As we all know in most web application either in CakePHP 4 or any of the PHP Framework, we sometimes need to integrate payment gateway. Inside this article, we will see Stripe payment Integration in CakePHP 4. Stripe payment system settings in CakePHP 4. Easy and in complete detailed concept.
Let’s get started.
CakePHP 4 Installation
To create a CakePHP project, run this command into your shell or terminal. Make sure composer should be installed in your system.
$ composer create-project --prefer-dist cakephp/app:~4.0 mycakephp
Above command will creates a project with the name called mycakephp.
Stripe PHP Package Installation
We will install stripe package via composer into CakePHP 4 setup. Open CakePHP 4 project in terminal and hit this composer command.
$ composer require stripe/stripe-php
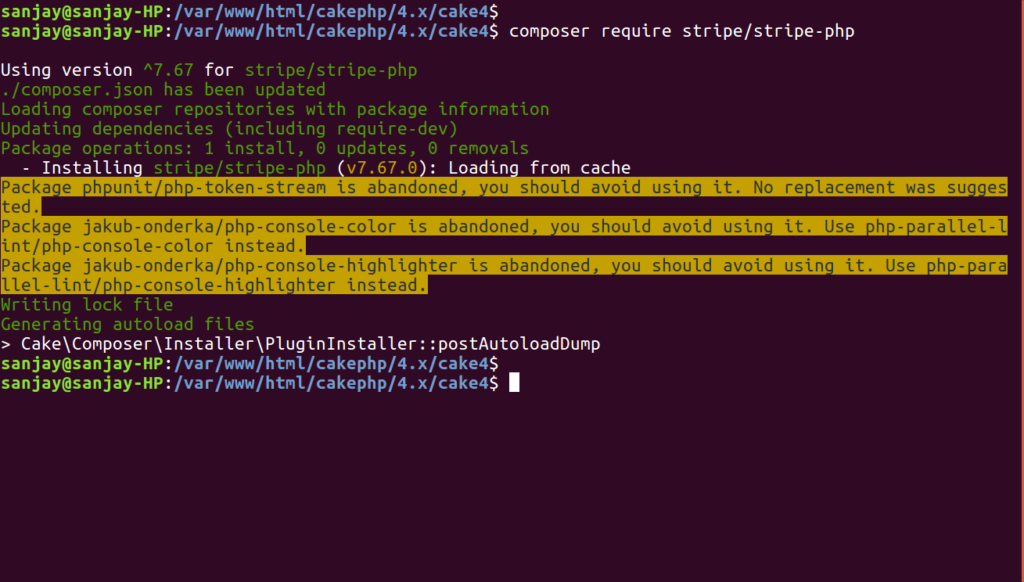
Set Stripe API Key and SECRET
Open up /config/paths.php file of CakePHP 4 application. Into the file, set few constants stripe api key, secret & vendor folder.
defined("VENDOR_PATH") || define('VENDOR_PATH', ROOT . DS . 'vendor'); defined("STRIPE_KEY") || define('STRIPE_KEY', "pk_test_MaQhP2b8XqG9R7PTL6VUmipt"); defined("STRIPE_SECRET") || define('STRIPE_SECRET', "sk_test_MtVxg0tJfCGJXQY8xZlu69Ie");
This is our stripe sandbox detail, you can replace it with your own. If you are wondering to find stripe test account keys. Follow these steps –
- Login to https://dashboard.stripe.com/
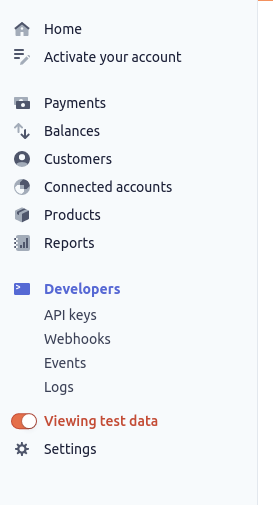
- You need to enable Viewing test data to use Test account details
- Go to Developer >> Click on API keys
Create Routes
Next, we need to set CakePHP 4 stripe payment gateway application routes. To configure routes, open up /config/routes.php file.
$routes->scope('/', function (RouteBuilder $builder) { //... other routes $builder->connect('/stripe', ['controller' => 'Stripes', 'action' => 'stripe']); $builder->connect('/payment', ['controller' => 'Stripes', 'action' => 'payment']); $builder->fallbacks(); });
Create Controller File
Create application controller in setup. To create controller file, go to /src/Controller/StripesController.php and create this file. Open StripesController.php and paste the given code.
