Adding text to cake images is a delightful way to personalize and enhance the visual appeal of celebratory images, such as those used in cake designs for special occasions. In PHP, incorporating text onto cake images involves leveraging image manipulation libraries to overlay text seamlessly onto the image.
In this tutorial, we’ll see the comprehensive process of writing text on a cake image using PHP. This functionality empowers developers and enthusiasts to customize cake images by adding personalized messages, greetings, or names directly onto the cake design.
Read More: How To Use ChatGPT API in PHP Using cURL Tutorial
Let’s get started.
How To Write Text on Cake Image Using PHP?
Let’s create a PHP file as “text-on-image.php” and also you need a cake image where you have to write your text.
Open file and write this complete code into it,
<?php error_reporting(E_ALL); // Path to your existing image $imagePath = 'cake-image.jpg'; // Replace this with your image path // Get the image information to determine its type $image_info = getimagesize($imagePath); // Load the existing image based on its type if ($image_info['mime'] == 'image/jpeg') { $image = imagecreatefromjpeg($imagePath); } elseif ($image_info['mime'] == 'image/png') { $image = imagecreatefrompng($imagePath); } else { die('Unsupported image type.'); // Stop the script if the image type is unsupported } // Set the font color (black in this example) $textColor = imagecolorallocate($image, 255, 255, 255); // Set the text to be written $text = "Happy Birthday \nSanjay Kumar"; // Your text here // Set the font path and size $font = 'Rubik_Bubbles/RubikBubbles-Regular.ttf'; // Replace with your font file path $fontSize = 20; // Set the position to place the text (coordinates) $textX = 250; $textY = 200; $angle = -20; // Change this value to set the rotation angle // Attempt to write the text onto the image if (!file_exists($font)) { die('Font file not found.'); } // Write the text with different shades and positions to simulate 3D effect imagettftext($image, $fontSize, $angle, $textX, $textY, $textColor, $font, $text); // Check if the font file exists if (!file_exists($font)) { die('Font file not found.'); } // Check if imagettftext function is available if (!function_exists('imagettftext')) { die('GD library does not support FreeType fonts.'); } // Set the content type header to display the modified image header('Content-type: '.$image_info['mime']); // Output the modified image if ($image_info['mime'] == 'image/jpeg') { imagejpeg($image); } elseif ($image_info['mime'] == 'image/png') { imagepng($image); } // Free up memory imagedestroy($image); ?>
Code Explanation
Here’s what each part of the code does:
- Error Reporting: Enables reporting of all PHP errors for debugging purposes.
- Image Path and Type Detection: Defines the path to the existing image and retrieves its MIME type using getimagesize().
- Image Creation: Loads the existing image using imagecreatefromjpeg() or imagecreatefrompng() based on its MIME type.
- Text and Font Setup: Defines the text, font file path, font size, text color, text position, and rotation angle.
- Text Writing: Uses imagettftext() to write the text onto the image with the specified settings. It simulates a 3D effect by drawing text multiple times with slight variations in position and color.
- Error Checking: Checks if the font file exists and if the imagettftext() function is available in the GD library.
- Image Output: Sets the content type header based on the image MIME type and outputs the modified image using imagejpeg() or imagepng().
- Memory Cleanup: Frees up memory by destroying the image resource using imagedestroy().
Read More: How To Validate Indian Mobile Phone Numbers in PHP
How To Download Text Font?
Example,
$font = 'Rubik_Bubbles/RubikBubbles-Regular.ttf';
To download any font as per need simply open this google font link.
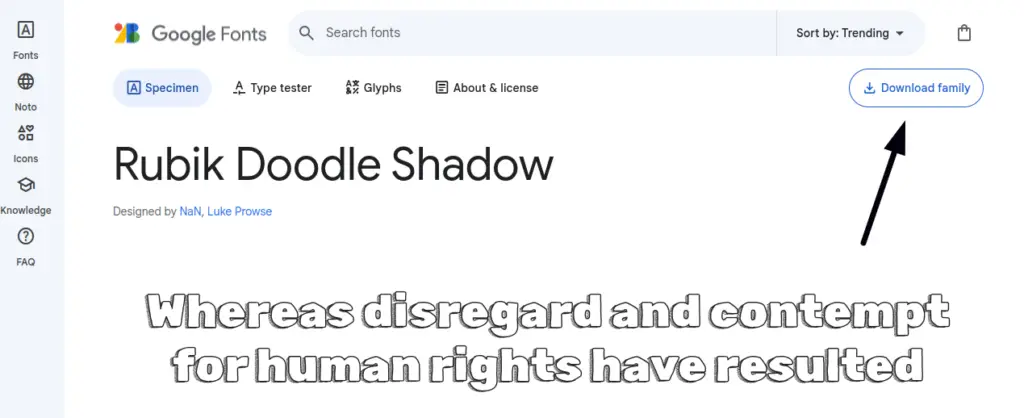
Once you download. Unzip that, link .ttf file with source code.
Application Testing
Open your project into browser,
URL: <Your_Project_URL>/text-on-image.php
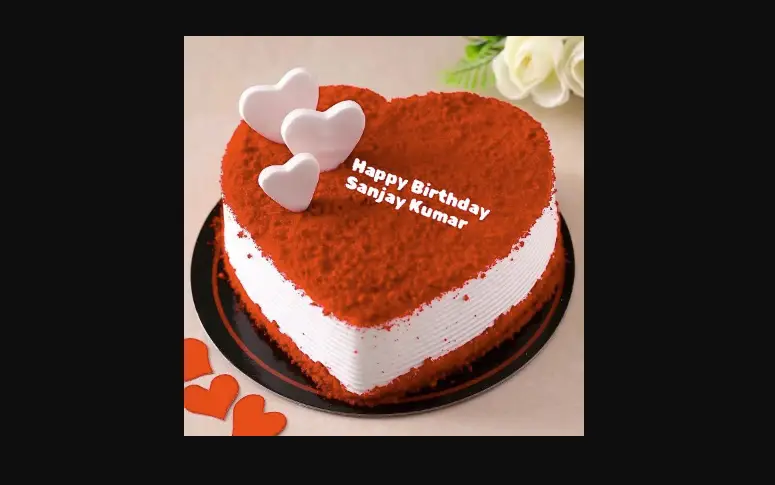
That’s it.
We hope this article helped you to learn about How To Write Text On Cake Image in PHP Example Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.