Inside this article we will one more important concept of laravel i.e REST api development in laravel 8 with Sanctum authentication. This will be step by step guide to create restful services from scratch.
We will create a secure set of API Authentication using Laravel 8 Sanctum. Sanctum is a laravel composer package.
What we will do in this article –
- User Register API
- Login API
- User Profile API
- Logout API
Above are the apis, we will create using sanctum authentication. This will be very interesting to learn.
- To Learn API development in Laravel 8 Using Passport, Click here.
- API development in Laravel 8 Using JWT Authentication, Click here.
Let’s get started.
Laravel Installation
We will create laravel project using composer. So, please make sure your system should have composer installed. If not, may be this article will help you to Install composer in system.
Here is the command to create a laravel project-
composer create-project --prefer-dist laravel/laravel blog
To start the development server of Laravel –
php artisan serve
URL: http://127.0.0.1:8000
Assuming laravel already installed inside your system.
Create Database & Connect
To create a database, either we can create via Manual tool of PhpMyadmin or by means of a mysql command.
CREATE DATABASE laravel_app;
To connect database with application, Open .env file from application root. Search for DB_ and update your details.
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=laravel_app DB_USERNAME=root DB_PASSWORD=root
Install And Configure Laravel Sanctum Auth
Laravel Sanctum provides a featherweight authentication system for SPAs (single page applications), mobile applications, and simple, token based APIs.
Open project into terminal and run this command.
$ composer require laravel/sanctum
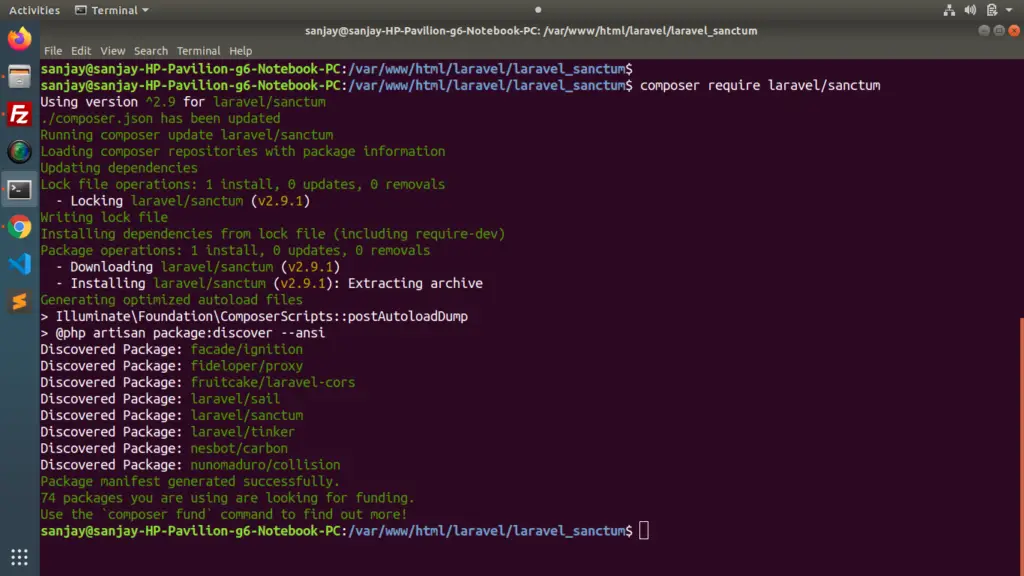
Publish Sanctum Package
Run this command to publish package.
$ php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
This will create a sanctum.php file in the /config directory, as well as the necessary migration files in the /database/migrations directory.
Migrate Migration Files
$ php artisan migrate
Setup User Model
Open User.php from /app/Models and write this following code into it.
<?php namespace App\Models; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; use Laravel\Sanctum\HasApiTokens; class User extends Authenticatable { use HasFactory, Notifiable, HasApiTokens; /** * The attributes that are mass assignable. * * @var array */ protected $fillable = [ 'name', 'email', 'password', ]; /** * The attributes that should be hidden for arrays. * * @var array */ protected $hidden = [ 'password', 'remember_token', ]; /** * The attributes that should be cast to native types. * * @var array */ protected $casts = [ 'email_verified_at' => 'datetime', ]; }
Create Authentication Controller
Open project into terminal and run this artisan command.
$ php artisan make:controller AuthController
It will create a file AuthController.php at /app/Http/Controllers folder.
Open AuthController.php file and write this following code into it.
