artisan command.
$ php artisan make:controller SiteController
It will create SiteController.php inside /app/Http/Controllers folder.
Open SiteController.php and write this code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Http; class SiteController extends Controller { /** * Using Http * * @return response() */ public function index() { $apiURL = 'https://api.onlinewebtutorblog.com/employees'; $response = Http::get($apiURL); $statusCode = $response->status(); $responseBody = json_decode($response->getBody(), true); dd($responseBody); } /** * Using GuzzleHttp * * @return response() */ public function posts() { $apiURL = 'https://api.onlinewebtutorblog.com/employees'; $client = new \GuzzleHttp\Client(); $response = $client->request('GET', $apiURL); $statusCode = $response->getStatusCode(); $responseBody = json_decode($response->getBody(), true); dd($responseBody); } }
This controller includes two methods to process HTTP cURL request – Using HTTP and using GuzzleHttp. You can opt any option to use it in your development.
Add Route
Open web.php file from /routes folder. Add these routes into it.
//... use App\Http\Controllers\SiteController; Route::get("posts", [SiteController::class, "index"]); Route::get("all-posts", [SiteController::class, "posts"]);
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Example URL #1 – http://127.0.0.1:8000/posts
Example URL #2 – http://127.0.0.1:8000/all-posts
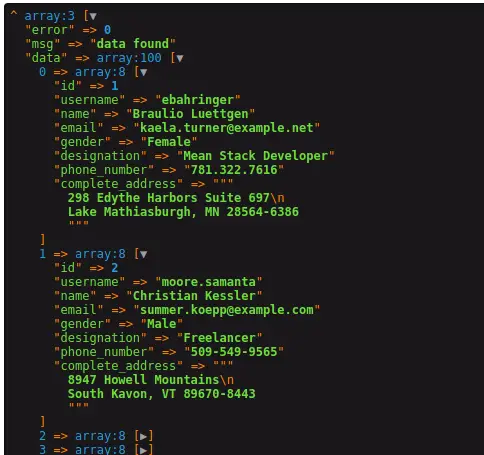
We hope this article helped you to learn Laravel 9 Http cURL Get Request Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.