Inside this article we will see how to encrypt data in javascript before sending to server. We will use Crypto js plugin. Submitting data to server in encrypted format is good practise to secure data at client side.
Here, we will provide a zipped folder in which you will get Encryption.js and Encryption.php file. This package will be used to encrypt and decrypt form data at the client-side as well as server-side. The javascript file will be used when you want to send the form data to the server.
Learn More –
- Copy Entire Contents of a Directory to Another Directory in PHP
- How To Generate Fake Data in PHP Using Faker Library
- How to Generate Fake Image URLs in PHP Using Faker
- How to Generate Random Name of Person in PHP
Let’s get started.
Create PHP Application
Create a folder as php-form inside your localhost directory.
Encryption Files Setup
Download this folder from here. Unzip and put inside your php-form application.
It contains Encryption.js and Encryption.php files.
Encryption.js – It contains encryption methods which encrypt form data before sending to server.
Encryption.php – It contains decryption methods which helps to decrypt data.
Next, you need to create form.php (It contains a form) and process.php (It process form data at server when we will submit).
Now, inside php-form application folder you have 4 files –
- Encryption.js
- Encryption.php
- form.php
- process.php
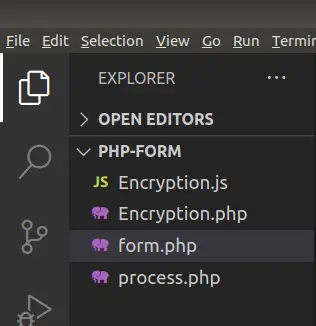
Application Code Settings
Open form.php and write this complete code into it.
<?php // You can put your nonce_value. // nonce_value means any random set of characters which helps to send and receive the valid HTTP request $nonceValue = 'your_nonce_value'; ?> <!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>Encrypt form data before sending to Server in Javascript</title> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> </head> <body> <div class="container py-4"> <div class="row"> <div class="col-xl-8 col-lg-8 m-auto"> <form method="POST" id="regForm"> <div class="card shadow"> <div class="card-header"> <h5 class="card-title font-weight-bold" style="text-align:center;">Encrypt form data before sending to Server in Javascript</h5> </div> <div class="card-body"> <div class="form-group"> <input type="text" id="name" name="name" class="form-control" placeholder="Your name" required/> </div> <div class="form-group"> <input type="email" id="email" name="email" class="form-control" placeholder="Your Email" required/> </div> <div class="form-group"> <input type="password" id="password" name="password" class="form-control" placeholder="Your Password" required/> </div> </div> <div class="card-footer"> <button type="submit" class="btn btn-success">Save</button> </div> </div> </form> </div> </div> </div> <!-- jQuery --> <script src="https://code.jquery.com/jquery-3.3.1.min.js"></script> <!-- Crypto js --> <script src="https://cdnjs.cloudflare.com/ajax/libs/crypto-js/4.0.0/crypto-js.min.js"></script> <!-- Encryption js --> <script src="Encryption.js"></script> <script> $(document).ready(function() { // Submit form $("#regForm").submit(function (event) { event.preventDefault(); var name = $("#name").val(); var email = $("#email").val(); var password = $("#password").val(); var nonceValue = "<?php echo $nonceValue; ?>"; // Encrypt form data let encryption = new Encryption(); var nameEncrypted = encryption.encrypt(name, nonceValue); var emailEncrypted = encryption.encrypt(email, nonceValue); var passwordEncrypted = encryption.encrypt(password, nonceValue); // Submit form using Ajax $.ajax({ url: 'process.php', // file to handle this request method: 'POST', data: { name: nameEncrypted, email: emailEncrypted, password: passwordEncrypted }, success:function(res) { // console response from server console.log(res); } }); }); }); </script> </body> </html>
Open process.php and write this complete code into it.