<?php namespace App\Http\Controllers; use Illuminate\Http\Request; use Illuminate\Support\Facades\Hash; use App\Models\User; use Illuminate\Support\Facades\Auth; class AuthController extends Controller { public function register(Request $request) { $validatedData = $request->validate([ 'name' => 'required|string|max:255', 'email' => 'required|string|email|max:255|unique:users', 'password' => 'required|string|confirmed|min:6', ]); $user = User::create([ 'name' => $validatedData['name'], 'email' => $validatedData['email'], 'password' => Hash::make($validatedData['password']), ]); $token = $user->createToken('auth_token')->plainTextToken; return response()->json([ 'message' => "User registered successfully", 'access_token' => $token, 'token_type' => 'Bearer', ]); } public function login(Request $request) { if (!Auth::attempt($request->only('email', 'password'))) { return response()->json([ 'message' => 'Invalid login details' ], 401); } $user = User::where('email', $request['email'])->firstOrFail(); $token = $user->createToken('auth_token')->plainTextToken; return response()->json([ 'access_token' => $token, 'token_type' => 'Bearer', 'message' => "User logged in successfully", ]); } public function profile(Request $request) { return $request->user(); } public function logout(Request $request) { auth()->user()->tokens()->delete(); return response()->json(['message' => 'User successfully signed out']); } }
Update RouteServiceProvider File
Open RouteServiceProvider.php from /app/Providers folder. Uncomment this line from file.
protected $namespace = 'App\\Http\\Controllers';
