In this interesting article you will learn to run your first program in PHP WebSocket.
We are assuming everything at your end is set from last two articles. Now, we will create a very basic PHP WebSocket Programming in a very easier way.
Create a folder with name say basic into your project folder. Inside this folder create two files: client.php and server.php.
- client.php: The file from where user can send their chat messages.
- server.php: The file which listen user’s messages.
Code Settings for “server.php”
Open server.php and write this following code into it.
<?php $host = "127.0.0.1"; // hostname $port = 8085; //port // Create PHP Socket $socket = socket_create(AF_INET, SOCK_STREAM, 0) or die("Not connected"); // Bind socket with host and port $result = socket_bind($socket, $host, $port) or die("Not bind"); // Listen socket from it's address $result = socket_listen($socket, 3) or die("Not listen"); // Check messages from client do { $accept = socket_accept($socket) or die("Not accept"); // Read socket message $msg = socket_read($accept, 1024) or die("Not read"); $msg = trim($msg); // Print into cosole echo $msg . "\n"; } while (true); // Socket close socket_close($socket);
You can see above code used several PHP WebSocket functions. This is all about the code which listen to socket for user’s messages.
Next, let’s prepare for client.php
Code Settings for “client.php”
Open client.php and write this following code into it.
<?php $host = "127.0.0.1"; // hostname $port = 8085; //port // Create PHP Socket $socket = socket_create(AF_INET, SOCK_STREAM, 0) or die("Not connected"); // Connect socket with host and port $result = socket_connect($socket, $host, $port) or die("Server not connected"); $msg = "Hello from client..."; if (isset($_POST['submit'])) { // form submission $msg = $_POST['message']; } // Write client message to socket socket_write($socket, $msg, strlen($msg)); // Socket close socket_close($socket); ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> Enter message:<br/> <input type="text" name="message"> <button name="submit">Submit</button> </form>
Now, everything is done for creating Uni-direction PHP WebSocket program.
Application Testing
Open terminal / command prompt.
Run this command to start server.php
$ php -f server.php
Back to browser and run client.php, it will display you as:
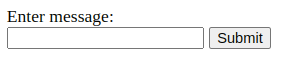
Next, whenever you type any message and click on submit it will pass message to socket. Server then, listen and read message print to console.
It will show you as:

Successfully, you have created your first program into PHP WebSocket.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.