Inside this article we will see the concept i.e how to upload image with validation in laravel 8. This article will be very interesting to see and also very easy to implement in your laravel 8 application.
You will learn Upload Image in laravel 8. Very few simple steps we need to follow to do it application setup. If you are looking for an article which gives the understanding of Image upload in laravel 8 then you are at right place to learn.
Step by step upload Image with validation in laravel 8 we will see in detail.
Learn More –
- Laravel 8 Database Seeder with Sample Data Insertion
- Laravel 8 Database Seeding from CSV File Tutorial
- Laravel 8 Database Seeding from JSON File Tutorial
- How To Get Path From Laravel 8 Application Root
Let’s get started.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create images Folder
Create a folder named as “images” inside /public folder. Make sure folder should have all permissions like read and write etc.
When we upload images from form, it will be saved inside this images folder.
Create Controller
Open project into terminal and run this artisan command to create controller.
$ php artisan make:controller UploadImageController
It will creates UploadImageController.php inside /app/Http/Controllers folder.
Open UploadImageController.php and write this complete code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; class UploadImageController extends Controller { public function imageUpload() { return view('image-upload'); } public function imageUploadPost(Request $request) { $request->validate([ 'image' => 'required|image|mimes:jpeg,png,jpg,gif,svg|max:2048', ]); $imageName = time() . '.' . $request->image->extension(); $request->image->move(public_path('images'), $imageName); return back() ->with('success', 'Successfully, you have uploaded image') ->with('image', $imageName); } }
In this controller file we have all the logic to show image upload form as well as upload image concept.
images is the name of folder.
Next,
We need blade template file.
Create Blade Template Layout
Create a blade template file with name image-upload.blade.php into /resources/views folder.
Open image-upload.blade.php and write this complete code into it.
<!DOCTYPE html> <html lang="en"> <head> <title>How To Upload Image in Laravel 8 Tutorial</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> </head> <body> <div class="container"> <h4>How To Upload Image in Laravel 8 Tutorial</h4> <div class="panel panel-primary"> <div class="panel-heading">How To Upload Image in Laravel 8 Tutorial</div> <div class="panel-body"> @if ($message = Session::get('success')) <div class="alert alert-success alert-block"> <button type="button" class="close" data-dismiss="alert">×</button> <strong>{{ $message }}</strong> </div> <img src="images/{{ Session::get('image') }}" style="height: 100px;width:100px;"> @endif @if (count($errors) > 0) <div class="alert alert-danger"> <strong>Whoops!</strong> There were some problems while uploading image. <ul> @foreach ($errors->all() as $error) <li>{{ $error }}</li> @endforeach </ul> </div> @endif <form action="{{ route('image.upload.post') }}" method="post" enctype="multipart/form-data"> @csrf <div class="form-group"> <label for="email">Upload Image:</label> <input type="file" name="image" class="form-control"> </div> <button type="submit" class="btn btn-success">Submit</button> </form> </div> </div> </div> </body> </html>
Add Routes
Open web.php from /routes folder and add these routes into it.
//... use App\Http\Controllers\UploadImageController; Route::get("image-upload", [UploadImageController::class, "imageUpload"])->name('image.upload'); Route::post("image-upload", [UploadImageController::class, "imageUploadPost"])->name('image.upload.post'); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL: http://127.0.0.1:8000/image-upload
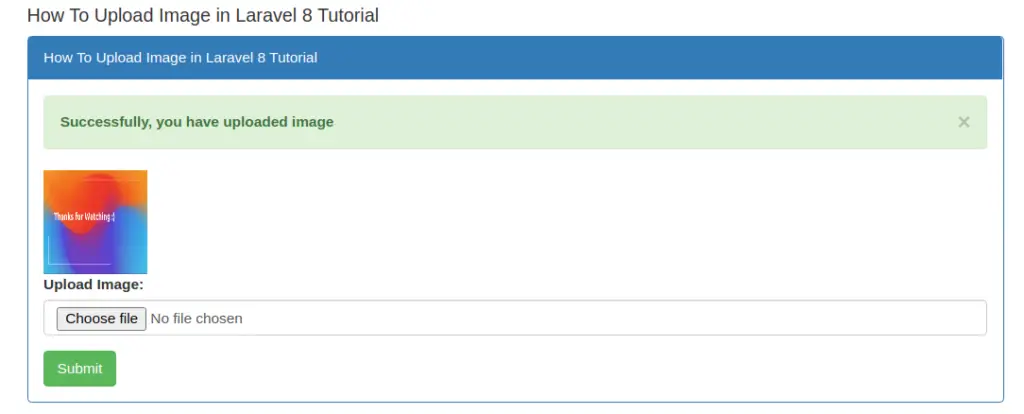
We hope this article helped you to learn How To Upload Image with Validation in Laravel 8 Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.