Using cURL to integrate the ChatGPT API into PHP offers up a world of possibilities for upgrading your applications with advanced natural language processing capabilities. We’ll walk you through a step-by-step approach for using the ChatGPT API in PHP, leveraging the cURL package for easy connection with the API endpoint.
Before we get into the details, it’s important to grasp the significance of including ChatGPT into your PHP projects.
ChatGPT, developed by OpenAI, allows your apps to interpret and generate human-like text, making it a powerful tool for developing intelligent chatbots, language translation services, and other applications.
Read More: How To Connect CodeIgniter 4 with Multiple Databases?
Let’s get started.
What is ChatGPT?
ChatGPT is a language model developed by OpenAI. It is part of the GPT (Generative Pre-trained Transformer) series, specifically GPT-3.5-turbo. ChatGPT is designed to generate human-like responses based on the input it receives.
Key features of ChatGPT include:
- Natural Language Understanding
- Contextual Understanding
- Versatility
- Large-scale Pre-training
Create an Account and Generate OpenAI API Key
To make use of ChatGPT, create an account on OpenAI platform to generate API keys.
You have to pass an email address and password. It will send you a verification email and later on asks for basic information like Name, Phone number, DOB etc.
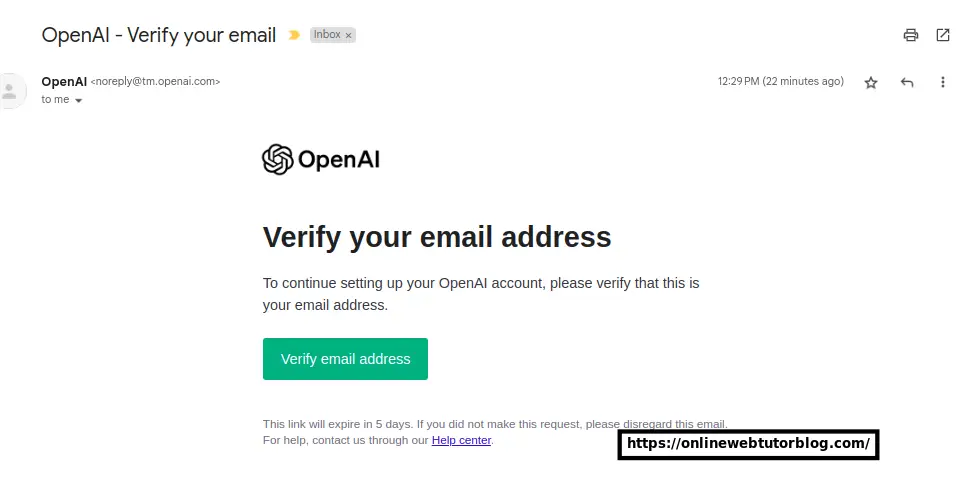
Once your account will be set successfully
Click on Create New Secret Key

Copy generated secret key.
Use of ChatGPT API in PHP Using cURL
Here, you can see we have the complete source code of ChatGPT API Integration in PHP.
<?php $openAISecretKey = "sk-UKlics6Ct2So5noVWWGzT3Bl..."; $search = "How artificial intelligence is transforming the world?"; $data = [ "model" => "gpt-3.5-turbo", "messages" => [ [ "role" => "user", "content" => $search, ], ], "temperature" => 0.5, "max_tokens" => 200, "top_p" => 1.0, "frequency_penalty" => 0.52, "presence_penalty" => 0.5, "stop" => ["11."], ]; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, "https://api.openai.com/v1/chat/completions"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_POST, 1); curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($data)); $headers = []; $headers[] = "Content-Type: application/json"; $headers[] = "Authorization: Bearer " . $openAISecretKey; curl_setopt($ch, CURLOPT_HTTPHEADER, $headers); $response = curl_exec($ch); // Check for cURL errors if (curl_errno($ch)) { echo "Curl error: " . curl_error($ch); } else { // Decode and print the API response $result = json_decode($response, true); echo "<pre>"; print_r($result); } // Close cURL session curl_close($ch);
Read More: Step-by-Step CodeIgniter 4 HMVC Programming Tutorial
Output
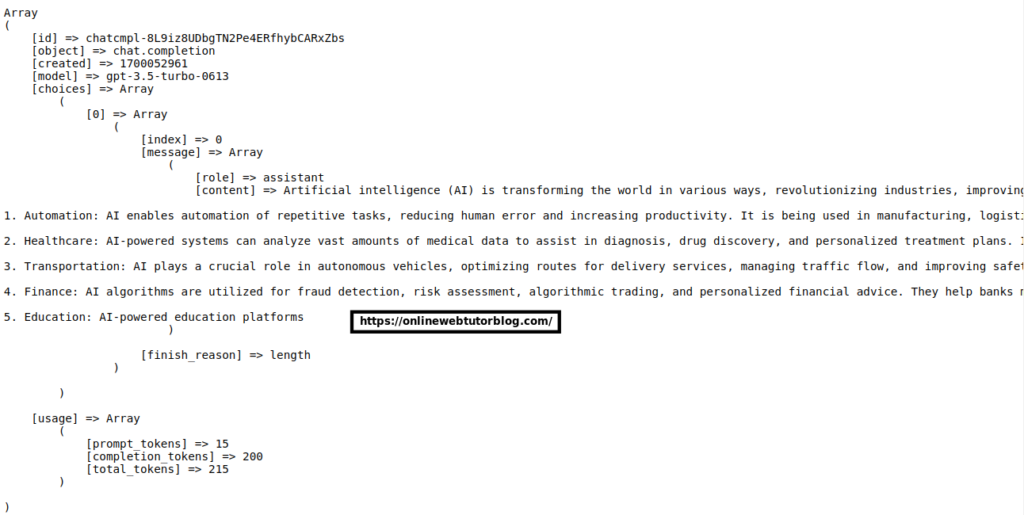
You will get all keys in response.
To get only proper message output of your query,
echo $result['choices'][0]['message']['content'];
That’s it.
We hope this article helped you to learn about How To Use ChatGPT API in PHP Using cURL Tutorial Example in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.