<?php require 'Encryption.php'; // Same nonce value what you have used at form.php $nonceValue = 'your_nonce_value'; // get encrypted form data $name = isset($_REQUEST['name']) ? $_REQUEST['name'] : ""; $email = isset($_REQUEST['email']) ? $_REQUEST['email'] : ""; $password = isset($_REQUEST['password']) ? $_REQUEST['password'] : ""; // create object of encryption method $Encryption = new Encryption(); // decrypt encrypted data $nameDecrypted = $Encryption->decrypt($name, $nonceValue); $emailDecrypted = $Encryption->decrypt($email, $nonceValue); $passwordDecrypted = $Encryption->decrypt($password, $nonceValue); // display result after decrypting echo json_encode(array( "name" => $nameDecrypted, "email" => $emailDecrypted, "password" => $passwordDecrypted )); ?>
Application Testing
Open browser and type URL – http://localhost/php-form/form.php
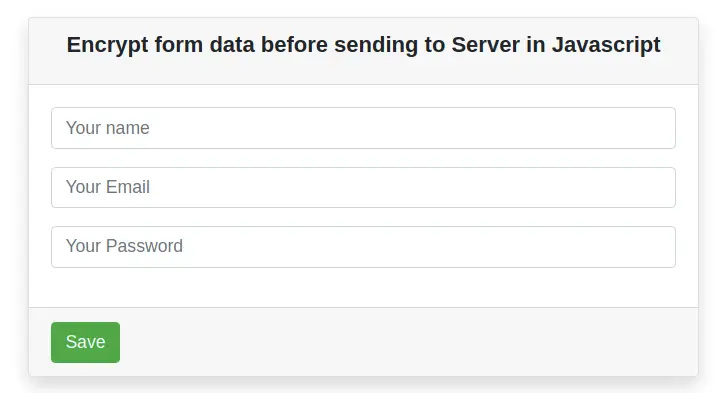
Provide data into form and submit to process.php
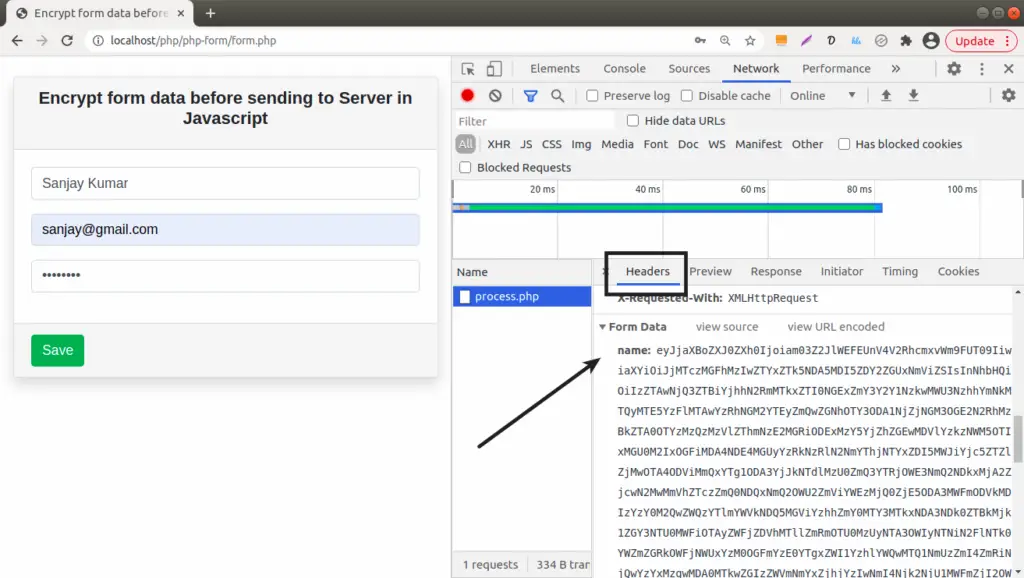
We can see into headers data is travelling into encrypted format.
Now, when process.php will process and decrypt data then look that the response –
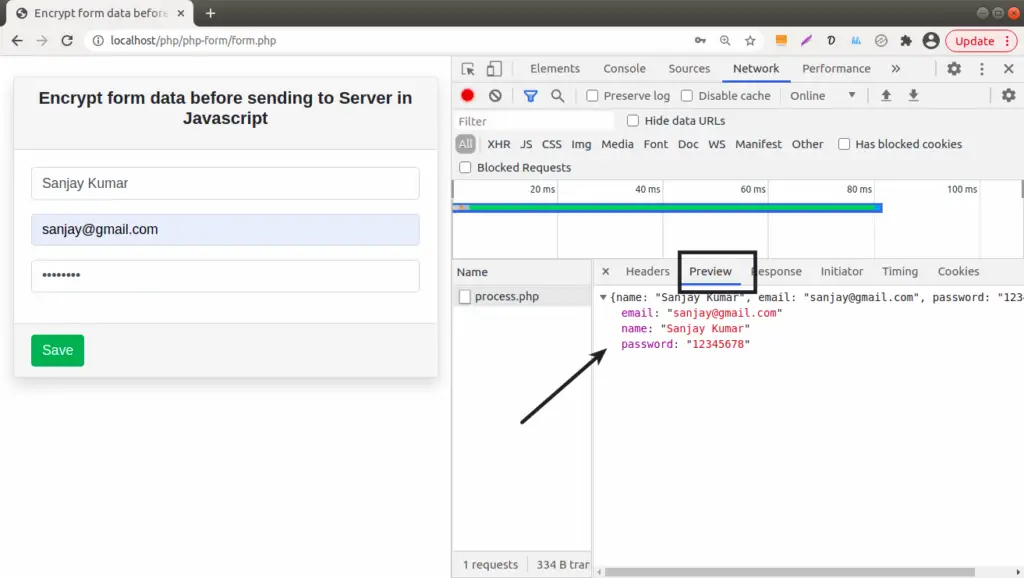
We hope this article helped you to learn Encrypt Form Data in JavaScript Before Sending to Server in a very detailed way.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.