Email communication often involves the need to share various files or documents alongside the message content. In CodeIgniter 4, enabling the functionality to send emails with attachments enhances communication by allowing users to share diverse files within their email correspondences, facilitating seamless information exchange.
In this tutorial, we’ll see the comprehensive process of sending emails with attachments using CodeIgniter 4. This functionality empowers developers to create and dispatch emails with different types of attachments
We will use attach() method to add files with email as attachments.
Read More: CodeIgniter 4 How To Find Data Using Multiple Ids Tutorial
Let’s get started.
What is Email Class in CodeIgniter 4?
Email class is a in-built library. There are several features available by the help of which we configure emails. CodeIgniter 4 email class have all these features
- Multiple Protocols: Mail, Sendmail, and SMTP
- TLS and SSL Encryption for SMTP
- Multiple recipients
- CC and BCCs
- HTML or Plaintext email
- Attachments
- Word wrapping
- Priorities
- BCC Batch Mode, enabling large email lists to be broken into small BCC batches.
- Email Debugging tools
CodeIgniter 4 Installation
To create a CodeIgniter 4 setup run this given command into your shell or terminal. Please make sure composer should be installed.
composer create-project codeigniter4/appstarter codeigniter-4
Assuming you have successfully installed application into your local system.
Environment (.env) Setup
When we install CodeIgniter 4, we will have env file at root. To use the environment variables means using variables at global scope we need to do env to .env
Either we can do via renaming file as simple as that. Also we can do by terminal command.
Open project in terminal
cp env .env
Above command will create a copy of env file to .env file. Now we are ready to use environment variables.
Enable Development Mode
CodeIgniter starts up in production mode by default. You need to make it in development mode to see any error if you are working with application.
Open .env file from root.
# CI_ENVIRONMENT = production
// Do it to
CI_ENVIRONMENT = development
Now application is in development mode.
Email Library Class
To use Email library class in codeigniter 4 application, we need to do some email configuration inside Email.php file of /app/Config folder
It’s a class file, where we set some values to class member variables.
After doing all needed configuration settings, we load in application like this before use.
$email = \Config\Services::email();
//... rest code for email
Read More: CodeIgniter 4 Export MySQL Table Data into CSV File Tutorial
Email Settings Configuration
For email configuration settings, we can use any sending email protocol like mail, sendmail or smtp.
Here, we will use SMTP and use Host as Gmail.
Open up the Email.php file from /app/Config folder.
<?php namespace Config; use CodeIgniter\Config\BaseConfig; class Email extends BaseConfig { /** * @var string */ public $fromEmail = "xxxxx@gmail.com"; /** * @var string */ public $fromName = "Online Web Tutor Support"; /** * @var string */ public $recipients; /** * The "user agent" * * @var string */ public $userAgent = 'CodeIgniter'; /** * The mail sending protocol: mail, sendmail, smtp * * @var string */ public $protocol = 'smtp'; /** * The server path to Sendmail. * * @var string */ public $mailPath = '/usr/sbin/sendmail'; /** * SMTP Server Address * * @var string */ public $SMTPHost = "smtp.gmail.com"; /** * SMTP Username * * @var string */ public $SMTPUser = "xxxxx@gmail.com"; /** * SMTP Password * * @var string */ public $SMTPPass = "xxxxxxx"; /** * SMTP Port * * @var integer */ public $SMTPPort = 587; /** * SMTP Timeout (in seconds) * * @var integer */ public $SMTPTimeout = 60; /** * Enable persistent SMTP connections * * @var boolean */ public $SMTPKeepAlive = false; /** * SMTP Encryption. Either tls or ssl * * @var string */ public $SMTPCrypto = 'tls'; /** * Enable word-wrap * * @var boolean */ public $wordWrap = true; /** * Character count to wrap at * * @var integer */ public $wrapChars = 76; /** * Type of mail, either 'text' or 'html' * * @var string */ public $mailType = 'text'; /** * Character set (utf-8, iso-8859-1, etc.) * * @var string */ public $charset = 'UTF-8'; /** * Whether to validate the email address * * @var boolean */ public $validate = false; /** * Email Priority. 1 = highest. 5 = lowest. 3 = normal * * @var integer */ public $priority = 3; /** * Newline character. (Use "\r\n" to comply with RFC 822) * * @var string */ public $CRLF = "\r\n"; /** * Newline character. (Use "\r\n" to comply with RFC 822) * * @var string */ public $newline = "\r\n"; /** * Enable BCC Batch Mode. * * @var boolean */ public $BCCBatchMode = false; /** * Number of emails in each BCC batch * * @var integer */ public $BCCBatchSize = 200; /** * Enable notify message from server * * @var boolean */ public $DSN = false; }
Here, inside this file we have configured all basic settings for sending emails. But one more thing before setting your email address and password to a SMTP details. You need to make it as for LESS SECURE APPS AT YOUR GOOGLE ACCOUNT.
