When we work with MySQL Queries, then definitely for some relational data we need to work with Joins. Inside this article we will see the concept of Left Join in Laravel 8.
Joins in Laravel 8 is the connection between one and more tables to get data. In MySQL we have Inner join, Left join, Right join etc.
We will see the concept of Left Join in this Laravel 8 article.
Learn Laravel 8 relationships –
This article will give you the detailed concept of about implementation of Left Join in laravel 8.
For this tutorial we will consider a employees table and a projects table.
Let’s get started.
Types of Joins available in Laravel 8
In Laravel 8 application, as per the documentation we have following types of joins available –
- Inner Join
- Left Join
- Right Join
- Cross Join
What is Left Join?
Left Join – This also works same the matched condition between two or more than two tables. But in this case we also get the rows of left table which doesn’t match with the condition with the right hand sided table. Means we get all rows of left table including values of matched with right table.
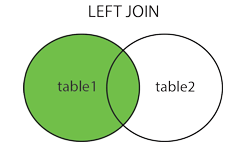
How can we use inside an application and get relational data we will see by making an application. Let’s create an application in which we use Left Join.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Create Migrations & Model
Open project into terminal and run this artisan command.
$ php artisan make:model Employee -m
It will create two files –
- Model file Employee.php at /app/Models folder.
- Migration file 2021_06_15_062204_create_employees_table.php at /database/migrations folder.
$ php artisan make:migration create_projects_table
It will create a migration file 2021_06_15_062234_create_projects_table.php at /database/migrations folder.
Open 2021_06_15_062204_create_employees_table.php and write this following code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateEmployeesTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('employees', function (Blueprint $table) { $table->increments("id"); $table->string("name", 50); $table->string("email", 50); $table->string("phone_no", 20); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('employees'); } }
Open 2021_06_15_062234_create_projects_table.php and write this following code into it.
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; class CreateProjectsTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('projects', function (Blueprint $table) { $table->id(); $table->integer('employee_id')->unsigned(); $table->string("project_name", 100); $table->foreign('employee_id') ->references('id')->on('employees') ->onDelete('cascade'); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('projects'); } }
Migrate Migrations
Back to terminal and run this command to migrate all migrations.
$ php artisan migrate
This command will migrate all.
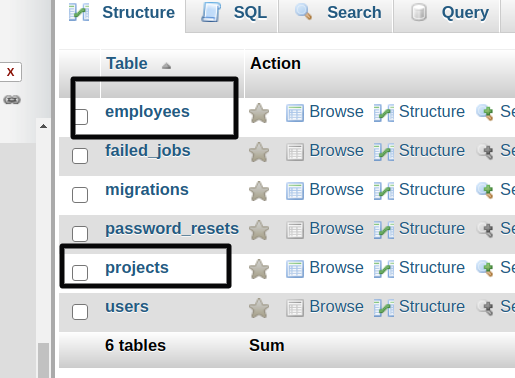
Insert Test Data For Tables
Here, we have some mysql queries which will insert test data to employees and projects table.
Copy command and run into SQL tab of mysql database.
-- -- Dumping data for table `employees` -- INSERT INTO `employees` (`id`, `name`, `email`, `phone_no`) VALUES (1, 'Sanjay Kumar', 'sanjay@gmail.com', '8527419630'), (2, 'Vijay Kumar', 'vijay@gmail.com', '9632587410'), (3, 'Ashish Kumar', 'ashish@gmail.com', '7896541356'), (4, 'Dhananjay Kumar', 'dhananjay@gmail.com', '7896585296'), (5, 'Pradeep Kumar', 'pradeep@gmail.com', '7896556565');
-- -- Dumping data for table `projects` -- INSERT INTO `projects` (`id`, `employee_id`, `project_name`) VALUES (1, 1, 'Project 4'), (2, 2, 'Project 7'), (3, 3, 'Project 50');
Create Controller
Open project into terminal and run this command.
$ php artisan make:controller SiteController
It will create SiteController.php file at /app/Http/Controllers folder.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Models\Employee; class SiteController extends Controller { public function getData() { $data = Employee::leftJoin("projects", function ($join) { $join->on("projects.employee_id", "=", "employees.id"); })->get(); //$data = Employee::leftJoin("projects", "projects.employee_id", "=", "employees.id")->get(); /* Get Last Executed Query */ // $query = Employee::leftJoin("projects", function ($join) { // $join->on("projects.employee_id", "=", "employees.id"); // })->toSql(); // echo $query; echo "<pre>"; print_r($data); } }
Left Join Logic
$data = Employee::leftJoin("projects", function ($join) { $join->on("projects.employee_id", "=", "employees.id"); })->get();
OR
$data = Employee::leftJoin("projects", "projects.employee_id", "=", "employees.id")->get();
Generated Query
select * from `employees` left join `projects` on `projects`.`employee_id` = `employees`.`id`
Add Route
Open web.php file from /routes folder.
//... use App\Http\Controllers\SiteController; Route::get("left-join", [SiteController::class, "getData"]); //...
Application Testing
Run this command into project terminal to start development server,
php artisan serve
URL – http://127.0.0.1:8000/left-join
You will get your data set at output screen.
Complete Data of Left table + Matched data with Right Table
Advanced Cases in Left Join
Assuming these cases, you can take a hint from these.
Example 1: Laravel Eloquent leftJoin() with 2 Tables
$users = User::leftJoin('posts', 'users.id', '=', 'posts.user_id') ->get(['users.*', 'posts.descrption']);
Generated Query
select `users`.*, `posts`.`descrption` from `users` left join `posts` on `users`.`id` = `posts`.`user_id`
Example 2: Laravel Eloquent leftJoin() with 3 Tables
$users = User::left
Join('posts', 'posts.user_id', '=', 'users.id') ->leftJoin
('comments', 'comments.post_id', '=', 'posts.id') ->get(['users.*', 'posts.descrption']);
Generated Query
select `users`.*, `posts`.`descrption` from `users` left join `posts` on `posts`.`user_id` = `users`.`id` left
join `comments` on `comments`.`post_id` = `posts`.`id`
Example 3: Laravel Eloquent leftJoin() with Multiple Conditions
$users = User::leftJoin('posts', 'posts.user_id', '=', 'users.id') ->where('users.status', 'active')
->where('posts.status','active')
->get(['users.*', 'posts.descrption']);
Generated Query
select `users`.*, `posts`.`descrption` from `users` left
join `posts` on `posts`.`user_id` = `users`.`id` where `users`.`status` = active and `posts`.`status` = active
We hope this article helped you to learn about MySQL Left Join in Laravel 8 Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.