<?php namespace App\Controller; use Stripe; class StripesController extends AppController { public function stripe() { $this->set("title", "Stripe Payment Gateway Integration"); } public function payment() { require_once VENDOR_PATH. '/stripe/stripe-php/init.php'; Stripe\Stripe::setApiKey(STRIPE_SECRET); $stripe = Stripe\Charge::create ([ "amount" => 70 * 100, "currency" => "usd", "source" => $_REQUEST["stripeToken"], "description" => "Test payment via Stripe From onlinewebtutorblog.com" ]); // after successfull payment, you can store payment related information into your database $this->Flash->success(__('Payment done successfully')); return $this->redirect(['action' => 'stripe']); } }
Create Template File
Let’s create template file for setting the layout of payment details for user. Firstly we need to create a folder in /templates with the name as Stripes. Create a file stripe.php inside /templates/Stripes/stripe.php. Open file and paste the given code
<!DOCTYPE html> <html> <head> <title>CakePHP 4 - Stripe Payment Gateway Integration - onlinewebtutorblog.com</title> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/twitter-bootstrap/3.3.7/css/bootstrap.min.css" /> <script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <style type="text/css"> .panel-title { display: inline; font-weight: bold; } .display-table { display: table; } .display-tr { display: table-row; } .display-td { display: table-cell; vertical-align: middle; width: 61%; } </style> </head> <body> <div class="container"> <h3 style="text-align: center;">CakePHP 4 - Stripe Payment Gateway Integration - onlinewebtutorblog.com</h3><br/> <?= $this->Flash->render() ?> <div class="row"> <div class="col-md-6 col-md-offset-3"> <div class="panel panel-default credit-card-box"> <div class="panel-body"> <?= $this->Form->create(null, [ "action" => $this->Url->build("/payment", ["fullBase" => false]), "method" => "post", "class" => "require-validation", "data-cc-on-file" => "false", "data-stripe-publishable-key" => STRIPE_KEY, "id" => "payment-form" ]) ?> <div class='form-row row'> <div class='col-xs-12 form-group required'> <label class='control-label'>Name on Card</label> <input class='form-control' size='4' type='text'> </div> </div> <div class='form-row row'> <div class='col-xs-12 form-group card required'> <label class='control-label'>Card Number</label> <input autocomplete='off' class='form-control card-number' size='20' type='text'> </div> </div> <div class='form-row row'> <div class='col-xs-12 col-md-4 form-group cvc required'> <label class='control-label'>CVC</label> <input autocomplete='off' class='form-control card-cvc' placeholder='ex. 311' size='4' type='text'> </div> <div class='col-xs-12 col-md-4 form-group expiration required'> <label class='control-label'>Expiration Month</label> <input class='form-control card-expiry-month' placeholder='MM' size='2' type='text'> </div> <div class='col-xs-12 col-md-4 form-group expiration required'> <label class='control-label'>Expiration Year</label> <input class='form-control card-expiry-year' placeholder='YYYY' size='4' type='text'> </div> </div> <div class='form-row row'> <div class='col-md-12 error form-group hide'> <div class='alert-danger alert'>Please correct the errors and try again.</div> </div> </div> <div class="row"> <div class="col-xs-12"> <button class="btn btn-primary btn-lg btn-block" type="submit">Pay Now ($70)</button> </div> </div> <?= $this->Form->end() ?> </div> </div> </div> </div> </div> </body> <script type="text/javascript" src="https://js.stripe.com/v2/"></script> <script type="text/javascript"> $(function() { var $form = $(".require-validation"); $('form.require-validation').bind('submit', function(e) { var $form = $(".require-validation"), inputSelector = ['input[type=email]', 'input[type=password]', 'input[type=text]', 'input[type=file]', 'textarea'].join(', '), $inputs = $form.find('.required').find(inputSelector), $errorMessage = $form.find('div.error'), valid = true; $errorMessage.addClass('hide'); $('.has-error').removeClass('has-error'); $inputs.each(function(i, el) { var $input = $(el); if ($input.val() === '') { $input.parent().addClass('has-error'); $errorMessage.removeClass('hide'); e.preventDefault(); } }); if (!$form.data('cc-on-file')) { e.preventDefault(); Stripe.setPublishableKey($form.data('stripe-publishable-key')); Stripe.createToken({ number: $('.card-number').val(), cvc: $('.card-cvc').val(), exp_month: $('.card-expiry-month').val(), exp_year: $('.card-expiry-year').val() }, stripeResponseHandler); } }); function stripeResponseHandler(status, response) { if (response.error) { $('.error') .removeClass('hide') .find('.alert') .text(response.error.message); } else { /* token contains id, last4, and card type */ var token = response['id']; $form.find('input[type=text]').empty(); $form.append("<input type='hidden' name='stripeToken' value='" + token + "'/>"); $form.get(0).submit(); } } }); </script> </html>
Application Testing
Now, here you can test with these given test card details.
Open project in terminal and start development server
$ bin/cake server -p 8765
This is the Form when you open the route http://localhost:8765/stripe
Name: Sample Number: 4242 4242 4242 4242 CSV: 123 Expiration Month: 04 Expiration Year: 2024
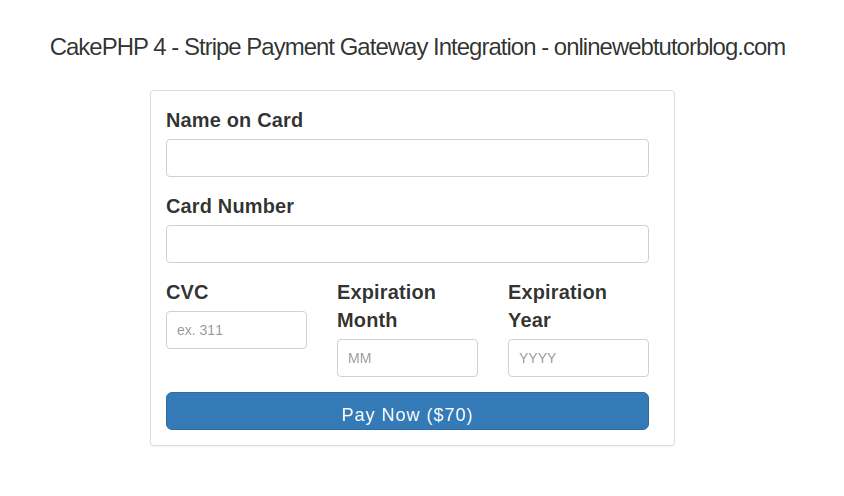

We hope this article helped you to learn about Stripe Payment Gateway Integration in CakePHP 4 in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.
How we can store customer name (cardholder name) in the stripe.
Hi, you need to use Stripe apis to create customer and store.