When you go at your given email account settings. You will find a Security Tab. When you click into it –
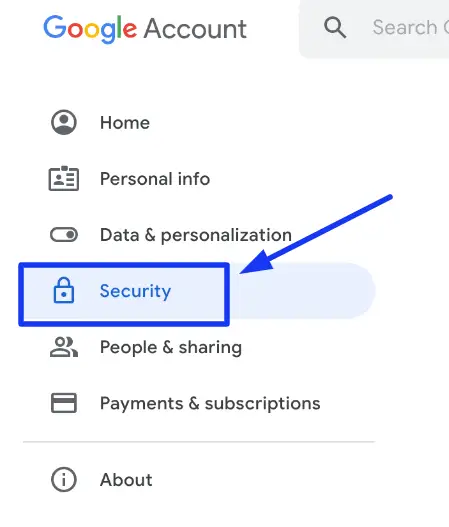
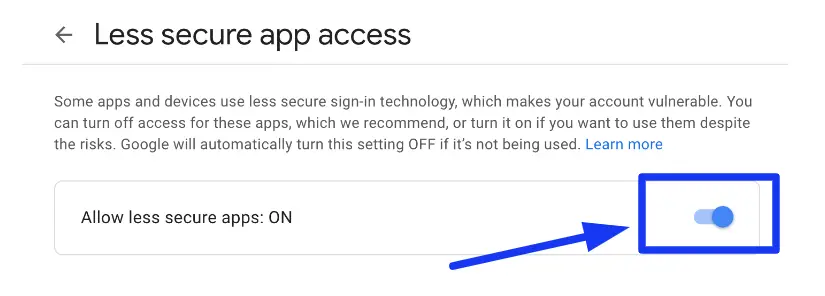
Next, we need to create a method in controller and call Email service.
Add Route
Open Routes.php from /app/Config folder. Add given route into it.
//... $routes->get("send-mail", "UserController::sendMail"); //...
Read More: CodeIgniter 4 Autocomplete Places Search Using Google Maps JavaScript API
Use & Send Email Using Library
We need to create a controller.
Controller Setup
Open project into terminal and run this spark command to create controller.
php spark make:controller User --suffix
It will create a file UserController.php inside /app/Controllers folder. Assuming we have a file 1.png an image inside /public/data folder.
Open file and write this code.
<?php namespace App\Controllers; use App\Controllers\BaseController; class UserController extends BaseController { public function sendMail() { $email = \Config\Services::email(); // loading for use // Email to $email->setTo("xxxx@gmail.com"); $email->setSubject("Sample Mail - Online Web Tutor"); $email->setMessage("This is test mail with sample attachment."); // Attachment $email->attach(FCPATH . "data/1.png"); // Send email if ($email->send()) { echo 'Email successfully sent, please check.'; } else { $data = $email->printDebugger(['headers']); print_r($data); } } }
Concept to attach file with email
$email->attach(FCPATH . "data/1.png");
FCPATH is a CodeIgniter 4 constant which returns the path upto /public folder.
If you have your attachment files inside /writable folder then you should use WRITEPATH in place of it.
Add Multiple Attachments to Email
Assuming we have files as two images, a PDF file inside /public/data folder.
// Attachments
$attachments = [
FCPATH . "data/1.png",
FCPATH . "data/my-image.jpg",
FCPATH . "data/onlinewebtutorblog.pdf"
];
foreach ($attachments as $file) {
$email->attach($file);
}
Complete Code for Sending Multiple Attachments
<?php namespace App\Controllers; use App\Controllers\BaseController; class UserController extends BaseController { public function sendMail() { $email = \Config\Services::email(); // loading for use // Email to $email->setTo("xxxx@gmail.com"); $email->setSubject("Sample Mail - Online Web Tutor"); $email->setMessage("This is test mail with sample attachments."); // Attachments $attachments = [ FCPATH . "data/1.png", FCPATH . "data/my-image.jpg", FCPATH . "data/onlinewebtutorblog.pdf" ]; foreach ($attachments as $file) { $email->attach($file); } // Send email if ($email->send()) { echo 'Email successfully sent, please check.'; } else { $data = $email->printDebugger(['headers']); print_r($data); } } }
Application Testing
Open project terminal and start development server via command:
php spark serve
URL: http://localhost:8080/send-mail
Output
Email successfully sent, please check.

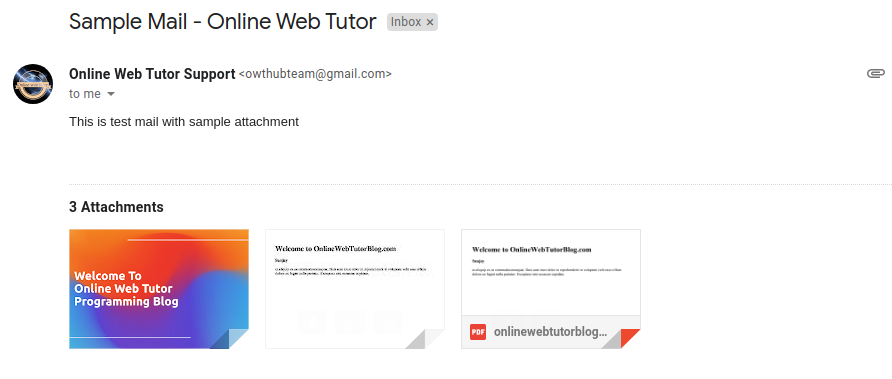
That’s it.
We hope this article helped you to learn about CodeIgniter 4 How To Send Email with Attachments Tutorial in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.