Create Authentication Routes
Open api.php from /routes folder.
# Add to header use App\Http\Controllers\AuthController; Route::post('register', [AuthController::class, 'register']); Route::post('login', [AuthController::class, 'login']); Route::group(['middleware' => ['auth:sanctum']], function () { Route::post('profile', [AuthController::class, 'profile']); Route::post('logout', [AuthController::class, 'logout']); });
Application Testing
Run this command into project terminal to start development server,
php artisan serve
Register API – http://127.0.0.1:8000/api/register
Method – POST
Body
{ "name": "Sanjay Kumar", "email": "sanjay@gmail.com", "password": "123456", "password_confirmation": "123456" }
Header
Content-Type:application/json Accept:application/json
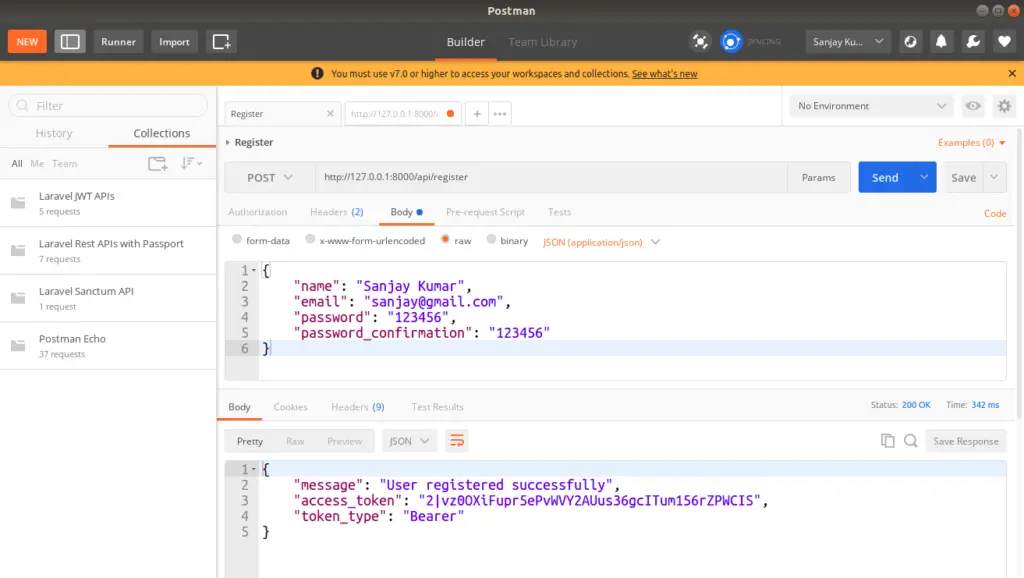
Login API – http://127.0.0.1:8000/api/login
Method – POST
Body
{ "email": "sanjay@gmail.com", "password": "123456" }
Header
Content-Type:application/json Accept:application/json
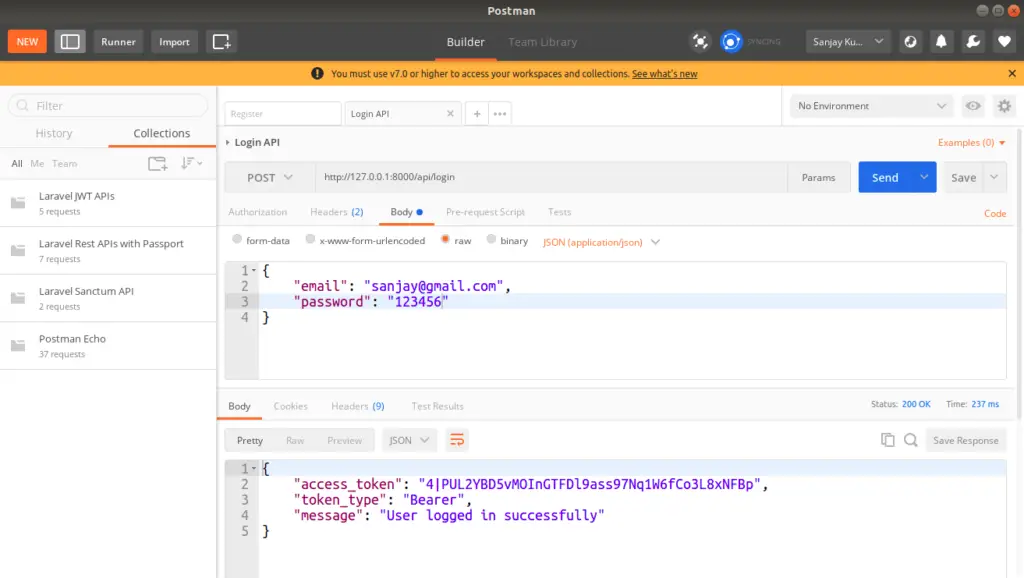
User Profile API – http://127.0.0.1:8000/api/profile
Method – POST
Header
Content-Type:application/json Accept:application/json Authorization: Bearer <Token>
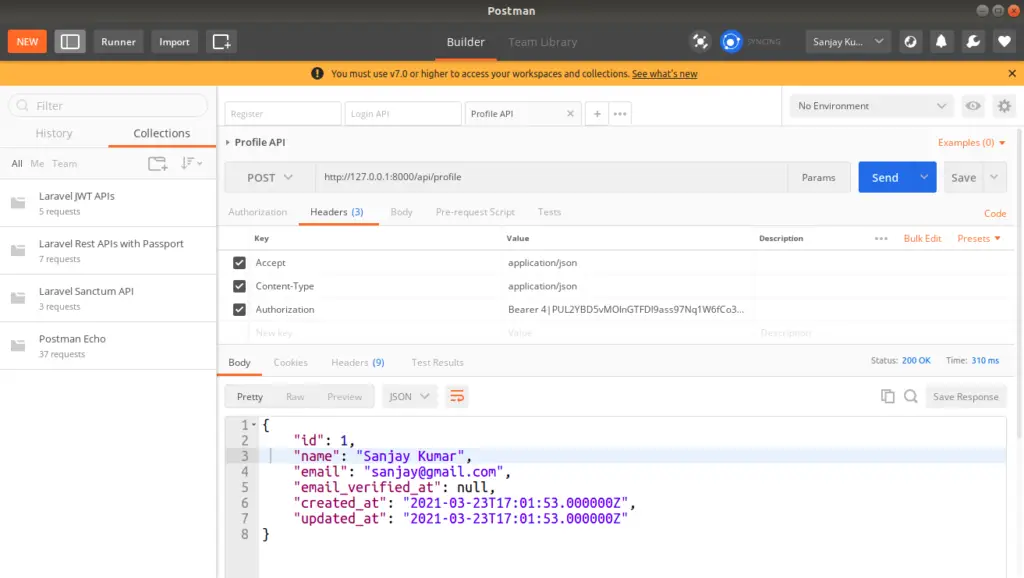
Logout API – http://127.0.0.1:8000/api/logout
Method – POST
Header
Content-Type:application/json Accept:application/json Authorization: Bearer <Token>
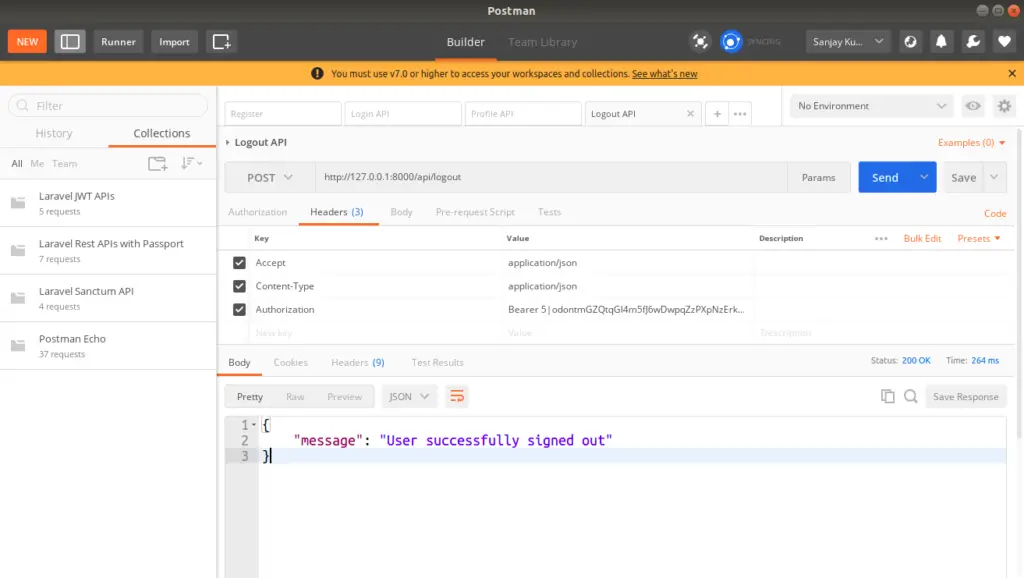
We hope this article helped you to learn about API Authentication using Laravel 8 Sanctum Tutorial in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.