In this article we will discuss that how we interact with Ratchet socket using PHP webhooks.
Already from last article we understood about this,
Ratchet Connectivity Using Javascript Webhook
By using javascript you can connect to Ratchet socket by this code …
<script> var conn = new WebSocket('ws://localhost:8080'); conn.onopen = function(e) { console.log("Connection established!"); }; conn.onmessage = function(e) { console.log(e.data); }; </script>
and also to send messages to it,
conn.send("Your messages to server");
Ratchet Connectivity Using PHP Webhook
By using PHP you can connect to Ratchet socket by these steps.
Create a separate client setup for your application.
Step #1
Install this composer package into your client’s setup.
$ composer require ratchet/pawl
Your composer.json file will look like this:
{
"require": {
"ratchet/pawl": "^0.4.1"
}
}
Package installation will also setup a /vendor folder inside your application.
Step #2
Create a file index.php inside client’s setup.
Open index.php and write this following code into it.
<?php require __DIR__ . '/vendor/autoload.php'; \Ratchet\Client\connect('ws://localhost:8080')->then(function($conn) { $conn->send('Hellooo, Online Web Tutor chatbot here...'); }, function ($e) { echo "Could not connect: {$e->getMessage()}\n"; });
Whenever you call this file, automatically it will send message to socket.
Output

Next,
In case if you wish to connect Ratchet socket with your API calls also you can do that.
Here, is the updated index.php which will accept data from API call and send to socket.
<?php require __DIR__ . '/vendor/autoload.php'; $data = file_get_contents("php://input"); $decodedData = json_decode($data, true); if (isset($decodedData) && !empty($decodedData)) { \Ratchet\Client\connect ('ws://localhost:8080')->then(function ($conn) { global $decodedData; $conn->send(json_encode($decodedData)); $conn->close(); }, function ($e) { echo "Could not connect: {$e->getMessage()}\n"; }); } else { echo "Data is required"; exit; }
Calling this API from Postman,
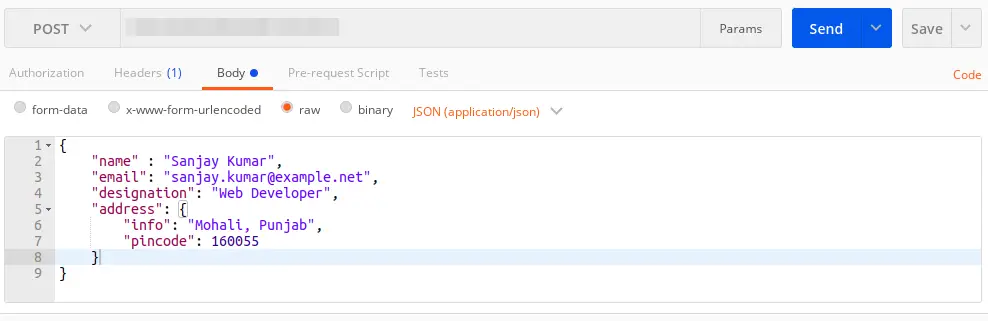
Server output on console

Successfully, we done it.
You can send your API data to your socket to read messages.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.